wbr914 Class Reference
Inheritance diagram for wbr914:
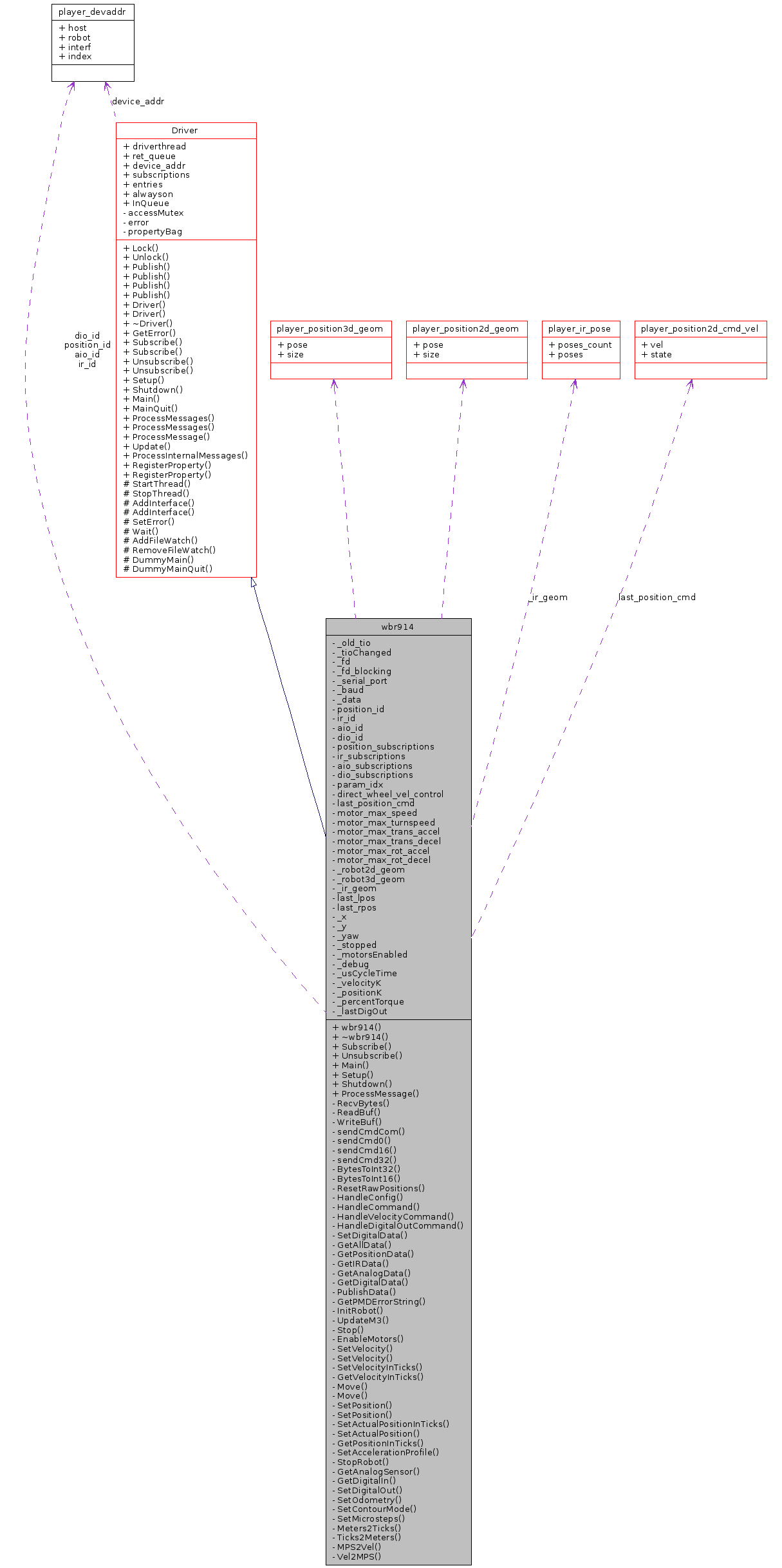
Detailed Description
Definition at line 137 of file wbr914.h.
Public Member Functions | |
wbr914 (ConfigFile *cf, int section) | |
virtual | ~wbr914 () |
Clean up any resources. | |
virtual int | Subscribe (player_devaddr_t id) |
Subscribe to this driver. | |
virtual int | Unsubscribe (player_devaddr_t id) |
Unsubscribe from this driver. | |
virtual void | Main () |
Main method for driver thread. | |
virtual int | Setup () |
Initialize the driver. | |
virtual int | Shutdown () |
Finalize the driver. | |
virtual int | ProcessMessage (QueuePointer &resp_queue, player_msghdr *hdr, void *data) |
Message handler. | |
Private Member Functions | |
bool | RecvBytes (unsigned char *s, int len) |
int | ReadBuf (unsigned char *s, size_t len) |
int | WriteBuf (unsigned char *s, size_t len) |
int | sendCmdCom (unsigned char address, unsigned char c, int cmd_num, unsigned char *arg, int ret_num, unsigned char *ret) |
int | sendCmd0 (unsigned char address, unsigned char c, int ret_num, unsigned char *ret) |
int | sendCmd16 (unsigned char address, unsigned char c, int16_t arg, int ret_num, unsigned char *ret) |
int | sendCmd32 (unsigned char address, unsigned char c, int32_t arg, int ret_num, unsigned char *ret) |
int32_t | BytesToInt32 (unsigned char *ptr) |
int16_t | BytesToInt16 (unsigned char *ptr) |
int | ResetRawPositions () |
int | HandleConfig (QueuePointer &resp_queue, player_msghdr *hdr, void *data) |
int | HandleCommand (player_msghdr *hdr, void *data) |
void | HandleVelocityCommand (player_position2d_cmd_vel_t *cmd) |
void | HandleDigitalOutCommand (player_dio_data_t *doutCmd) |
void | SetDigitalData (player_dio_data_t *d) |
void | GetAllData (void) |
void | GetPositionData (player_position2d_data_t *d) |
void | GetIRData (player_ir_data_t *d) |
void | GetAnalogData (player_aio_data_t *d) |
void | GetDigitalData (player_dio_data_t *d) |
void | PublishData (void) |
const char * | GetPMDErrorString (int rc) |
int | InitRobot () |
void | UpdateM3 () |
void | Stop (int StopMode) |
bool | EnableMotors (bool enable) |
void | SetVelocity (uint8_t chan, float mps) |
void | SetVelocity (float mpsL, float mpsR) |
void | SetVelocityInTicks (int32_t left, int32_t right) |
void | GetVelocityInTicks (int32_t *left, int32_t *right) |
void | Move (uint8_t chan, float meters) |
void | Move (float metersL, float metersR) |
void | SetPosition (uint8_t chan, float meters) |
void | SetPosition (float metersL, float metersR) |
void | SetActualPositionInTicks (int32_t left, int32_t right) |
void | SetActualPosition (float left, float right) |
void | GetPositionInTicks (int32_t *left, int32_t *right) |
void | SetAccelerationProfile () |
void | StopRobot () |
int | GetAnalogSensor (int s, short *val) |
void | GetDigitalIn (unsigned short *digIn) |
void | SetDigitalOut (unsigned short digOut) |
void | SetOdometry (player_position2d_set_odom_req_t *od) |
void | SetContourMode (ProfileMode_t prof) |
void | SetMicrosteps () |
int32_t | Meters2Ticks (float meters) |
float | Ticks2Meters (int32_t ticks) |
int32_t | MPS2Vel (float mps) |
float | Vel2MPS (int32_t vel) |
Private Attributes | |
termios | _old_tio |
bool | _tioChanged |
int | _fd |
bool | _fd_blocking |
const char * | _serial_port |
int | _baud |
player_data_t | _data |
player_devaddr_t | position_id |
player_devaddr_t | ir_id |
player_devaddr_t | aio_id |
player_devaddr_t | dio_id |
int | position_subscriptions |
int | ir_subscriptions |
int | aio_subscriptions |
int | dio_subscriptions |
int | param_idx |
int | direct_wheel_vel_control |
player_position2d_cmd_vel_t | last_position_cmd |
int | motor_max_speed |
int | motor_max_turnspeed |
short | motor_max_trans_accel |
short | motor_max_trans_decel |
short | motor_max_rot_accel |
short | motor_max_rot_decel |
player_position2d_geom_t | _robot2d_geom |
player_position3d_geom_t | _robot3d_geom |
player_ir_pose_t | _ir_geom |
int32_t | last_lpos |
int32_t | last_rpos |
double | _x |
double | _y |
double | _yaw |
bool | _stopped |
bool | _motorsEnabled |
int | _debug |
int | _usCycleTime |
double | _velocityK |
double | _positionK |
int | _percentTorque |
uint16_t | _lastDigOut |
Member Function Documentation
int wbr914::Subscribe | ( | player_devaddr_t | id | ) | [virtual] |
Subscribe to this driver.
The Subscribe() and Unsubscribe() methods are used to control subscriptions to the driver; a driver MAY override them, but usually won't.
- Parameters:
-
addr Address of the device to subscribe to (the driver may have more than one interface).
- Returns:
- Returns 0 on success.
Reimplemented from Driver.
Definition at line 517 of file wbr914.cc.
References aio_subscriptions, dio_subscriptions, ir_subscriptions, Device::MatchDeviceAddress(), position_subscriptions, and Driver::Subscribe().
Here is the call graph for this function:

int wbr914::Unsubscribe | ( | player_devaddr_t | id | ) | [virtual] |
Unsubscribe from this driver.
The Subscribe() and Unsubscribe() methods are used to control subscriptions to the driver; a driver MAY override them, but usually won't.
- Parameters:
-
addr Address of the device to unsubscribe from (the driver may have more than one interface).
- Returns:
- Returns 0 on success.
Reimplemented from Driver.
Definition at line 546 of file wbr914.cc.
References aio_subscriptions, dio_subscriptions, ir_subscriptions, Device::MatchDeviceAddress(), position_subscriptions, and Driver::Unsubscribe().
Here is the call graph for this function:

void wbr914::Main | ( | ) | [virtual] |
Main method for driver thread.
drivers have their own thread of execution, created using StartThread(); this is the entry point for the driver thread, and must be overloaded by all threaded drivers.
Reimplemented from Driver.
Definition at line 628 of file wbr914.cc.
References _debug, EnableMotors(), GetAllData(), PLAYER_MSG0, position_subscriptions, Driver::ProcessMessages(), PublishData(), ResetRawPositions(), and Driver::Unlock().
Here is the call graph for this function:
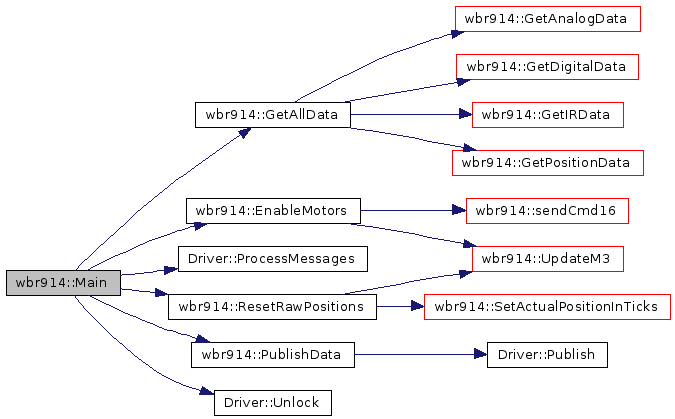
int wbr914::Setup | ( | ) | [virtual] |
Initialize the driver.
This function is called with the first client subscribes; it MUST be implemented by the driver.
- Returns:
- Returns 0 on success.
Implements Driver.
Definition at line 322 of file wbr914.cc.
References _baud, _debug, _fd, _fd_blocking, _old_tio, _serial_port, _tioChanged, _usCycleTime, _velocityK, BytesToInt16(), InitRobot(), PLAYER_MSG0, ResetRawPositions(), sendCmd0(), sendCmd16(), SetAccelerationProfile(), SetMicrosteps(), and UpdateM3().
Here is the call graph for this function:
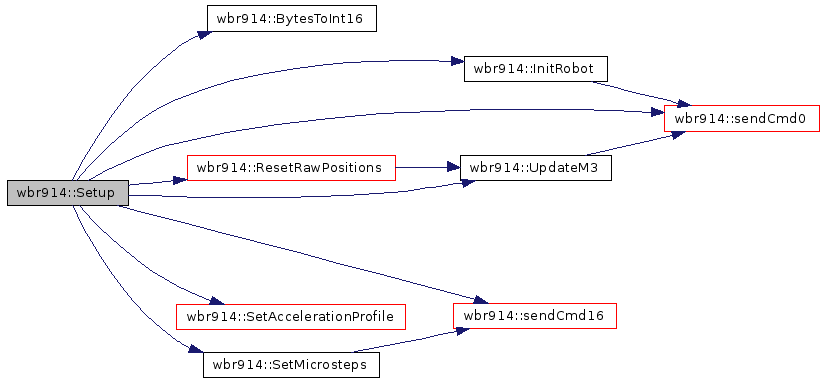
int wbr914::Shutdown | ( | ) | [virtual] |
Finalize the driver.
This function is called with the last client unsubscribes; it MUST be implemented by the driver.
- Returns:
- Returns 0 on success.
Implements Driver.
Definition at line 494 of file wbr914.cc.
References _fd, EnableMotors(), StopRobot(), and Driver::StopThread().
Referenced by ~wbr914().
Here is the call graph for this function:

int wbr914::ProcessMessage | ( | QueuePointer & | resp_queue, | |
player_msghdr * | hdr, | |||
void * | data | |||
) | [virtual] |
Message handler.
This function is called once for each message in the incoming queue. Reimplement it to provide message handling. Return 0 if you handled the message and -1 otherwise
- Parameters:
-
resp_queue The queue to which any response should go. hdr The message header data The message body
Reimplemented from Driver.
Definition at line 684 of file wbr914.cc.
References PLAYER_MSGTYPE_CMD, PLAYER_MSGTYPE_REQ, and player_msghdr::type.
The documentation for this class was generated from the following files: