Stg::StgModel Class Reference
#include <stage.hh>
Inherits Stg::StgAncestor.
Inherited by Stg::StgModelBlinkenlight, Stg::StgModelBlobfinder, Stg::StgModelCamera, Stg::StgModelFiducial, Stg::StgModelLaser, Stg::StgModelPosition, and Stg::StgModelRanger.
Detailed Description
StgModel class
Public Member Functions | |
void | AddBlinkenlight (stg_blinkenlight_t *b) |
void | AddBlock (stg_point_t *pts, size_t pt_count, stg_meters_t zmin, stg_meters_t zmax, stg_color_t color, bool inherit_color) |
void | AddBlockRect (double x, double y, double width, double height) |
void | AddCallback (void *address, stg_model_callback_t cb, void *user) |
void | AddFlag (StgFlag *flag) |
void | AddLoadCallback (stg_model_callback_t cb, void *user) |
void | AddSaveCallback (stg_model_callback_t cb, void *user) |
void | AddShutdownCallback (stg_model_callback_t cb, void *user) |
void | AddStartupCallback (stg_model_callback_t cb, void *user) |
void | AddToPose (double dx, double dy, double dz, double da) |
void | AddToPose (stg_pose_t pose) |
void | AddUpdateCallback (stg_model_callback_t cb, void *user) |
void | CallCallbacks (void *address) |
void | ClearBlinkenlights () |
void | ClearBlocks () |
bool | DataIsFresh () |
void | Disable () |
void | Enable () |
int | FiducialKey () |
int | FiducialReturn () |
stg_color_t | GetColor () |
int | GetFlagCount () |
stg_geom_t | GetGeom () |
stg_pose_t | GetGlobalPose () |
stg_velocity_t | GetGlobalVelocity () |
stg_laser_return_t | GetLaserReturn () |
StgModel * | GetModel (const char *name) |
stg_pose_t | GetPose () |
void * | GetProperty (char *key) |
int | GetRangerReturn () |
StgModel * | GetUnsubscribedModelOfType (stg_model_type_t type) |
stg_velocity_t | GetVelocity () |
StgWorld * | GetWorld () |
void | GlobalToLocal (stg_pose_t *pose) |
int | GuiMask () |
void | Init () |
bool | IsAntecedent (StgModel *testmod) |
bool | IsDescendent (StgModel *testmod) |
bool | IsRelated (StgModel *mod2) |
virtual void | Load () |
void | Load (Worldfile *wf, int wf_entity) |
void | LoadControllerModule (char *lib) |
stg_point3_t | LocalToGlobal (stg_point3_t local) |
stg_pose_t | LocalToGlobal (stg_pose_t pose) |
void | NeedRedraw () |
bool | ObstacleReturn () |
StgModel * | Parent () |
void | PlaceInFreeSpace (stg_meters_t xmin, stg_meters_t xmax, stg_meters_t ymin, stg_meters_t ymax) |
StgFlag * | PopFlag () |
virtual void | Print (char *prefix) |
virtual const char * | PrintWithPose () |
void | PushFlag (StgFlag *flag) |
int | RemoveCallback (void *member, stg_model_callback_t callback) |
void | RemoveFlag (StgFlag *flag) |
void | RemoveLoadCallback (stg_model_callback_t cb) |
void | RemoveSaveCallback (stg_model_callback_t cb) |
void | RemoveShutdownCallback (stg_model_callback_t cb) |
void | RemoveStartupCallback (stg_model_callback_t cb) |
void | RemoveUpdateCallback (stg_model_callback_t cb) |
StgModel * | Root () |
return the root model of the tree containing this model | |
virtual void | Save () |
void | Say (const char *str) |
void | SetBlobReturn (int val) |
void | SetBoundary (int val) |
void | SetColor (stg_color_t col) |
void | SetFiducialKey (int key) |
void | SetFiducialReturn (int fid) |
void | SetGeom (stg_geom_t src) |
void | SetGlobalPose (stg_pose_t gpose) |
void | SetGlobalVelocity (stg_velocity_t gvel) |
void | SetGripperReturn (int val) |
void | SetGuiGrid (int val) |
void | SetGuiMask (int val) |
void | SetGuiNose (int val) |
void | SetGuiOutline (int val) |
void | SetLaserReturn (stg_laser_return_t val) |
void | SetMapResolution (stg_meters_t res) |
void | SetMass (stg_kg_t mass) |
void | SetObstacleReturn (int val) |
int | SetParent (StgModel *newparent) |
void | SetPose (stg_pose_t pose) |
int | SetProperty (char *key, void *data) |
Set a named property of a Stage model. | |
void | SetRangerReturn (int val) |
void | SetStall (stg_bool_t stall) |
void | SetVelocity (stg_velocity_t vel) |
void | SetWatts (stg_watts_t watts) |
void | SetWorldfile (Worldfile *wf, int wf_entity) |
bool | Stalled () |
StgModel (StgWorld *world, StgModel *parent, stg_model_type_t type=MODEL_TYPE_PLAIN) | |
void | Subscribe () |
void | UnsetProperty (char *key) |
void | Unsubscribe () |
Static Public Member Functions | |
static StgModel * | LookupId (uint32_t id) |
Protected Member Functions | |
virtual void | DataVisualize (StgCamera *cam) |
void | DataVisualizeTree (StgCamera *cam) |
void | DrawBlinkenlights () |
virtual void | DrawBlocks () |
void | DrawBlocksTree () |
void | DrawFlagList () |
void | DrawGrid () |
void | DrawImage (uint32_t texture_id, Stg::StgCamera *cam, float alpha) |
Draw the image stored in texture_id above the model. | |
void | DrawOrigin () |
void | DrawOriginTree () |
virtual void | DrawPicker () |
virtual void | DrawSelected (void) |
virtual void | DrawStatus (StgCamera *cam) |
void | DrawStatusTree (StgCamera *cam) |
void | DrawTrailArrows () |
void | DrawTrailBlocks () |
void | DrawTrailFootprint () |
void | GPoseDirtyTree () |
void | Map () |
void | MapWithChildren () |
virtual void | PopColor () |
void | PopCoords () |
void | PopPose () |
virtual void | PushColor (double r, double g, double b, double a) |
virtual void | PushColor (stg_color_t col) |
void | PushLocalCoords () |
void | PushMyPose () |
void | Raytrace (stg_radians_t bearing, stg_meters_t range, stg_radians_t fov, stg_block_match_func_t func, const void *arg, stg_raytrace_sample_t *samples, uint32_t sample_count, bool ztest=true) |
void | Raytrace (stg_radians_t bearing, stg_meters_t range, stg_block_match_func_t func, const void *arg, stg_raytrace_sample_t *sample, bool ztest=true) |
void | Raytrace (stg_pose_t pose, stg_meters_t range, stg_radians_t fov, stg_block_match_func_t func, const void *arg, stg_raytrace_sample_t *samples, uint32_t sample_count, bool ztest=true) |
void | Raytrace (stg_pose_t pose, stg_meters_t range, stg_block_match_func_t func, const void *arg, stg_raytrace_sample_t *sample, bool ztest=true) |
void | registerOption (Option *opt) |
Register an Option for pickup by the GUI. | |
void | ShiftPose (stg_pose_t *pose) |
void | ShiftToTop () |
virtual void | Shutdown () |
virtual void | Startup () |
StgModel * | TestCollision (stg_meters_t *hitx, stg_meters_t *hity) |
StgModel * | TestCollision (stg_pose_t pose, stg_meters_t *hitx, stg_meters_t *hity) |
int | TreeToPtrArray (GPtrArray *array) |
void | UnMap () |
void | UnMapWithChildren () |
virtual void | Update () |
void | UpdateIfDue () |
virtual void | UpdatePose () |
Static Protected Member Functions | |
static void | DrawBlocks (gpointer dummykey, StgModel *mod, void *arg) |
Protected Attributes | |
GPtrArray * | blinkenlights |
int | blob_return |
GList * | blocks |
int | blocks_dl |
int | boundary |
GHashTable * | callbacks |
stg_color_t | color |
bool | data_fresh |
stg_bool_t | disabled |
int | fiducial_key |
int | fiducial_return |
GList * | flag_list |
stg_geom_t | geom |
stg_pose_t | global_pose |
bool | gpose_dirty |
int | gripper_return |
int | gui_grid |
int | gui_mask |
int | gui_nose |
int | gui_outline |
ctrlinit_t * | initfunc |
stg_usec_t | interval |
stg_laser_return_t | laser_return |
stg_usec_t | last_update |
char | load_hook |
stg_meters_t | map_resolution |
stg_kg_t | mass |
int | obstacle_return |
bool | on_velocity_list |
StgModel * | parent |
stg_pose_t | pose |
GData * | props |
int | ranger_return |
bool | rebuild_displaylist |
char | save_hook |
char * | say_string |
char | shutdown_hook |
stg_bool_t | stall |
char | startup_hook |
int | subs |
GArray * | trail |
stg_model_type_t | type |
char | update_hook |
stg_velocity_t | velocity |
stg_watts_t | watts |
Worldfile * | wf |
int | wf_entity |
StgWorld * | world |
Static Protected Attributes | |
static const char * | typestr |
Member Function Documentation
void StgModel::AddToPose | ( | double | dx, | |
double | dy, | |||
double | dz, | |||
double | da | |||
) |
add values to a model's pose in its parent's coordinate system
void StgModel::AddToPose | ( | stg_pose_t | pose | ) |
add values to a model's pose in its parent's coordinate system
void StgModel::ClearBlocks | ( | ) |
remove all blocks from this model, freeing their memory
stg_geom_t Stg::StgModel::GetGeom | ( | ) | [inline] |
Get a model's geometry - it's size and local pose (offset from origin in local coords)
stg_pose_t StgModel::GetGlobalPose | ( | ) | [virtual] |
get the pose of a model in the global CS
Reimplemented from Stg::StgAncestor.
stg_velocity_t StgModel::GetGlobalVelocity | ( | ) |
get the velocity of a model in the global CS
stg_pose_t Stg::StgModel::GetPose | ( | ) | [inline] |
Get the pose of a model in its parent's coordinate system
void * StgModel::GetProperty | ( | char * | key | ) |
named-property interface
StgModel * StgModel::GetUnsubscribedModelOfType | ( | stg_model_type_t | type | ) |
returns the first descendent of this model that is unsubscribed and has the type indicated by the string
stg_velocity_t Stg::StgModel::GetVelocity | ( | ) | [inline] |
Get a model's velocity (in its local reference frame)
void StgModel::GlobalToLocal | ( | stg_pose_t * | pose | ) |
Convert a pose in the world coordinate system into a model's local coordinate system. Overwrites [pose] with the new coordinate.
void StgModel::GPoseDirtyTree | ( | ) | [protected] |
Causes this model and its children to recompute their global position instead of using a cached pose in StgModel::GetGlobalPose()..
void StgModel::Init | ( | ) |
Should be called after all models are loaded, to do any last-minute setup
void StgModel::Load | ( | ) | [virtual] |
configure a model by reading from the current world file
Reimplemented in Stg::StgModelBlobfinder, Stg::StgModelLaser, Stg::StgModelFiducial, Stg::StgModelRanger, Stg::StgModelCamera, and Stg::StgModelPosition.
void Stg::StgModel::Load | ( | Worldfile * | wf, | |
int | wf_entity | |||
) | [inline] |
stg_point3_t StgModel::LocalToGlobal | ( | stg_point3_t | local | ) |
Return the 3d point in world coordinates of a 3d point specified in the model's local coordinate system
stg_pose_t StgModel::LocalToGlobal | ( | stg_pose_t | pose | ) |
Return the global pose (i.e. pose in world coordinates) of a pose specified in the model's local coordinate system
static StgModel* Stg::StgModel::LookupId | ( | uint32_t | id | ) | [inline, static] |
Look up a model pointer by a unique model ID
void Stg::StgModel::Raytrace | ( | stg_pose_t | pose, | |
stg_meters_t | range, | |||
stg_radians_t | fov, | |||
stg_block_match_func_t | func, | |||
const void * | arg, | |||
stg_raytrace_sample_t * | samples, | |||
uint32_t | sample_count, | |||
bool | ztest = true | |||
) | [protected] |
raytraces multiple rays around the point and heading identified by pose, in local coords
void StgModel::Raytrace | ( | stg_pose_t | pose, | |
stg_meters_t | range, | |||
stg_block_match_func_t | func, | |||
const void * | arg, | |||
stg_raytrace_sample_t * | sample, | |||
bool | ztest = true | |||
) | [protected] |
raytraces a single ray from the point and heading identified by pose, in local coords
void StgModel::Save | ( | ) | [virtual] |
save the state of the model to the current world file
void StgModel::SetFiducialKey | ( | int | key | ) |
set a model's fiducial key: only fiducial finders with a matching key can detect this model as a fiducial.
void StgModel::SetFiducialReturn | ( | int | fid | ) |
set a model's geometry (size and center offsets)
void StgModel::SetGeom | ( | stg_geom_t | src | ) |
set a model's geometry (size and center offsets)
void StgModel::SetGlobalPose | ( | stg_pose_t | gpose | ) |
set the pose of model in global coordinates
int StgModel::SetParent | ( | StgModel * | newparent | ) |
Change a model's parent - experimental
void StgModel::SetPose | ( | stg_pose_t | pose | ) |
set a model's pose in its parent's coordinate system
int StgModel::SetProperty | ( | char * | key, | |
void * | data | |||
) |
Set a named property of a Stage model.
Set a property of a Stage model.
This function can set both predefined and user-defined properties of a model. Predefined properties are intrinsic to every model, such as pose and color. Every supported predefined properties has its identifying string defined as a preprocessor macro in stage.h. Users should use the macro instead of a hard-coded string, so that the compiler can help you to avoid mis-naming properties.
User-defined properties allow the user to attach arbitrary data pointers to a model. User-defined property data is not copied, so the original pointer must remain valid. User-defined property names are simple strings. Names beginning with an underscore ('_') are reserved for internal libstage use: users should not use names beginning with underscore (at risk of causing very weird behaviour).
Any callbacks registered for the named property will be called.
Returns 0 on success, or a positive error code on failure.
CAUTION* The caller is responsible for making sure the pointer points to data of the correct type for the property, so use carefully. Check the docs or the equivalent stg_model_set_<property>() function definition to see the type of data required for each property.
void StgModel::SetVelocity | ( | stg_velocity_t | vel | ) |
set a model's velocity in its parent's coordinate system
void Stg::StgModel::SetWorldfile | ( | Worldfile * | wf, | |
int | wf_entity | |||
) | [inline] |
Set the worldfile and worldfile entity ID
bool Stg::StgModel::Stalled | ( | ) | [inline] |
Returns the value of the model's stall boolean, which is true iff the model has crashed into another model
void StgModel::Subscribe | ( | ) |
subscribe to a model's data
StgModel * StgModel::TestCollision | ( | stg_meters_t * | hitx, | |
stg_meters_t * | hity | |||
) | [protected] |
Check to see if the current pose is in a collision with obstacles. Returns a pointer to the first entity we are in collision with, and stores the location of the hit in hitx,hity (if non-null) Returns NULL if no collision.
StgModel * StgModel::TestCollision | ( | stg_pose_t | pose, | |
stg_meters_t * | hitx, | |||
stg_meters_t * | hity | |||
) | [protected] |
Check to see if the given change in pose will yield a collision with obstacles. Returns a pointer to the first entity we are in collision with, and stores the location of the hit in hitx,hity (if non-null) Returns NULL if no collision.
void StgModel::Unsubscribe | ( | ) |
unsubscribe from a model's data
Member Data Documentation
int Stg::StgModel::blocks_dl [protected] |
OpenGL display list identifier
GHashTable* Stg::StgModel::callbacks [protected] |
callback functions can be attached to any field in this structure. When the internal function model_change(<mod>,<field>) is called, the callback is triggered
bool Stg::StgModel::data_fresh [protected] |
this can be set to indicate that the model has
GData* Stg::StgModel::props [protected] |
GData datalist can contain arbitrary named data items. Can be used by derived model types to store properties, and for user code to associate arbitrary items with a model.
char* Stg::StgModel::say_string [protected] |
if non-null, this string is displayed in the GUI
stg_model_type_t Stg::StgModel::type [protected] |
Compile the display list for this model
StgWorld* Stg::StgModel::world [protected] |
new data that may be of interest to users. This allows polling the model instead of adding a data callback.
The documentation for this class was generated from the following files:
- stage.hh
- model.cc
- model_callbacks.cc
- model_load.cc
- model_props.cc
Generated on Wed Jul 30 11:36:06 2008 for Stage by
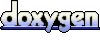