Message Class Reference
#include <message.h>
Collaboration diagram for Message:
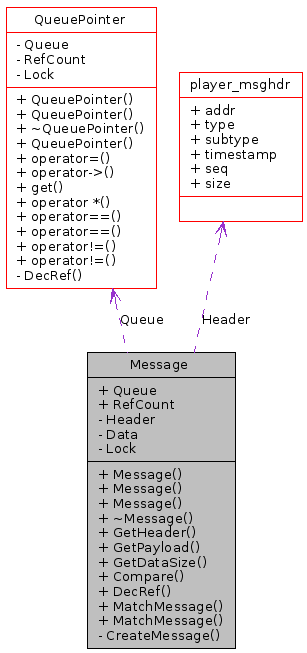
Detailed Description
Reference-counted message objects.All Player messages are transferred between drivers as pointers to Message objects. These objects are reference-counted so that messages can be delivered to multiple recipients with minimal memory overhead.
Messages are not usually manipulated directly in driver code. The details of allocating, filling, parsing, and deleting Message objects are handled by the Driver and MessageQueue classes.
The only method of interest to driver authors is the helper MatchMessage(), which can be used in a Driver::ProcessMessage method to determine if a message header matches a given signature.
Definition at line 120 of file message.h.
Public Member Functions | |
Message (const struct player_msghdr &Header, void *data, bool copy=true) | |
Create a new message. | |
Message (const struct player_msghdr &Header, void *data, QueuePointer &_queue, bool copy=true) | |
Create a new message with an associated queue. | |
Message (const Message &rhs) | |
Copy pointers from existing message and increment refcount. | |
~Message () | |
Destroy message, dec ref counts and delete data if ref count == 0. | |
player_msghdr_t * | GetHeader () |
Get pointer to header. | |
void * | GetPayload () |
Get pointer to payload. | |
unsigned int | GetDataSize () |
Size of message data. | |
bool | Compare (Message &other) |
Compare type, subtype, device, and device_index. | |
void | DecRef () |
Decrement ref count. | |
Static Public Member Functions | |
static bool | MatchMessage (player_msghdr_t *hdr, int type, int subtype, player_devaddr_t addr) |
Helper for message processing. | |
static bool | MatchMessage (player_msghdr_t *hdr, int type, int subtype) |
Helper for message processing. | |
Public Attributes | |
QueuePointer | Queue |
queue to which any response to this message should be directed | |
unsigned int * | RefCount |
Reference count. | |
Private Member Functions | |
void | CreateMessage (const struct player_msghdr &Header, void *data, bool copy=true) |
Private Attributes | |
player_msghdr_t | Header |
message header | |
uint8_t * | Data |
Pointer to the message data. | |
pthread_mutex_t * | Lock |
Used to lock access to Data. |
Constructor & Destructor Documentation
Message::Message | ( | const struct player_msghdr & | Header, | |
void * | data, | |||
bool | copy = true | |||
) |
Create a new message.
If copy is set to false then the pointer is claimed by the message, otherwise it is copied.
Message::Message | ( | const struct player_msghdr & | Header, | |
void * | data, | |||
QueuePointer & | _queue, | |||
bool | copy = true | |||
) |
Create a new message with an associated queue.
If copy is set to false then the pointer is claimed by the message, otherwise it is copied.
Member Function Documentation
static bool Message::MatchMessage | ( | player_msghdr_t * | hdr, | |
int | type, | |||
int | subtype, | |||
player_devaddr_t | addr | |||
) | [inline, static] |
Helper for message processing.
Returns true if hdr
matches the supplied type
, subtype
, and addr
. Set type and/or subtype to -1 for don't care.
Definition at line 147 of file message.h.
References player_msghdr::addr, player_devaddr::host, player_devaddr::index, player_devaddr::interf, player_devaddr::robot, player_msghdr::subtype, and player_msghdr::type.
Referenced by P2OS::HandleActArrayCommand(), P2OS::HandleArmGripperCommand(), wbr914::HandleCommand(), P2OS::HandleCommand(), Erratic::HandleCommand(), wbr914::HandleConfig(), P2OS::HandleConfig(), Erratic::HandleConfig(), P2OS::HandleGripperCommand(), P2OS::HandleLiftCommand(), P2OS::HandleLimbCommand(), ToRanger::ProcessMessage(), SphereDriver::ProcessMessage(), SegwayRMP::ProcessMessage(), RFLEX::ProcessMessage(), MapTransform::ProcessMessage(), LaserTransform::ProcessMessage(), Khepera::ProcessMessage(), ImageBase::ProcessMessage(), GarciaDriver::ProcessMessage(), ER::ProcessMessage(), ClodBuster::ProcessMessage(), AMCLOdom::ProcessMessage(), AMCLLaser::ProcessMessage(), AdaptiveMCL::ProcessMessage(), and Alsa::ProcessMessage().
static bool Message::MatchMessage | ( | player_msghdr_t * | hdr, | |
int | type, | |||
int | subtype | |||
) | [inline, static] |
Helper for message processing.
This version matches for any message destination (i.e. all a drivers reigstered interfaces) Returns true if hdr
matches the supplied type
, subtype
. Set type and/or subtype to -1 for don't care.
Definition at line 165 of file message.h.
References player_msghdr::subtype, and player_msghdr::type.
The documentation for this class was generated from the following file: