stage.hh
Go to the documentation of this file.00001 #ifndef STG_H 00002 #define STG_H 00003 /* 00004 * Stage : a multi-robot simulator. Part of the Player Project 00005 * 00006 * Copyright (C) 2001-2008 Richard Vaughan, Brian Gerkey, Andrew 00007 * Howard, Toby Collett, Reed Hedges, Alex Couture-Beil, Jeremy 00008 * Asher, Pooya Karimian 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License 00021 * along with this program; if not, write to the Free Software 00022 * Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA 00023 * 00024 */ 00025 00037 #include <unistd.h> 00038 #include <stdint.h> // for portable int types eg. uint32_t 00039 #include <assert.h> 00040 #include <math.h> 00041 #include <stdlib.h> 00042 #include <stdio.h> 00043 #include <string.h> 00044 #include <sys/types.h> 00045 #include <sys/time.h> 00046 #include <iostream> 00047 #include <vector> 00048 00049 // we use GLib's data structures extensively. Perhaps we'll move to 00050 // C++ STL types to lose this dependency one day. 00051 #include <glib.h> 00052 00053 // FLTK Gui includes 00054 #include <FL/Fl.H> 00055 #include <FL/Fl_Box.H> 00056 #include <FL/Fl_Gl_Window.H> 00057 #include <FL/Fl_Menu_Bar.H> 00058 #include <FL/Fl_Window.H> 00059 #include <FL/fl_draw.H> 00060 #include <FL/gl.h> // FLTK takes care of platform-specific GL stuff 00061 //#include <FL/glut.H> 00062 00063 #ifdef __APPLE__ 00064 #include <OpenGL/glu.h> 00065 #else 00066 #include <GL/glu.h> 00067 #endif 00068 00069 #include "option.hh" 00070 00072 namespace Stg 00073 { 00074 // foreward declare 00075 class StgCanvas; 00076 class Worldfile; 00077 class StgWorld; 00078 class StgWorldGui; 00079 class StgModel; 00080 class FileManager; 00081 class OptionsDlg; 00082 class StgCamera; 00083 00085 void Init( int* argc, char** argv[] ); 00086 00088 bool InitDone(); 00089 00092 typedef enum { 00093 MODEL_TYPE_PLAIN = 0, 00094 MODEL_TYPE_LASER, 00095 MODEL_TYPE_FIDUCIAL, 00096 MODEL_TYPE_RANGER, 00097 MODEL_TYPE_POSITION, 00098 MODEL_TYPE_BLOBFINDER, 00099 MODEL_TYPE_BLINKENLIGHT, 00100 MODEL_TYPE_CAMERA, 00101 MODEL_TYPE_COUNT // must be the last entry, to count the number of 00102 // types 00103 } stg_model_type_t; 00104 00105 00107 const char COPYRIGHT[] = 00108 "Copyright Richard Vaughan and contributors 2000-2008"; 00109 00111 const char AUTHORS[] = 00112 "Richard Vaughan, Brian Gerkey, Andrew Howard, Reed Hedges, Pooya Karimian, Toby Collett, Jeremy Asher, Alex Couture-Beil and contributors."; 00113 00115 const char WEBSITE[] = "http://playerstage.org"; 00116 00118 const char DESCRIPTION[] = 00119 "Robot simulation library\nPart of the Player Project"; 00120 00122 const char LICENSE[] = 00123 "Stage robot simulation library\n" \ 00124 "Copyright (C) 2000-2008 Richard Vaughan and contributors\n" \ 00125 "Part of the Player Project [http://playerstage.org]\n" \ 00126 "\n" \ 00127 "This program is free software; you can redistribute it and/or\n" \ 00128 "modify it under the terms of the GNU General Public License\n" \ 00129 "as published by the Free Software Foundation; either version 2\n" \ 00130 "of the License, or (at your option) any later version.\n" \ 00131 "\n" \ 00132 "This program is distributed in the hope that it will be useful,\n" \ 00133 "but WITHOUT ANY WARRANTY; without even the implied warranty of\n" \ 00134 "MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the\n" \ 00135 "GNU General Public License for more details.\n" \ 00136 "\n" \ 00137 "You should have received a copy of the GNU General Public License\n" \ 00138 "along with this program; if not, write to the Free Software\n" \ 00139 "Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.\n" \ 00140 "\n" \ 00141 "The text of the license may also be available online at\n" \ 00142 "http://www.gnu.org/licenses/old-licenses/gpl-2.0.html\n"; 00143 00146 const uint32_t TOKEN_MAX = 64; 00147 00150 const double thousand = 1e3; 00151 00154 const double million = 1e6; 00155 00158 const double billion = 1e9; 00159 00162 inline double rtod( double r ){ return( r*180.0/M_PI ); } 00163 00166 inline double dtor( double d ){ return( d*M_PI/180.0 ); } 00167 00170 inline double normalize( double a ) 00171 { 00172 //optimized version of return( atan2(sin(a), cos(a))); 00173 while( a < -M_PI ) a += 2.0 * M_PI; 00174 while( a > M_PI ) a -= 2.0 * M_PI; 00175 return a; 00176 } 00177 00178 00181 inline int sgn( int a){ return( a<0 ? -1 : 1); } 00182 00185 inline double sgn( double a){ return( a<0 ? -1.0 : 1.0); } 00186 00187 // forward declare 00188 class StgModel; 00189 00192 typedef enum { 00193 STG_IMAGE_FORMAT_PNG, 00194 STG_IMAGE_FORMAT_JPG 00195 } stg_image_format_t; 00196 00199 enum { FiducialNone = 0 }; 00200 00203 typedef uint32_t stg_id_t; 00204 00207 typedef double stg_meters_t; 00208 00211 typedef double stg_radians_t; 00212 00214 typedef struct timeval stg_time_t; 00215 00217 typedef unsigned long stg_msec_t; 00218 00220 typedef uint64_t stg_usec_t; 00221 00223 typedef double stg_kg_t; // Kilograms (mass) 00224 00226 typedef double stg_joules_t; 00227 00229 typedef double stg_watts_t; 00230 00232 typedef uint32_t stg_bool_t; 00233 00234 //typedef double stg_friction_t; 00235 00237 typedef uint32_t stg_color_t; 00238 00240 stg_color_t stg_color_pack( double r, double g, double b, double a ); 00241 00243 void stg_color_unpack( stg_color_t col, 00244 double* r, double* g, double* b, double* a ); 00245 00248 //typedef int stg_obstacle_return_t; 00249 00252 //typedef int stg_blob_return_t; 00253 00255 //typedef int stg_fiducial_return_t; 00256 00257 //typedef int stg_ranger_return_t; 00258 00259 //typedef enum { STG_GRIP_NO = 0, STG_GRIP_YES } stg_gripper_return_t; 00260 00262 typedef struct 00263 { 00264 stg_meters_t x, y, z; 00265 } stg_size_t; 00266 00268 typedef struct 00269 { 00270 stg_meters_t x, y, z; //< location in 3 axes 00271 stg_radians_t a; //< rotation about the z axis. 00272 } stg_pose_t; 00273 00275 typedef stg_pose_t stg_velocity_t; 00276 00279 typedef struct 00280 { 00281 stg_pose_t pose; //< position 00282 stg_size_t size; //< extent 00283 } stg_geom_t; 00284 00286 typedef struct 00287 { 00288 double min; //< smallest value in range 00289 double max; //< largest value in range 00290 } stg_bounds_t; 00291 00293 typedef struct 00294 { 00295 stg_bounds_t x; //< volume extent along x axis 00296 stg_bounds_t y; //< volume extent along y axis 00297 stg_bounds_t z; //< volume extent along z axis 00298 } stg_bounds3d_t; 00299 00302 typedef struct 00303 { 00304 stg_meters_t min; //< smallest value in range 00305 stg_meters_t max; //< largest value in range 00306 } stg_range_bounds_t; 00307 00309 typedef struct 00310 { 00311 stg_bounds_t x, y, z; 00312 } stg_bbox3d_t; 00313 00315 typedef struct 00316 { 00317 stg_bounds_t range; //< min and max range of sensor 00318 stg_radians_t angle; //< width of viewing angle of sensor 00319 } stg_fov_t; 00320 00321 00323 typedef struct 00324 { 00325 stg_meters_t x, y; 00326 } stg_point_t; 00327 00329 typedef struct 00330 { 00331 float x, y, z; 00332 } stg_vertex_t; 00333 00335 typedef struct 00336 { 00337 float x, y, z, r, g, b, a; 00338 } stg_colorvertex_t; 00339 00341 typedef struct 00342 { 00343 stg_meters_t x, y, z; 00344 } stg_point3_t; 00345 00348 typedef struct 00349 { 00350 int32_t x,y; 00351 } stg_point_int_t; 00352 00356 stg_point_t* stg_points_create( size_t count ); 00357 00359 void stg_points_destroy( stg_point_t* pts ); 00360 00363 stg_point_t* stg_unit_square_points_create(); 00364 00365 00368 typedef int(*stg_line3d_func_t)(int32_t x, int32_t y, int32_t z, 00369 void* arg ); 00370 00371 00376 int stg_line_3d( int32_t x, int32_t y, int32_t z, 00377 int32_t dx, int32_t dy, int32_t dz, 00378 stg_line3d_func_t visit_voxel, 00379 void* arg ); 00380 00383 int stg_polygon_3d( stg_point_int_t* pts, 00384 unsigned int pt_count, 00385 stg_line3d_func_t visit_voxel, 00386 void* arg ); 00387 00390 stg_pose_t new_pose( stg_meters_t x, stg_meters_t y, stg_meters_t z, stg_radians_t a ); 00391 00394 stg_pose_t random_pose( stg_meters_t xmin, stg_meters_t xmax, 00395 stg_meters_t ymin, stg_meters_t ymax ); 00396 00397 const uint32_t STG_MOVE_TRANS = (1 << 0); 00398 const uint32_t STG_MOVE_ROT = (1 << 1); 00399 const uint32_t STG_MOVE_SCALE = (1 << 2); 00400 00401 typedef uint32_t stg_movemask_t; 00402 00403 const char STG_MP_PREFIX[] = "_mp_"; 00404 const char STG_MP_POSE[] = "_mp_pose"; 00405 const char STG_MP_VELOCITY[] = "_mp_velocity"; 00406 const char STG_MP_GEOM[] = "_mp_geom"; 00407 const char STG_MP_COLOR[] = "_mp_color"; 00408 const char STG_MP_WATTS[] = "_mp_watts"; 00409 const char STG_MP_FIDUCIAL_RETURN[] = "_mp_fiducial_return"; 00410 const char STG_MP_LASER_RETURN[] = "_mp_laser_return"; 00411 const char STG_MP_OBSTACLE_RETURN[] = "_mp_obstacle_return"; 00412 const char STG_MP_RANGER_RETURN[] = "_mp_ranger_return"; 00413 const char STG_MP_GRIPPER_RETURN[] = "_mp_gripper_return"; 00414 const char STG_MP_MASS[] = "_mp_mass"; 00415 00416 00418 typedef enum 00419 { 00420 LaserTransparent, 00421 LaserVisible, 00422 LaserBright 00423 } stg_laser_return_t; 00424 00425 00426 namespace Draw 00427 { 00428 typedef struct { 00429 double x, y, z; 00430 } vertex_t; 00431 00432 typedef struct { 00433 vertex_t min, max; 00434 } bounds3_t; 00435 00436 typedef enum { 00437 STG_D_DRAW_POINTS, 00438 STG_D_DRAW_LINES, 00439 STG_D_DRAW_LINE_STRIP, 00440 STG_D_DRAW_LINE_LOOP, 00441 STG_D_DRAW_TRIANGLES, 00442 STG_D_DRAW_TRIANGLE_STRIP, 00443 STG_D_DRAW_TRIANGLE_FAN, 00444 STG_D_DRAW_QUADS, 00445 STG_D_DRAW_QUAD_STRIP, 00446 STG_D_DRAW_POLYGON, 00447 STG_D_PUSH, 00448 STG_D_POP, 00449 STG_D_ROTATE, 00450 STG_D_TRANSLATE, 00451 } type_t; 00452 00454 typedef struct 00455 { 00456 type_t type; 00457 } hdr_t; 00458 00460 typedef hdr_t push_t; 00462 typedef hdr_t pop_t; 00463 00465 typedef struct 00466 { 00468 type_t type; 00470 size_t vert_count; 00472 vertex_t verts[]; 00473 } draw_t; 00474 00476 typedef struct 00477 { 00479 type_t type; 00481 vertex_t vector; 00482 } translate_t; 00483 00485 typedef struct 00486 { 00488 type_t type; 00490 vertex_t vector; 00492 stg_radians_t angle; 00493 } rotate_t; 00494 00496 draw_t* create( type_t type, 00497 vertex_t* verts, 00498 size_t vert_count ); 00499 00501 void destroy( draw_t* d ); 00502 } // end namespace draw 00503 00504 00505 // MACROS ------------------------------------------------------ 00506 // Some useful macros 00507 00508 00513 stg_color_t stg_lookup_color(const char *name); 00514 00517 void stg_pose_sum( stg_pose_t* result, stg_pose_t* p1, stg_pose_t* p2 ); 00518 00520 stg_pose_t pose_sum( stg_pose_t p1, stg_pose_t p2 ); 00521 00522 // PRETTY PRINTING ------------------------------------------------- 00523 00525 void stg_print_err( const char* err ); 00527 void stg_print_geom( stg_geom_t* geom ); 00529 void stg_print_pose( stg_pose_t* pose ); 00531 void stg_print_velocity( stg_velocity_t* vel ); 00532 00533 // const uint32_t STG_SHOW_BLOCKS = (1<<0); 00534 // const uint32_t STG_SHOW_DATA = (1<<1); 00535 // const uint32_t STG_SHOW_GEOM = (1<<2); 00536 // const uint32_t STG_SHOW_GRID = (1<<3); 00537 // const uint32_t STG_SHOW_OCCUPANCY = (1<<4); 00538 // const uint32_t STG_SHOW_TRAILS = (1<<5); 00539 // const uint32_t STG_SHOW_FOLLOW = (1<<6); 00540 // const uint32_t STG_SHOW_CLOCK = (1<<7); 00541 // const uint32_t STG_SHOW_QUADTREE = (1<<8); 00542 // const uint32_t STG_SHOW_ARROWS = (1<<9); 00543 // const uint32_t STG_SHOW_FOOTPRINT = (1<<10); 00544 // const uint32_t STG_SHOW_BLOCKS_2D = (1<<10); 00545 // const uint32_t STG_SHOW_TRAILRISE = (1<<11); 00546 // const uint32_t STG_SHOW_STATUS = (1<<12); 00547 00548 // forward declare 00549 class StgWorld; 00550 class StgModel; 00551 00552 00554 typedef StgModel* (*stg_creator_t)( StgWorld*, StgModel* ); 00555 00556 typedef struct 00557 { 00558 const char* token; 00559 stg_creator_t creator; 00560 } stg_typetable_entry_t; 00561 00563 extern stg_typetable_entry_t typetable[MODEL_TYPE_COUNT]; 00564 00565 void RegisterModel( stg_model_type_t type, 00566 const char* name, 00567 stg_creator_t creator ); 00568 00569 void RegisterModels(); 00570 00571 void gl_draw_grid( stg_bounds3d_t vol ); 00572 void gl_pose_shift( stg_pose_t* pose ); 00573 void gl_coord_shift( double x, double y, double z, double a ); 00574 00575 class StgFlag 00576 { 00577 public: 00578 stg_color_t color; 00579 double size; 00580 00581 StgFlag( stg_color_t color, double size ); 00582 StgFlag* Nibble( double portion ); 00583 }; 00584 00586 void gl_draw_string( float x, float y, float z, const char *string); 00587 void gl_speech_bubble( float x, float y, float z, const char* str ); 00588 void gl_draw_octagon( float w, float h, float m ); 00589 00590 void stg_block_list_scale( GList* blocks, 00591 stg_size_t* size ); 00592 void stg_block_list_destroy( GList* list ); 00593 00594 00595 typedef int(*stg_model_callback_t)(StgModel* mod, void* user ); 00596 00597 // return val, or minval if val < minval, or maxval if val > maxval 00598 double constrain( double val, double minval, double maxval ); 00599 00600 00603 typedef struct 00604 { 00605 stg_model_callback_t callback; 00606 void* arg; 00607 } stg_cb_t; 00608 00609 stg_cb_t* cb_create( stg_model_callback_t callback, void* arg ); 00610 void cb_destroy( stg_cb_t* cb ); 00611 00613 typedef struct 00614 { 00615 stg_pose_t pose; 00616 stg_size_t size; 00617 } stg_rotrect_t; // rotated rectangle 00618 00622 void stg_rotrects_normalize( stg_rotrect_t* rects, int num ); 00623 00629 int stg_rotrects_from_image_file( const char* filename, 00630 stg_rotrect_t** rects, 00631 unsigned int* rect_count, 00632 unsigned int* widthp, 00633 unsigned int* heightp ); 00634 00635 // /** matching function should return 0 iff the candidate block is 00636 // acceptable */ 00637 class StgBlock; 00638 00639 typedef bool (*stg_block_match_func_t)(StgBlock* candidate, StgModel* finder, const void* arg ); 00640 00641 // TODO - some of this needs to be implemented, the rest junked. 00642 00643 /* // -------------------------------------------------------------- */ 00644 00645 /* // standard energy consumption of some devices in W. */ 00646 /* // */ 00647 /* // The MOTIONKG value is a hack to approximate cost of motion, as */ 00648 /* // Stage does not yet have an acceleration model. */ 00649 /* // */ 00650 /* #define STG_ENERGY_COST_LASER 20.0 // 20 Watts! (LMS200 - from SICK web site) */ 00651 /* #define STG_ENERGY_COST_FIDUCIAL 10.0 // 10 Watts */ 00652 /* #define STG_ENERGY_COST_RANGER 0.5 // 500mW (estimate) */ 00653 /* #define STG_ENERGY_COST_MOTIONKG 10.0 // 10 Watts per KG when moving */ 00654 /* #define STG_ENERGY_COST_BLOB 4.0 // 4W (estimate) */ 00655 00656 /* typedef struct */ 00657 /* { */ 00658 /* stg_joules_t joules; // current energy stored in Joules/1000 */ 00659 /* stg_watts_t watts; // current power expenditure in mW (mJoules/sec) */ 00660 /* int charging; // 1 if we are receiving energy, -1 if we are */ 00661 /* // supplying energy, 0 if we are neither charging nor */ 00662 /* // supplying energy. */ 00663 /* stg_meters_t range; // the range that our charging probe hit a charger */ 00664 /* } stg_energy_data_t; */ 00665 00666 /* typedef struct */ 00667 /* { */ 00668 /* stg_joules_t capacity; // maximum energy we can store (we start fully charged) */ 00669 /* stg_meters_t probe_range; // the length of our recharge probe */ 00670 /* //stg_pose_t probe_pose; // TODO - the origin of our probe */ 00671 00672 /* stg_watts_t give_rate; // give this many Watts to a probe that hits me (possibly 0) */ 00673 00674 /* stg_watts_t trickle_rate; // this much energy is consumed or */ 00675 /* // received by this device per second as a */ 00676 /* // baseline trickle. Positive values mean */ 00677 /* // that the device is just burning energy */ 00678 /* // stayting alive, which is appropriate */ 00679 /* // for most devices. Negative values mean */ 00680 /* // that the device is receiving energy */ 00681 /* // from the environment, simulating a */ 00682 /* // solar cell or some other ambient energy */ 00683 /* // collector */ 00684 00685 /* } stg_energy_config_t; */ 00686 00687 00688 /* // BLINKENLIGHT ------------------------------------------------------------ */ 00689 00690 /* // a number of milliseconds, used for example as the blinkenlight interval */ 00691 /* #define STG_LIGHT_ON UINT_MAX */ 00692 /* #define STG_LIGHT_OFF 0 */ 00693 00694 //typedef int stg_interval_ms_t; 00695 00696 00697 /* // TOKEN ----------------------------------------------------------------------- */ 00698 /* // token stuff for parsing worldfiles - this may one day replace 00699 the worldfile c++ code */ 00700 00701 /* #define CFG_OPEN '(' */ 00702 /* #define CFG_CLOSE ')' */ 00703 /* #define STR_OPEN '\"' */ 00704 /* #define STR_CLOSE '\"' */ 00705 /* #define TPL_OPEN '[' */ 00706 /* #define TPL_CLOSE ']' */ 00707 00708 /* typedef enum { */ 00709 /* STG_T_NUM = 0, */ 00710 /* STG_T_BOOLEAN, */ 00711 /* STG_T_MODELPROP, */ 00712 /* STG_T_WORLDPROP, */ 00713 /* STG_T_NAME, */ 00714 /* STG_T_STRING, */ 00715 /* STG_T_KEYWORD, */ 00716 /* STG_T_CFG_OPEN, */ 00717 /* STG_T_CFG_CLOSE, */ 00718 /* STG_T_TPL_OPEN, */ 00719 /* STG_T_TPL_CLOSE, */ 00720 /* } stg_token_type_t; */ 00721 00722 00723 00724 00725 /* typedef struct stg_token */ 00726 /* { */ 00727 /* char* token; ///< the text of the token */ 00728 /* stg_token_type_t type; ///< the type of the token */ 00729 /* unsigned int line; ///< the line on which the token appears */ 00730 00731 /* struct stg_token* next; ///< linked list support */ 00732 /* struct stg_token* child; ///< tree support */ 00733 00734 /* } stg_token_t; */ 00735 00736 /* stg_token_t* stg_token_next( stg_token_t* tokens ); */ 00737 /* /// print [token] formatted for a human reader, with a string [prefix] */ 00738 /* void stg_token_print( char* prefix, stg_token_t* token ); */ 00739 00740 /* /// print a token array suitable for human reader */ 00741 /* void stg_tokens_print( stg_token_t* tokens ); */ 00742 /* void stg_tokens_free( stg_token_t* tokens ); */ 00743 00744 /* /// create a new token structure from the arguments */ 00745 /* stg_token_t* stg_token_create( const char* token, stg_token_type_t type, int line ); */ 00746 00747 /* /// add a token to a list */ 00748 /* stg_token_t* stg_token_append( stg_token_t* head, */ 00749 /* char* token, stg_token_type_t type, int line ); */ 00750 00751 /* const char* stg_token_type_string( stg_token_type_t type ); */ 00752 00753 /* const char* stg_model_type_string( stg_model_type_t type ); */ 00754 00755 /* // functions for parsing worldfiles */ 00756 /* stg_token_t* stg_tokenize( FILE* wf ); */ 00757 /* //StgWorld* sc_load_worldblock( stg_client_t* cli, stg_token_t** tokensptr ); */ 00758 /* //stg_model_t* sc_load_modelblock( StgWorld* world, stg_model_t* parent, */ 00759 /* // stg_token_t** tokensptr ); */ 00760 00761 00762 00763 00764 //#ifdef __cplusplus 00765 //} 00766 //#endif 00767 00768 00769 // list iterator macros 00770 #define LISTFUNCTION( LIST, TYPE, FUNC ) for( GList* it=LIST; it; it=it->next ) FUNC((TYPE)it->data); 00771 00772 #define LISTMETHOD( LIST, TYPE, METHOD ) for( GList* it=LIST; it; it=it->next ) ((TYPE)it->data)->METHOD(); 00773 00774 #define LISTFUNCTIONARG( LIST, TYPE, FUNC, ARG ) for( GList* it=LIST; it; it=it->next ) FUNC((TYPE)it->data, ARG); 00775 00776 #define LISTMETHODARG( LIST, TYPE, METHOD, ARG ) for( GList* it=LIST; it; it=it->next ) ((TYPE)it->data)->METHOD(ARG); 00777 00778 00779 // Error macros - output goes to stderr 00780 #define PRINT_ERR(m) fprintf( stderr, "\033[41merr\033[0m: "m" (%s %s)\n", __FILE__, __FUNCTION__) 00781 #define PRINT_ERR1(m,a) fprintf( stderr, "\033[41merr\033[0m: "m" (%s %s)\n", a, __FILE__, __FUNCTION__) 00782 #define PRINT_ERR2(m,a,b) fprintf( stderr, "\033[41merr\033[0m: "m" (%s %s)\n", a, b, __FILE__, __FUNCTION__) 00783 #define PRINT_ERR3(m,a,b,c) fprintf( stderr, "\033[41merr\033[0m: "m" (%s %s)\n", a, b, c, __FILE__, __FUNCTION__) 00784 #define PRINT_ERR4(m,a,b,c,d) fprintf( stderr, "\033[41merr\033[0m: "m" (%s %s)\n", a, b, c, d, __FILE__, __FUNCTION__) 00785 #define PRINT_ERR5(m,a,b,c,d,e) fprintf( stderr, "\033[41merr\033[0m: "m" (%s %s)\n", a, b, c, d, e, __FILE__, __FUNCTION__) 00786 00787 // Warning macros 00788 #define PRINT_WARN(m) printf( "\033[44mwarn\033[0m: "m" (%s %s)\n", __FILE__, __FUNCTION__) 00789 #define PRINT_WARN1(m,a) printf( "\033[44mwarn\033[0m: "m" (%s %s)\n", a, __FILE__, __FUNCTION__) 00790 #define PRINT_WARN2(m,a,b) printf( "\033[44mwarn\033[0m: "m" (%s %s)\n", a, b, __FILE__, __FUNCTION__) 00791 #define PRINT_WARN3(m,a,b,c) printf( "\033[44mwarn\033[0m: "m" (%s %s)\n", a, b, c, __FILE__, __FUNCTION__) 00792 #define PRINT_WARN4(m,a,b,c,d) printf( "\033[44mwarn\033[0m: "m" (%s %s)\n", a, b, c, d, __FILE__, __FUNCTION__) 00793 #define PRINT_WARN5(m,a,b,c,d,e) printf( "\033[44mwarn\033[0m: "m" (%s %s)\n", a, b, c, d, e, __FILE__, __FUNCTION__) 00794 00795 // Message macros 00796 #ifdef DEBUG 00797 #define PRINT_MSG(m) printf( "Stage: "m" (%s %s)\n", __FILE__, __FUNCTION__) 00798 #define PRINT_MSG1(m,a) printf( "Stage: "m" (%s %s)\n", a, __FILE__, __FUNCTION__) 00799 #define PRINT_MSG2(m,a,b) printf( "Stage: "m" (%s %s)\n", a, b, __FILE__, __FUNCTION__) 00800 #define PRINT_MSG3(m,a,b,c) printf( "Stage: "m" (%s %s)\n", a, b, c, __FILE__, __FUNCTION__) 00801 #define PRINT_MSG4(m,a,b,c,d) printf( "Stage: "m" (%s %s)\n", a, b, c, d, __FILE__, __FUNCTION__) 00802 #define PRINT_MSG5(m,a,b,c,d,e) printf( "Stage: "m" (%s %s)\n", a, b, c, d, e,__FILE__, __FUNCTION__) 00803 #else 00804 #define PRINT_MSG(m) printf( "Stage: "m"\n" ) 00805 #define PRINT_MSG1(m,a) printf( "Stage: "m"\n", a) 00806 #define PRINT_MSG2(m,a,b) printf( "Stage: "m"\n,", a, b ) 00807 #define PRINT_MSG3(m,a,b,c) printf( "Stage: "m"\n", a, b, c ) 00808 #define PRINT_MSG4(m,a,b,c,d) printf( "Stage: "m"\n", a, b, c, d ) 00809 #define PRINT_MSG5(m,a,b,c,d,e) printf( "Stage: "m"\n", a, b, c, d, e ) 00810 #endif 00811 00812 // DEBUG macros 00813 #ifdef DEBUG 00814 #define PRINT_DEBUG(m) printf( "debug: "m" (%s %s)\n", __FILE__, __FUNCTION__) 00815 #define PRINT_DEBUG1(m,a) printf( "debug: "m" (%s %s)\n", a, __FILE__, __FUNCTION__) 00816 #define PRINT_DEBUG2(m,a,b) printf( "debug: "m" (%s %s)\n", a, b, __FILE__, __FUNCTION__) 00817 #define PRINT_DEBUG3(m,a,b,c) printf( "debug: "m" (%s %s)\n", a, b, c, __FILE__, __FUNCTION__) 00818 #define PRINT_DEBUG4(m,a,b,c,d) printf( "debug: "m" (%s %s)\n", a, b, c ,d, __FILE__, __FUNCTION__) 00819 #define PRINT_DEBUG5(m,a,b,c,d,e) printf( "debug: "m" (%s %s)\n", a, b, c ,d, e, __FILE__, __FUNCTION__) 00820 #else 00821 #define PRINT_DEBUG(m) 00822 #define PRINT_DEBUG1(m,a) 00823 #define PRINT_DEBUG2(m,a,b) 00824 #define PRINT_DEBUG3(m,a,b,c) 00825 #define PRINT_DEBUG4(m,a,b,c,d) 00826 #define PRINT_DEBUG5(m,a,b,c,d,e) 00827 #endif 00828 00829 class StgBlock; 00830 class StgModel; 00831 00832 00833 stg_msec_t stg_realtime(); 00834 stg_msec_t stg_realtime_since_start( void ); 00835 00836 00837 00838 // ANCESTOR CLASS 00839 00842 typedef int (*stg_model_callback_t)( StgModel* mod, void* user ); 00843 00844 00846 class StgAncestor 00847 { 00848 friend class StgCanvas; // allow StgCanvas access to our private members 00849 00850 protected: 00851 GList* children; 00852 00853 char* token; 00854 bool debug; 00855 00856 public: 00857 00859 void ForEachDescendant( stg_model_callback_t func, void* arg ); 00860 00862 unsigned int child_type_counts[MODEL_TYPE_COUNT]; 00863 00864 StgAncestor(); 00865 virtual ~StgAncestor(); 00866 00867 // unsigned int GetNumChildrenOfType( const char* typestr ); 00868 // void IncrementNumChildrenOfType( const char* typestr ); 00869 00870 virtual void AddChild( StgModel* mod ); 00871 virtual void RemoveChild( StgModel* mod ); 00872 virtual stg_pose_t GetGlobalPose(); 00873 00874 const char* Token() 00875 { return this->token; } 00876 00877 void SetToken( const char* str ) 00878 { 00879 this->token = strdup( str ); 00880 } 00881 00882 // // PURE VIRTUAL - descendents must implement 00883 // virtual void PushColor( stg_color_t col ) = 0; 00884 // virtual void PushColor( double r, double g, double b, double a ) = 0; 00885 // virtual void PopColor() = 0; // does nothing 00886 }; 00887 00888 00889 typedef struct 00890 { 00891 int enabled; 00892 stg_pose_t pose; 00893 stg_meters_t size; 00894 stg_color_t color; 00895 stg_msec_t period; 00896 double duty_cycle; 00897 } stg_blinkenlight_t; 00898 00899 typedef struct 00900 { 00901 uint32_t counter; 00902 GSList** lists; 00903 } stg_bigblock_t; 00904 00905 typedef struct 00906 { 00907 StgWorld* world; 00908 StgBlock* block; 00909 } stg_render_info_t; 00910 00911 00912 class StgBlockGrid 00913 { 00914 private: 00915 //stg_bigblock_t* map; 00916 GSList** cells; 00917 00918 //GTrashStack* trashstack; 00919 uint32_t width, height;// bwidth, bheight; 00920 00921 public: 00922 //uint32_t numbits; 00923 StgBlockGrid( uint32_t width, uint32_t height ); 00924 ~StgBlockGrid(); 00925 void AddBlock( uint32_t x, uint32_t y, StgBlock* block ); 00926 void RemoveBlock( uint32_t x, uint32_t y, StgBlock* block ); 00927 GSList* GetList( uint32_t x, uint32_t y ); 00928 void GlobalRemoveBlock( StgBlock* block ); 00929 void Draw( bool drawall ); 00930 00933 //uint32_t BigBlockOccupancy( uint32_t bbx, uint32_t bby ); 00934 }; 00935 00938 typedef struct 00939 { 00940 stg_pose_t pose; 00941 stg_meters_t range; 00942 StgBlock* block; 00943 } stg_raytrace_sample_t; 00944 00945 00946 const uint32_t INTERVAL_LOG_LEN = 32; 00947 00948 class Region; 00949 class SuperRegion; 00950 00952 class StgWorld : public StgAncestor 00953 { 00954 friend class StgModel; // allow access to private members 00955 friend class StgBlock; 00956 friend class StgTime; 00957 friend class StgCanvas; 00958 00959 private: 00960 static GList* world_list; 00961 00963 GQueue* csstack; 00964 00965 void PushPose(); 00966 void PopPose(); 00967 stg_pose_t* PeekPose(); 00968 void ShiftPose( stg_pose_t* pose ); 00969 00970 void DrawPose(); 00971 00972 static bool quit_all; 00973 static unsigned int next_id; 00974 static int AddBlockPixel( int x, int y, int z, 00975 stg_render_info_t* rinfo ) ; //< used as a callback by StgModel 00976 00977 stg_usec_t real_time_start; 00978 00979 bool quit; 00980 00982 int32_t MetersToPixels( stg_meters_t x ){ return (int32_t)floor(x * ppm) ; }; 00983 00985 void Initialize( const char* token, 00986 stg_msec_t interval_sim, 00987 double ppm ); 00988 00989 // dummy implementations to be overloaded by GUI subclasses 00990 virtual void PushColor( stg_color_t col ) { /* do nothing */ }; 00991 virtual void PushColor( double r, double g, double b, double a ) { /* do nothing */ }; 00992 virtual void PopColor(){ /* do nothing */ }; 00993 00994 00996 stg_usec_t real_time_now; 00997 00999 stg_usec_t quit_time; 01000 01001 bool destroy; 01002 01003 GHashTable* models_by_name; 01004 GList* velocity_list; 01005 01006 bool dirty; 01007 01008 int total_subs; 01009 double ppm; 01010 01011 void StartUpdatingModel( StgModel* mod ); 01012 void StopUpdatingModel( StgModel* mod ); 01013 01014 SuperRegion* CreateSuperRegion( int32_t x, int32_t y ); 01015 void DestroySuperRegion( SuperRegion* sr ); 01016 01017 void Raytrace( stg_pose_t pose, 01018 stg_meters_t range, 01019 stg_block_match_func_t func, 01020 StgModel* finder, 01021 const void* arg, 01022 stg_raytrace_sample_t* sample, 01023 bool ztest ); 01024 01025 void Raytrace( stg_pose_t pose, 01026 stg_meters_t range, 01027 stg_radians_t fov, 01028 stg_block_match_func_t func, 01029 StgModel* finder, 01030 const void* arg, 01031 stg_raytrace_sample_t* samples, 01032 uint32_t sample_count, 01033 bool ztest ); 01034 01035 void RemoveBlock( int x, int y, StgBlock* block ); 01036 01037 protected: 01038 GHashTable* superregions; 01039 GList* update_list; //< the descendants that need Update() called 01040 stg_usec_t interval_sim; 01041 01042 static void UpdateCb( StgWorld* world); 01043 01044 stg_usec_t sim_time; 01045 inline bool PastQuitTime() const { return (quit_time > 0) && (sim_time >= quit_time); } 01046 01047 GList* ray_list; 01048 // store rays traced for debugging purposes 01049 void RecordRay( double x1, double y1, double x2, double y2 ); 01050 Worldfile* wf; 01051 bool graphics; 01052 stg_bounds3d_t extent; 01053 01055 void Extend( stg_point3_t pt ); 01056 01057 //GHashTable* blocks; 01058 GArray lines; 01059 01060 virtual void AddModel( StgModel* mod ); 01061 virtual void RemoveModel( StgModel* mod ); 01062 01063 GList* GetRayList(){ return ray_list; }; 01064 void ClearRays(); 01065 01066 long unsigned int updates; 01067 01068 FileManager* fileMan; 01069 01070 public: 01071 static const int DEFAULT_PPM = 50; // default resolution in pixels per meter 01072 static const stg_msec_t DEFAULT_INTERVAL_SIM = 100; 01073 static bool UpdateAll(); //returns true when time to quit, false otherwise 01074 01075 StgWorld( const char* token = "MyWorld", 01076 stg_msec_t interval_sim = DEFAULT_INTERVAL_SIM, 01077 double ppm = DEFAULT_PPM ); 01078 01079 virtual ~StgWorld(); 01080 01081 stg_usec_t SimTimeNow(void){ return sim_time; } 01082 stg_usec_t RealTimeNow(void); 01083 stg_usec_t RealTimeSinceStart(void); 01084 // void PauseUntilNextUpdateTime(void); 01085 // void IdleUntilNextUpdateTime( int (*idler)(void) ); 01086 01087 void AddBlock( StgBlock* block ); 01088 void RemoveBlock( StgBlock* block ); 01089 01090 stg_usec_t GetSimInterval(){ return interval_sim; }; 01091 01092 Worldfile* GetWorldFile(){ return wf; }; 01093 01094 inline virtual bool IsGUI() { return false; } 01095 01096 virtual void Load( const char* worldfile_path ); 01097 virtual void UnLoad(); 01098 virtual void Reload(); 01099 virtual bool Save( const char* filename ); 01100 virtual bool Update(void); 01101 01102 bool TestQuit(){ return( quit || quit_all ); } 01103 void Quit(){ quit = true; } 01104 void QuitAll(){ quit_all = true; } 01105 void CancelQuit(){ quit = false; } 01106 void CancelQuitAll(){ quit_all = false; } 01107 01110 double Resolution(){ return ppm; }; 01111 01114 //StgModel* GetModel( const stg_id_t id ); 01115 01118 StgModel* GetModel( const char* name ); 01119 01121 stg_bounds3d_t GetExtent(){ return extent; }; 01122 01124 long unsigned int GetUpdateCount() { return updates; } 01125 }; 01126 01127 01128 typedef struct { 01129 stg_pose_t pose; 01130 stg_color_t color; 01131 stg_usec_t time; 01132 } stg_trail_item_t; 01133 01134 typedef int ctrlinit_t( StgModel* mod ); 01135 //typedef void ctrlupdate_t( StgModel* mod ); 01136 01138 class StgModel : public StgAncestor 01139 { 01140 friend class StgAncestor; 01141 friend class StgWorld; 01142 friend class StgWorldGui; 01143 friend class StgCanvas; 01144 friend class StgBlock; 01145 01146 private: 01148 static uint32_t count; 01149 static GHashTable* modelsbyid; 01151 uint32_t id; 01152 std::vector<Option*> drawOptions; 01153 const std::vector<Option*>& getOptions() const { return drawOptions; } 01154 01155 protected: 01156 stg_pose_t pose; 01157 stg_velocity_t velocity; 01158 stg_watts_t watts; //< power consumed by this model 01159 stg_color_t color; 01160 stg_kg_t mass; 01161 stg_geom_t geom; 01162 stg_laser_return_t laser_return; 01163 int obstacle_return; 01164 int blob_return; 01165 int gripper_return; 01166 int ranger_return; 01167 int fiducial_return; 01168 int fiducial_key; 01169 int boundary; 01170 stg_meters_t map_resolution; 01171 stg_bool_t stall; 01172 01174 char* say_string; 01175 01176 bool on_velocity_list; 01177 01178 int gui_nose; 01179 int gui_grid; 01180 int gui_outline; 01181 int gui_mask; 01182 01184 void registerOption( Option* opt ) { drawOptions.push_back( opt ); } 01185 01186 01187 StgModel* parent; //< the model that owns this one, possibly NULL 01188 01192 GData* props; 01193 01197 GHashTable* callbacks; 01198 01199 int subs; //< the number of subscriptions to this model 01200 01201 stg_usec_t interval; //< time between updates in us 01202 stg_usec_t last_update; //< time of last update in us 01203 01204 stg_bool_t disabled; //< if non-zero, the model is disabled 01205 01206 // GList* d_list; 01207 GList* blocks; //< list of stg_block_t structs that comprise a body 01208 01209 GArray* trail; 01210 01211 bool rebuild_displaylist; //< iff true, regenerate block display list before redraw 01212 01213 stg_pose_t global_pose; 01214 01215 bool gpose_dirty; //< set this to indicate that global pose may have 01216 //changed 01217 01218 bool data_fresh; 01219 01220 01221 01222 01223 //stg_id_t id; // globally unique ID used as hash table key and 01224 // worldfile section identifier 01225 01226 StgWorld* world; // pointer to the world in which this model exists 01227 01232 StgModel* TestCollision( stg_pose_t pose, 01233 stg_meters_t* hitx, 01234 stg_meters_t* hity ); 01235 01240 StgModel* TestCollision( stg_meters_t* hitx, 01241 stg_meters_t* hity ); 01242 01243 void Map(); 01244 void UnMap(); 01245 01246 void MapWithChildren(); 01247 void UnMapWithChildren(); 01248 01249 int TreeToPtrArray( GPtrArray* array ); 01250 01253 void Raytrace( stg_pose_t pose, 01254 stg_meters_t range, 01255 stg_block_match_func_t func, 01256 const void* arg, 01257 stg_raytrace_sample_t* sample, 01258 bool ztest = true ); 01259 01262 void Raytrace( stg_pose_t pose, 01263 stg_meters_t range, 01264 stg_radians_t fov, 01265 stg_block_match_func_t func, 01266 const void* arg, 01267 stg_raytrace_sample_t* samples, 01268 uint32_t sample_count, 01269 bool ztest = true ); 01270 01271 void Raytrace( stg_radians_t bearing, 01272 stg_meters_t range, 01273 stg_block_match_func_t func, 01274 const void* arg, 01275 stg_raytrace_sample_t* sample, 01276 bool ztest = true ); 01277 01278 void Raytrace( stg_radians_t bearing, 01279 stg_meters_t range, 01280 stg_radians_t fov, 01281 stg_block_match_func_t func, 01282 const void* arg, 01283 stg_raytrace_sample_t* samples, 01284 uint32_t sample_count, 01285 bool ztest = true ); 01286 01287 01291 void GPoseDirtyTree(); 01292 01293 virtual void Startup(); 01294 virtual void Shutdown(); 01295 virtual void Update(); 01296 virtual void UpdatePose(); 01297 01298 void DrawBlocksTree(); 01299 virtual void DrawBlocks(); 01300 virtual void DrawStatus( StgCamera* cam ); 01301 void DrawStatusTree( StgCamera* cam ); 01302 01303 void DrawOriginTree(); 01304 void DrawOrigin(); 01305 01306 void PushLocalCoords(); 01307 void PopCoords(); 01308 01310 void DrawImage( uint32_t texture_id, Stg::StgCamera* cam, float alpha ); 01311 01312 01313 // static wrapper for DrawBlocks() 01314 static void DrawBlocks( gpointer dummykey, 01315 StgModel* mod, 01316 void* arg ); 01317 01318 virtual void DrawPicker(); 01319 virtual void DataVisualize( StgCamera* cam ); 01320 01321 virtual void DrawSelected(void); 01322 01323 void DrawTrailFootprint(); 01324 void DrawTrailBlocks(); 01325 void DrawTrailArrows(); 01326 void DrawGrid(); 01327 void UpdateIfDue(); 01328 01329 /* hooks for attaching special callback functions (not used as 01330 variables) */ 01331 char startup_hook, shutdown_hook, load_hook, save_hook, update_hook; 01332 01333 ctrlinit_t* initfunc; 01334 01335 GList* flag_list; 01336 01337 Worldfile* wf; 01338 int wf_entity; 01339 01340 GPtrArray* blinkenlights; 01341 void DrawBlinkenlights(); 01342 01344 int blocks_dl; 01345 01347 //void BuildDisplayList(); 01348 01349 stg_model_type_t type; 01350 static const char* typestr; 01351 01352 void DataVisualizeTree( StgCamera* cam ); 01353 01354 virtual void PushColor( stg_color_t col ) 01355 { world->PushColor( col ); } 01356 01357 virtual void PushColor( double r, double g, double b, double a ) 01358 { world->PushColor( r,g,b,a ); } 01359 01360 virtual void PopColor(){ world->PopColor(); } 01361 01362 void DrawFlagList(); 01363 01364 void PushMyPose(); 01365 void PopPose(); 01366 void ShiftPose( stg_pose_t* pose ); 01367 void ShiftToTop(); 01368 01369 public: 01370 void PlaceInFreeSpace( stg_meters_t xmin, stg_meters_t xmax, 01371 stg_meters_t ymin, stg_meters_t ymax ); 01372 01374 static StgModel* LookupId( uint32_t id ) 01375 { return (StgModel*)g_hash_table_lookup( modelsbyid, (void*)id ); } 01376 01377 StgModel( StgWorld* world, StgModel* parent, stg_model_type_t type = MODEL_TYPE_PLAIN ); 01378 virtual ~StgModel(); 01379 01380 void Say( const char* str ); 01381 01382 void Load( Worldfile* wf, int wf_entity ) 01383 { 01386 SetWorldfile( wf, wf_entity ); 01387 Load(); // call virtual load 01388 } 01389 01391 void SetWorldfile( Worldfile* wf, int wf_entity ) 01392 { this->wf = wf; this->wf_entity = wf_entity; } 01393 01395 virtual void Load(); 01396 01398 virtual void Save(); 01399 01401 void Init(); 01402 01403 void AddFlag( StgFlag* flag ); 01404 void RemoveFlag( StgFlag* flag ); 01405 01406 void PushFlag( StgFlag* flag ); 01407 StgFlag* PopFlag(); 01408 01409 int GetFlagCount(){ return g_list_length( flag_list ); } 01410 01411 void AddBlinkenlight( stg_blinkenlight_t* b ) 01412 { 01413 g_ptr_array_add( this->blinkenlights, b ); 01414 } 01415 01416 void ClearBlinkenlights() 01417 { 01418 g_ptr_array_set_size( this->blinkenlights, 0 ); 01419 } 01420 01421 void Enable(){ disabled = false; }; 01422 void Disable(){ disabled = true; }; 01423 01424 // Load a control program for this model from an external library 01425 void LoadControllerModule( char* lib ); 01426 01427 // call this to ensure the GUI window is redrawn 01428 void NeedRedraw(); 01429 01430 void AddBlock( stg_point_t* pts, 01431 size_t pt_count, 01432 stg_meters_t zmin, 01433 stg_meters_t zmax, 01434 stg_color_t color, 01435 bool inherit_color ); 01436 01437 void AddBlockRect( double x, double y, 01438 double width, double height ); 01439 01441 void ClearBlocks(); 01442 01443 //const char* TypeStr(){ return this->typestr; } 01444 StgModel* Parent(){ return this->parent; } 01445 StgModel* GetModel( const char* name ); 01446 int GuiMask(){ return this->gui_mask; }; 01447 inline StgWorld* GetWorld(){ return this->world; } 01448 01450 StgModel* Root() 01451 { return( parent ? parent->Root() : this ); } 01452 01453 bool ObstacleReturn(){ return obstacle_return; } 01454 stg_laser_return_t GetLaserReturn(){ return laser_return; } 01455 int GetRangerReturn(){ return ranger_return; } 01456 int FiducialReturn(){ return fiducial_return; } 01457 int FiducialKey(){ return fiducial_key; } 01458 01459 bool IsAntecedent( StgModel* testmod ); 01460 01461 // returns true if model [testmod] is a descendent of model [mod] 01462 bool IsDescendent( StgModel* testmod ); 01463 01464 bool IsRelated( StgModel* mod2 ); 01465 01467 stg_pose_t GetGlobalPose(); 01468 01470 stg_velocity_t GetGlobalVelocity(); 01471 01472 /* set the velocity of a model in the global coordinate system */ 01473 void SetGlobalVelocity( stg_velocity_t gvel ); 01474 01476 void Subscribe(); 01477 01479 void Unsubscribe(); 01480 01482 void SetGlobalPose( stg_pose_t gpose ); 01483 01485 void SetVelocity( stg_velocity_t vel ); 01486 01488 void SetPose( stg_pose_t pose ); 01489 01491 void AddToPose( stg_pose_t pose ); 01492 01494 void AddToPose( double dx, double dy, double dz, double da ); 01495 01497 void SetGeom( stg_geom_t src ); 01498 01500 void SetFiducialReturn( int fid ); 01501 01504 void SetFiducialKey( int key ); 01505 01506 stg_color_t GetColor(){ return color; } 01507 01508 // stg_laser_return_t GetLaserReturn(){ return laser_return; } 01509 01511 int SetParent( StgModel* newparent); 01512 01515 stg_geom_t GetGeom(){ return geom; } 01516 01518 stg_pose_t GetPose(){ return pose; } 01519 01521 stg_velocity_t GetVelocity(){ return velocity; } 01522 01523 // guess what these do? 01524 void SetColor( stg_color_t col ); 01525 void SetMass( stg_kg_t mass ); 01526 void SetStall( stg_bool_t stall ); 01527 void SetGripperReturn( int val ); 01528 void SetLaserReturn( stg_laser_return_t val ); 01529 void SetObstacleReturn( int val ); 01530 void SetBlobReturn( int val ); 01531 void SetRangerReturn( int val ); 01532 void SetBoundary( int val ); 01533 void SetGuiNose( int val ); 01534 void SetGuiMask( int val ); 01535 void SetGuiGrid( int val ); 01536 void SetGuiOutline( int val ); 01537 void SetWatts( stg_watts_t watts ); 01538 void SetMapResolution( stg_meters_t res ); 01539 01540 bool DataIsFresh(){ return this->data_fresh; } 01541 01542 /* attach callback functions to data members. The function gets 01543 called when the member is changed using SetX() accessor method */ 01544 01545 void AddCallback( void* address, 01546 stg_model_callback_t cb, 01547 void* user ); 01548 01549 int RemoveCallback( void* member, 01550 stg_model_callback_t callback ); 01551 01552 void CallCallbacks( void* address ); 01553 01554 /* wrappers for the generic callback add & remove functions that hide 01555 some implementation detail */ 01556 01557 void AddStartupCallback( stg_model_callback_t cb, void* user ) 01558 { AddCallback( &startup_hook, cb, user ); }; 01559 01560 void RemoveStartupCallback( stg_model_callback_t cb ) 01561 { RemoveCallback( &startup_hook, cb ); }; 01562 01563 void AddShutdownCallback( stg_model_callback_t cb, void* user ) 01564 { AddCallback( &shutdown_hook, cb, user ); }; 01565 01566 void RemoveShutdownCallback( stg_model_callback_t cb ) 01567 { RemoveCallback( &shutdown_hook, cb ); } 01568 01569 void AddLoadCallback( stg_model_callback_t cb, void* user ) 01570 { AddCallback( &load_hook, cb, user ); } 01571 01572 void RemoveLoadCallback( stg_model_callback_t cb ) 01573 { RemoveCallback( &load_hook, cb ); } 01574 01575 void AddSaveCallback( stg_model_callback_t cb, void* user ) 01576 { AddCallback( &save_hook, cb, user ); } 01577 01578 void RemoveSaveCallback( stg_model_callback_t cb ) 01579 { RemoveCallback( &save_hook, cb ); } 01580 01581 void AddUpdateCallback( stg_model_callback_t cb, void* user ) 01582 { AddCallback( &update_hook, cb, user ); } 01583 01584 void RemoveUpdateCallback( stg_model_callback_t cb ) 01585 { RemoveCallback( &update_hook, cb ); } 01586 01589 void* GetProperty( char* key ); 01590 01621 int SetProperty( char* key, void* data ); 01622 void UnsetProperty( char* key ); 01623 01624 virtual void Print( char* prefix ); 01625 virtual const char* PrintWithPose(); 01626 01630 void GlobalToLocal( stg_pose_t* pose ); 01631 01635 //void LocalToGlobal( stg_pose_t* pose ); 01636 01639 stg_pose_t LocalToGlobal( stg_pose_t pose ); 01640 01643 stg_point3_t LocalToGlobal( stg_point3_t local ); 01644 01647 StgModel* GetUnsubscribedModelOfType( stg_model_type_t type ); 01648 01649 // Iff true, model may output some debugging visualizations and other info 01650 //bool debug; 01651 01654 bool Stalled(){ return this->stall; } 01655 }; 01656 01657 // BLOCKS 01658 class StgBlock 01659 { 01660 public: 01661 01665 StgBlock( StgModel* mod, 01666 stg_point_t* pts, 01667 size_t pt_count, 01668 stg_meters_t height, 01669 stg_meters_t z_offset, 01670 stg_color_t color, 01671 bool inherit_color ); 01672 01673 ~StgBlock(); 01674 01675 void Map(); 01676 void UnMap(); // draw the block into the world 01677 01678 void DrawGlobal(); // draw the block in OpenGL using pts_global coords 01679 void Draw(); // draw the block in OpenGL 01680 //void Draw2D(); // draw the block in OpenGL 01681 void DrawSolid(); // draw the block in OpenGL as a solid single color 01682 void DrawFootPrint(); // draw the projection of the block onto the z=0 plane 01683 01684 static void ScaleList( GList* blocks, stg_size_t* size ); 01685 void RecordRenderPoint( GSList** head, GSList* link, unsigned int* c1, unsigned int* c2 ); 01686 01687 StgModel* Model(){ return mod; }; 01688 01689 stg_point_t* Points( unsigned int *count ) 01690 { if( count ) *count = pt_count; return pts; }; 01691 01692 bool IntersectGlobalZ( stg_meters_t z ) 01693 { return( z >= global_zmin && z <= global_zmax ); } 01694 01695 stg_color_t Color() 01696 { return( inherit_color ? mod->GetColor() : color ); } 01697 01698 private: 01699 stg_point_t* pts; //< points defining a polygon 01700 size_t pt_count; //< the number of points 01701 stg_meters_t zmin; 01702 stg_meters_t zmax; 01703 01704 stg_meters_t global_zmin; 01705 stg_meters_t global_zmax; 01706 01707 StgModel* mod; //< model to which this block belongs 01708 01709 stg_point_int_t* pts_global_pixels; //< points defining a polygon in global coords 01710 01711 stg_color_t color; 01712 bool inherit_color; 01713 01714 GArray* rendered_points; 01715 01716 inline void DrawTop(); 01717 inline void DrawSides(); 01718 01719 inline void PushColor( stg_color_t col ) 01720 { mod->PushColor( col ); } 01721 01722 inline void PushColor( double r, double g, double b, double a ) 01723 { mod->PushColor( r,g,b,a ); } 01724 01725 inline void PopColor() 01726 { mod->PopColor(); } 01727 }; 01728 01729 // COLOR STACK CLASS 01730 class GlColorStack 01731 { 01732 public: 01733 GlColorStack(); 01734 ~GlColorStack(); 01735 01736 void Push( GLdouble col[4] ); 01737 void Push( double r, double g, double b, double a ); 01738 void Push( double r, double g, double b ); 01739 void Push( stg_color_t col ); 01740 01741 void Pop(); 01742 01743 unsigned int Length() 01744 { return g_queue_get_length( colorstack ); } 01745 01746 private: 01747 GQueue* colorstack; 01748 }; 01749 01750 class StgCamera 01751 { 01752 protected: 01753 float _pitch; //left-right (about y) 01754 float _yaw; //up-down (about x) 01755 float _x, _y, _z; 01756 01757 public: 01758 StgCamera() : _pitch( 0 ), _yaw( 0 ), _x( 0 ), _y( 0 ), _z( 0 ) { } 01759 virtual ~StgCamera() { } 01760 01761 virtual void Draw( void ) const = 0; 01762 virtual void SetProjection( void ) const = 0; 01763 01764 inline float yaw( void ) const { return _yaw; } 01765 inline float pitch( void ) const { return _pitch; } 01766 01767 inline float x( void ) const { return _x; } 01768 inline float y( void ) const { return _y; } 01769 inline float z( void ) const { return _z; } 01770 01771 virtual void reset() = 0; 01772 virtual void Load( Worldfile* wf, int sec ) = 0; 01773 01774 //TODO data should be passed in somehow else. (at least min/max stuff) 01775 //virtual void SetProjection( float pixels_width, float pixels_height, float y_min, float y_max ) const = 0; 01776 }; 01777 01778 class StgPerspectiveCamera : public StgCamera 01779 { 01780 private: 01781 float _z_near; 01782 float _z_far; 01783 float _vert_fov; 01784 float _horiz_fov; 01785 float _aspect; 01786 01787 public: 01788 StgPerspectiveCamera( void ); 01789 01790 virtual void Draw( void ) const; 01791 virtual void SetProjection( void ) const; 01792 //void SetProjection( float aspect ) const; 01793 void update( void ); 01794 01795 void strafe( float amount ); 01796 void forward( float amount ); 01797 01798 inline void setPose( float x, float y, float z ) { _x = x; _y = y; _z = z; } 01799 inline void addPose( float x, float y, float z ) { _x += x; _y += y; _z += z; if( _z < 0.1 ) _z = 0.1; } 01800 void move( float x, float y, float z ); 01801 inline void setFov( float horiz_fov, float vert_fov ) { _horiz_fov = horiz_fov; _vert_fov = vert_fov; } 01803 inline void setAspect( float aspect ) { 01804 //std::cout << "aspect: " << aspect << " vert: " << _vert_fov << " => " << aspect * _vert_fov << std::endl; 01805 //_vert_fov = aspect / _horiz_fov; 01806 _aspect = aspect; 01807 } 01808 inline void setYaw( float yaw ) { _yaw = yaw; } 01809 inline float horizFov( void ) const { return _horiz_fov; } 01810 inline float vertFov( void ) const { return _vert_fov; } 01811 inline void addYaw( float yaw ) { _yaw += yaw; } 01812 inline void setPitch( float pitch ) { _pitch = pitch; } 01813 inline void addPitch( float pitch ) { _pitch += pitch; if( _pitch < 0 ) _pitch = 0; else if( _pitch > 180 ) _pitch = 180; } 01814 01815 inline float realDistance( float z_buf_val ) const { 01816 //formulat found at http://www.cs.unc.edu/~hoff/techrep/openglz.html 01817 //Z = Zn*Zf / (Zf - z*(Zf-Zn)) 01818 return _z_near * _z_far / ( _z_far - z_buf_val * ( _z_far - _z_near ) ); 01819 } 01820 inline void scroll( float dy ) { _z += dy; } 01821 inline float nearClip( void ) const { return _z_near; } 01822 inline float farClip( void ) const { return _z_far; } 01823 inline void setClip( float near, float far ) { _z_far = far; _z_near = near; } 01824 01825 inline void reset() { setPitch( 70 ); setYaw( 0 ); } 01826 01827 void Load( Worldfile* wf, int sec ); 01828 void Save( Worldfile* wf, int sec ); 01829 }; 01830 01831 class StgOrthoCamera : public StgCamera 01832 { 01833 private: 01834 float _scale; 01835 01836 float _pixels_width; 01837 float _pixels_height; 01838 float _y_min; 01839 float _y_max; 01840 01841 public: 01842 StgOrthoCamera( void ) : _scale( 15 ) { } 01843 virtual void Draw() const; 01844 virtual void SetProjection( float pixels_width, float pixels_height, float y_min, float y_max ); 01845 virtual void SetProjection( void ) const; 01846 01847 void move( float x, float y ); 01848 inline void setYaw( float yaw ) { _yaw = yaw; } 01849 inline void setPitch( float pitch ) { _pitch = pitch; } 01850 inline void addYaw( float yaw ) { _yaw += yaw; } 01851 inline void addPitch( float pitch ) { 01852 _pitch += pitch; 01853 if( _pitch > 90 ) 01854 _pitch = 90; 01855 else if( _pitch < 0 ) 01856 _pitch = 0; 01857 } 01858 01859 inline void setScale( float scale ) { _scale = scale; } 01860 inline void setPose( float x, float y) { _x = x; _y = y; } 01861 01862 void scale( float scale, float shift_x = 0, float h = 0, float shift_y = 0, float w = 0 ); 01863 inline void reset( void ) { _pitch = _yaw = 0; } 01864 01865 inline float scale() const { return _scale; } 01866 01867 void Load( Worldfile* wf, int sec ); 01868 void Save( Worldfile* wf, int sec ); 01869 }; 01870 01871 class StgCanvas : public Fl_Gl_Window 01872 { 01873 friend class StgWorldGui; // allow access to private members 01874 friend class StgModel; 01875 01876 private: 01877 GlColorStack colorstack; 01878 01879 StgCamera* current_camera; 01880 StgOrthoCamera camera; 01881 StgPerspectiveCamera perspective_camera; 01882 bool dirty_buffer; 01883 Worldfile* wf; 01884 01885 int startx, starty; 01886 bool selectedModel; 01887 bool clicked_empty_space; 01888 int empty_space_startx, empty_space_starty; 01889 GList* selected_models; 01890 01891 StgModel* last_selection; 01892 01893 01894 stg_msec_t interval; // window refresh interval in ms 01895 01896 GList* ray_list; 01897 void RecordRay( double x1, double y1, double x2, double y2 ); 01898 void DrawRays(); 01899 void ClearRays(); 01900 void DrawGlobalGrid(); 01901 01902 Option 01903 showBlinken, 01904 showBlocks, 01905 showClock, 01906 showData, 01907 showFlags, 01908 showFollow, 01909 showFootprints, 01910 showGrid, 01911 showOccupancy, 01912 showScreenshots, 01913 showStatus, 01914 showTrailArrows, 01915 showTrailRise, 01916 showTrails, 01917 showTree, 01918 pCamOn, 01919 visualizeAll; 01920 01921 public: 01922 StgCanvas( StgWorldGui* world, int x, int y, int W,int H); 01923 ~StgCanvas(); 01924 01925 bool graphics; 01926 StgWorldGui* world; 01927 01928 void Screenshot(); 01929 01930 void createMenuItems( Fl_Menu_Bar* menu, std::string path ); 01931 01932 void FixViewport(int W,int H); 01933 void DrawFloor(); //simpler floor compared to grid 01934 void DrawBlocks(); 01935 void resetCamera(); 01936 virtual void renderFrame(); 01937 virtual void draw(); 01938 virtual int handle( int event ); 01939 void resize(int X,int Y,int W,int H); 01940 01941 void CanvasToWorld( int px, int py, 01942 double *wx, double *wy, double* wz ); 01943 01944 StgModel* getModel( int x, int y ); 01945 bool selected( StgModel* mod ); 01946 void select( StgModel* mod ); 01947 void unSelect( StgModel* mod ); 01948 void unSelectAll(); 01949 01950 inline void setDirtyBuffer( void ) { dirty_buffer = true; } 01951 inline bool dirtyBuffer( void ) const { return dirty_buffer; } 01952 01953 inline void PushColor( stg_color_t col ) 01954 { colorstack.Push( col ); } 01955 01956 void PushColor( double r, double g, double b, double a ) 01957 { colorstack.Push( r,g,b,a ); } 01958 01959 void PopColor(){ colorstack.Pop(); } 01960 01961 void InvertView( uint32_t invertflags ); 01962 01963 static void TimerCallback( StgCanvas* canvas ); 01964 static void perspectiveCb( Fl_Widget* w, void* p ); 01965 01966 void Load( Worldfile* wf, int section ); 01967 void Save( Worldfile* wf, int section ); 01968 }; 01969 01973 class StgWorldGui : public StgWorld, public Fl_Window 01974 { 01975 friend class StgCanvas; 01976 friend class StgModelCamera; 01977 01978 private: 01979 bool paused; 01980 //int wf_section; 01981 StgCanvas* canvas; 01982 Fl_Menu_Bar* mbar; 01983 OptionsDlg* oDlg; 01984 std::vector<Option*> drawOptions; 01985 void updateOptions(); 01986 stg_usec_t interval_log[INTERVAL_LOG_LEN]; 01987 01988 stg_usec_t real_time_of_last_update; 01989 stg_usec_t interval_real; 01990 01991 // static callback functions 01992 static void windowCb( Fl_Widget* w, void* p ); 01993 static void fileLoadCb( Fl_Widget* w, void* p ); 01994 static void fileSaveCb( Fl_Widget* w, void* p ); 01995 static void fileSaveAsCb( Fl_Widget* w, void* p ); 01996 static void fileExitCb( Fl_Widget* w, void* p ); 01997 static void viewOptionsCb( Fl_Widget* w, void* p ); 01998 static void optionsDlgCb( Fl_Widget* w, void* p ); 01999 static void helpAboutCb( Fl_Widget* w, void* p ); 02000 02001 // GUI functions 02002 bool saveAsDialog(); 02003 bool closeWindowQuery(); 02004 02005 // Quit time pause 02006 bool pause_time; 02007 02008 protected: 02009 virtual void PushColor( stg_color_t col ) 02010 { canvas->PushColor( col ); } 02011 02012 virtual void PushColor( double r, double g, double b, double a ) 02013 { canvas->PushColor( r,g,b,a ); } 02014 02015 virtual void PopColor() 02016 { canvas->PopColor(); } 02017 02018 void DrawTree( bool leaves ); 02019 void DrawFloor(); 02020 02021 StgCanvas* GetCanvas( void ) { return canvas; } 02022 02023 public: 02024 static const stg_msec_t DEFAULT_INTERVAL_REAL = 100; 02025 02026 StgWorldGui(int W,int H,const char*L=0); 02027 ~StgWorldGui(); 02028 02029 virtual bool Update(); 02030 02031 virtual void Load( const char* filename ); 02032 virtual void UnLoad(); 02033 virtual bool Save( const char* filename ); 02034 02035 inline virtual bool IsGUI() { return true; } 02036 02037 void Start(){ paused = false; }; 02038 void Stop(){ paused = true; }; 02039 void TogglePause(){ paused = !paused; }; 02040 02043 std::string ClockString( void ); 02044 02047 void SetRealTimeInterval( stg_usec_t usec ) 02048 { interval_real = usec; } 02049 }; 02050 02051 02052 // BLOBFINDER MODEL -------------------------------------------------------- 02053 02056 typedef struct 02057 { 02058 stg_color_t color; 02059 uint32_t left, top, right, bottom; 02060 stg_meters_t range; 02061 } stg_blobfinder_blob_t; 02062 02064 class StgModelBlobfinder : public StgModel 02065 { 02066 private: 02067 GArray* colors; 02068 GArray* blobs; 02069 02070 // predicate for ray tracing 02071 static bool BlockMatcher( StgBlock* testblock, StgModel* finder ); 02072 02073 static Option showBlobData; 02074 02075 virtual void DataVisualize( StgCamera* cam ); 02076 02077 public: 02078 unsigned int scan_width; 02079 unsigned int scan_height; 02080 stg_meters_t range; 02081 stg_radians_t fov; 02082 stg_radians_t pan; 02083 02084 static const char* typestr; 02085 02086 // constructor 02087 StgModelBlobfinder( StgWorld* world, 02088 StgModel* parent ); 02089 // destructor 02090 ~StgModelBlobfinder(); 02091 02092 virtual void Startup(); 02093 virtual void Shutdown(); 02094 virtual void Update(); 02095 virtual void Load(); 02096 02097 stg_blobfinder_blob_t* GetBlobs( unsigned int* count ) 02098 { 02099 if( count ) *count = blobs->len; 02100 return (stg_blobfinder_blob_t*)blobs->data; 02101 } 02102 02104 void AddColor( stg_color_t col ); 02105 02107 void RemoveColor( stg_color_t col ); 02108 02111 void RemoveAllColors(); 02112 }; 02113 02114 // ENERGY model -------------------------------------------------------------- 02115 02117 typedef struct 02118 { 02120 stg_joules_t stored; 02121 02123 stg_bool_t charging; 02124 02126 stg_meters_t range; 02127 02129 GPtrArray* connections; 02130 } stg_energy_data_t; 02131 02133 typedef struct 02134 { 02136 stg_joules_t capacity; 02137 02139 stg_watts_t give_rate; 02140 02142 stg_watts_t take_rate; 02143 02145 stg_meters_t probe_range; 02146 02148 stg_bool_t give; 02149 02150 } stg_energy_config_t; 02151 02152 // there is currently no energy command packet 02153 02154 02155 // LASER MODEL -------------------------------------------------------- 02156 02159 typedef struct 02160 { 02161 stg_meters_t range; 02162 double reflectance; 02163 } stg_laser_sample_t; 02164 02165 typedef struct 02166 { 02167 uint32_t sample_count; 02168 uint32_t resolution; 02169 stg_range_bounds_t range_bounds; 02170 stg_radians_t fov; 02171 stg_usec_t interval; 02172 } stg_laser_cfg_t; 02173 02175 class StgModelLaser : public StgModel 02176 { 02177 private: 02178 int dl_debug_laser; 02179 02181 int data_dl; 02182 bool data_dirty; 02183 02184 stg_laser_sample_t* samples; 02185 uint32_t sample_count; 02186 stg_meters_t range_min, range_max; 02187 stg_radians_t fov; 02188 uint32_t resolution; 02189 02190 static Option showLaserData; 02191 static Option showLaserStrikes; 02192 02193 public: 02194 static const char* typestr; 02195 // constructor 02196 StgModelLaser( StgWorld* world, 02197 StgModel* parent ); 02198 02199 // destructor 02200 ~StgModelLaser(); 02201 02202 virtual void Startup(); 02203 virtual void Shutdown(); 02204 virtual void Update(); 02205 virtual void Load(); 02206 virtual void Print( char* prefix ); 02207 virtual void DataVisualize( StgCamera* cam ); 02208 02209 uint32_t GetSampleCount(){ return sample_count; } 02210 02211 stg_laser_sample_t* GetSamples( uint32_t* count=NULL); 02212 02213 void SetSamples( stg_laser_sample_t* samples, uint32_t count); 02214 02215 // Get the user-tweakable configuration of the laser 02216 stg_laser_cfg_t GetConfig( ); 02217 02218 // Set the user-tweakable configuration of the laser 02219 void SetConfig( stg_laser_cfg_t cfg ); 02220 }; 02221 02222 // \todo GRIPPER MODEL -------------------------------------------------------- 02223 02224 // typedef enum { 02225 // STG_GRIPPER_PADDLE_OPEN = 0, // default state 02226 // STG_GRIPPER_PADDLE_CLOSED, 02227 // STG_GRIPPER_PADDLE_OPENING, 02228 // STG_GRIPPER_PADDLE_CLOSING, 02229 // } stg_gripper_paddle_state_t; 02230 02231 // typedef enum { 02232 // STG_GRIPPER_LIFT_DOWN = 0, // default state 02233 // STG_GRIPPER_LIFT_UP, 02234 // STG_GRIPPER_LIFT_UPPING, // verbed these to match the paddle state 02235 // STG_GRIPPER_LIFT_DOWNING, 02236 // } stg_gripper_lift_state_t; 02237 02238 // typedef enum { 02239 // STG_GRIPPER_CMD_NOP = 0, // default state 02240 // STG_GRIPPER_CMD_OPEN, 02241 // STG_GRIPPER_CMD_CLOSE, 02242 // STG_GRIPPER_CMD_UP, 02243 // STG_GRIPPER_CMD_DOWN 02244 // } stg_gripper_cmd_type_t; 02245 02246 // /** gripper configuration packet 02247 // */ 02248 // typedef struct 02249 // { 02250 // stg_size_t paddle_size; ///< paddle dimensions 02251 02252 // stg_gripper_paddle_state_t paddles; 02253 // stg_gripper_lift_state_t lift; 02254 02255 // double paddle_position; ///< 0.0 = full open, 1.0 full closed 02256 // double lift_position; ///< 0.0 = full down, 1.0 full up 02257 02258 // stg_meters_t inner_break_beam_inset; ///< distance from the end of the paddle 02259 // stg_meters_t outer_break_beam_inset; ///< distance from the end of the paddle 02260 // stg_bool_t paddles_stalled; // true iff some solid object stopped 02261 // // the paddles closing or opening 02262 02263 // GSList *grip_stack; ///< stack of items gripped 02264 // int grip_stack_size; ///< maximum number of objects in stack, or -1 for unlimited 02265 02266 // double close_limit; ///< How far the gripper can close. If < 1.0, the gripper has its mouth full. 02267 02268 // } stg_gripper_config_t; 02269 02270 // /** gripper command packet 02271 // */ 02272 // typedef struct 02273 // { 02274 // stg_gripper_cmd_type_t cmd; 02275 // int arg; 02276 // } stg_gripper_cmd_t; 02277 02278 02279 // /** gripper data packet 02280 // */ 02281 // typedef struct 02282 // { 02283 // stg_gripper_paddle_state_t paddles; 02284 // stg_gripper_lift_state_t lift; 02285 02286 // double paddle_position; ///< 0.0 = full open, 1.0 full closed 02287 // double lift_position; ///< 0.0 = full down, 1.0 full up 02288 02289 // stg_bool_t inner_break_beam; ///< non-zero iff beam is broken 02290 // stg_bool_t outer_break_beam; ///< non-zero iff beam is broken 02291 02292 // stg_bool_t paddle_contacts[2]; ///< non-zero iff paddles touch something 02293 02294 // stg_bool_t paddles_stalled; // true iff some solid object stopped 02295 // // the paddles closing or opening 02296 02297 // int stack_count; ///< number of objects in stack 02298 02299 02300 // } stg_gripper_data_t; 02301 02302 02303 // \todo BUMPER MODEL -------------------------------------------------------- 02304 02305 // typedef struct 02306 // { 02307 // stg_pose_t pose; 02308 // stg_meters_t length; 02309 // } stg_bumper_config_t; 02310 02311 // typedef struct 02312 // { 02313 // StgModel* hit; 02314 // stg_point_t hit_point; 02315 // } stg_bumper_sample_t; 02316 02317 02318 // FIDUCIAL MODEL -------------------------------------------------------- 02319 02322 typedef struct 02323 { 02324 stg_meters_t max_range_anon; //< maximum detection range 02325 stg_meters_t max_range_id; 02326 stg_meters_t min_range; 02327 stg_radians_t fov; 02328 stg_radians_t heading; 02329 02332 int key; 02333 } stg_fiducial_config_t; 02334 02337 typedef struct 02338 { 02339 stg_meters_t range; 02340 stg_radians_t bearing; 02341 stg_pose_t geom; 02342 stg_pose_t pose; 02343 int id; 02344 02345 } stg_fiducial_t; 02346 02348 class StgModelFiducial : public StgModel 02349 { 02350 private: 02351 // if neighbor is visible, add him to the fiducial scan 02352 void AddModelIfVisible( StgModel* him ); 02353 02354 // static wrapper function can be used as a function pointer 02355 static int AddModelIfVisibleStatic( StgModel* him, StgModelFiducial* me ) 02356 { if( him != me ) me->AddModelIfVisible( him ); return 0; } 02357 02358 virtual void Update(); 02359 virtual void DataVisualize( StgCamera* cam ); 02360 02361 GArray* data; 02362 02363 static Option showFiducialData; 02364 02365 public: 02366 static const char* typestr; 02367 // constructor 02368 StgModelFiducial( StgWorld* world, 02369 StgModel* parent ); 02370 // destructor 02371 virtual ~StgModelFiducial(); 02372 02373 virtual void Load(); 02374 void Shutdown( void ); 02375 02376 stg_meters_t max_range_anon; //< maximum detection range 02377 stg_meters_t max_range_id; 02378 stg_meters_t min_range; 02379 stg_radians_t fov; 02380 stg_radians_t heading; 02381 int key; 02382 02383 stg_fiducial_t* fiducials; 02384 uint32_t fiducial_count; 02385 }; 02386 02387 02388 // RANGER MODEL -------------------------------------------------------- 02389 02390 typedef struct 02391 { 02392 stg_pose_t pose; 02393 stg_size_t size; 02394 stg_bounds_t bounds_range; 02395 stg_radians_t fov; 02396 int ray_count; 02397 } stg_ranger_sensor_t; 02398 02400 class StgModelRanger : public StgModel 02401 { 02402 protected: 02403 02404 virtual void Startup(); 02405 virtual void Shutdown(); 02406 virtual void Update(); 02407 virtual void DataVisualize( StgCamera* cam ); 02408 02409 public: 02410 static const char* typestr; 02411 // constructor 02412 StgModelRanger( StgWorld* world, 02413 StgModel* parent ); 02414 // destructor 02415 virtual ~StgModelRanger(); 02416 02417 virtual void Load(); 02418 virtual void Print( char* prefix ); 02419 02420 uint32_t sensor_count; 02421 stg_ranger_sensor_t* sensors; 02422 stg_meters_t* samples; 02423 02424 private: 02425 static Option showRangerData; 02426 static Option showRangerTransducers; 02427 02428 }; 02429 02430 // BLINKENLIGHT MODEL ---------------------------------------------------- 02431 class StgModelBlinkenlight : public StgModel 02432 { 02433 private: 02434 double dutycycle; 02435 bool enabled; 02436 stg_msec_t period; 02437 bool on; 02438 02439 static Option showBlinkenData; 02440 public: 02441 02442 static const char* typestr; 02443 StgModelBlinkenlight( StgWorld* world, 02444 StgModel* parent ); 02445 02446 ~StgModelBlinkenlight(); 02447 02448 virtual void Load(); 02449 virtual void Update(); 02450 virtual void DataVisualize( StgCamera* cam ); 02451 }; 02452 02453 // CAMERA MODEL ---------------------------------------------------- 02454 typedef struct { 02455 // GL_V3F 02456 GLfloat x, y, z; 02457 } ColoredVertex; 02458 02460 class StgModelCamera : public StgModel 02461 { 02462 private: 02463 StgCanvas* _canvas; 02464 02465 GLfloat* _frame_data; //opengl read buffer 02466 GLubyte* _frame_color_data; //opengl read buffer 02467 02468 bool _valid_vertexbuf_cache; 02469 ColoredVertex* _vertexbuf_cache; //cached unit vectors with appropriate rotations (these must be scalled by z-buffer length) 02470 02471 int _width; //width of buffer 02472 int _height; //height of buffer 02473 static const int _depth = 4; 02474 02475 int _camera_quads_size; 02476 GLfloat* _camera_quads; 02477 GLubyte* _camera_colors; 02478 02479 static Option showCameraData; 02480 02481 StgPerspectiveCamera _camera; 02482 float _yaw_offset; //position camera is mounted at 02483 float _pitch_offset; 02484 02486 bool GetFrame(); 02487 02488 public: 02489 StgModelCamera( StgWorld* world, 02490 StgModel* parent ); 02491 02492 ~StgModelCamera(); 02493 02494 virtual void Load(); 02495 02497 virtual void Update(); 02498 02500 //virtual void Draw( uint32_t flags, StgCanvas* canvas ); 02501 02503 virtual void DataVisualize( StgCamera* cam ); 02504 02506 inline int getWidth( void ) const { return _width; } 02507 02509 inline int getHeight( void ) const { return _height; } 02510 02512 inline const StgPerspectiveCamera& getCamera( void ) const { return _camera; } 02513 02515 inline const GLfloat* FrameDepth() const { return _frame_data; } 02516 02518 inline const GLubyte* FrameColor() const { return _frame_color_data; } 02519 02521 inline void setPitch( float pitch ) { _pitch_offset = pitch; _valid_vertexbuf_cache = false; } 02522 02524 inline void setYaw( float yaw ) { _yaw_offset = yaw; _valid_vertexbuf_cache = false; } 02525 }; 02526 02527 // POSITION MODEL -------------------------------------------------------- 02528 02530 typedef enum 02531 { STG_POSITION_CONTROL_VELOCITY, 02532 STG_POSITION_CONTROL_POSITION 02533 } stg_position_control_mode_t; 02534 02536 typedef enum 02537 { STG_POSITION_LOCALIZATION_GPS, 02538 STG_POSITION_LOCALIZATION_ODOM 02539 } stg_position_localization_mode_t; 02540 02542 typedef enum 02543 { STG_POSITION_DRIVE_DIFFERENTIAL, 02544 STG_POSITION_DRIVE_OMNI, 02545 STG_POSITION_DRIVE_CAR 02546 } stg_position_drive_mode_t; 02547 02548 02550 class StgModelPosition : public StgModel 02551 { 02552 private: 02553 stg_pose_t goal; //< the current velocity or pose to reach, depending on the value of control_mode 02554 stg_position_control_mode_t control_mode; 02555 stg_position_drive_mode_t drive_mode; 02556 stg_position_localization_mode_t localization_mode; 02557 stg_velocity_t integration_error; 02558 public: 02559 static const char* typestr; 02560 // constructor 02561 StgModelPosition( StgWorld* world, 02562 StgModel* parent ); 02563 // destructor 02564 ~StgModelPosition(); 02565 02566 virtual void Startup(); 02567 virtual void Shutdown(); 02568 virtual void Update(); 02569 virtual void Load(); 02570 02572 void SetOdom( stg_pose_t odom ); 02573 02576 void SetSpeed( double x, double y, double a ); 02577 void SetXSpeed( double x ); 02578 void SetYSpeed( double y ); 02579 void SetZSpeed( double z ); 02580 void SetTurnSpeed( double a ); 02581 void SetSpeed( stg_velocity_t vel ); 02582 02585 void GoTo( double x, double y, double a ); 02586 void GoTo( stg_pose_t pose ); 02587 02588 // localization state 02589 stg_pose_t est_pose; 02590 stg_pose_t est_pose_error; 02591 stg_pose_t est_origin; 02592 }; 02593 02594 02595 }; // end namespace stg 02596 02597 #endif
Generated on Wed Jul 30 11:36:06 2008 for Stage by
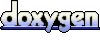