It controls the e-puck velocity and odometry. More...
#include <epuckPosition2d.hpp>
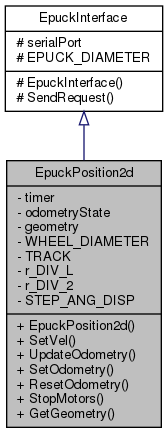
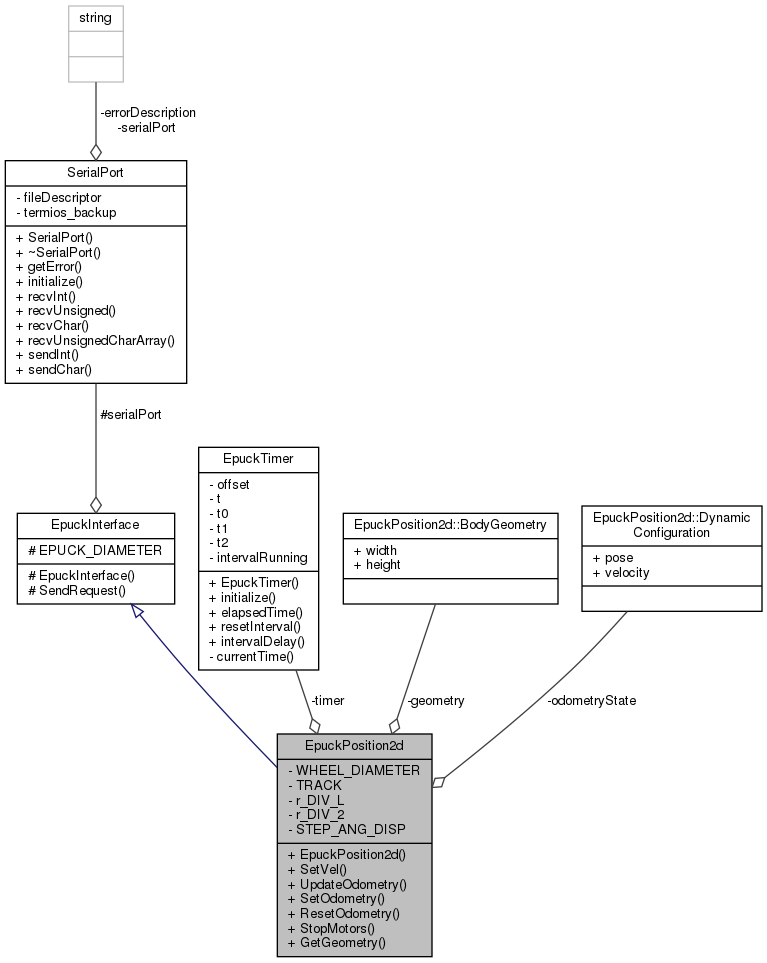
Detailed Description
It controls the e-puck velocity and odometry.
- Date
- August 2008
Classes | |
struct | BodyGeometry |
Struct which represents the geometry of e-puck body. More... | |
struct | DynamicConfiguration |
Struct which represents the pose and velocity of e-puck. More... | |
Public Member Functions | |
EpuckPosition2d (const SerialPort *const serialPort) | |
The EpuckPosition2d class constructor. More... | |
void | SetVel (float px, float pa) const |
Set linear and angular velocities to e-puck. More... | |
DynamicConfiguration | UpdateOdometry () |
Estimate the current pose and velocities. More... | |
void | SetOdometry (Triple odometry) |
Set the current pose estimated by odometry. More... | |
void | ResetOdometry () |
Set the current pose estimated by odometry - (x,y,theta) - to (0,0,0). | |
void | StopMotors () const |
Stop the e-puck motors. More... | |
BodyGeometry | GetGeometry () const |
Give the e-puck geometry. More... | |
Private Attributes | |
EpuckTimer | timer |
DynamicConfiguration | odometryState |
BodyGeometry | geometry |
Additional Inherited Members | |
![]() | |
enum | Request { CONFIG_CAMERA = 0x02, SET_VEL = 0x13, GET_STEPS = 0x14, STOP_MOTORS = 0x15, GET_IR_PROX = 0x16, GET_CAMERA_IMG = 0x17, SET_LED_POWER = 0x18 } |
Request codes acceptable by e-puck. More... | |
![]() | |
EpuckInterface (const SerialPort *const serialPort) | |
The EpuckInterface class constructor. More... | |
void | SendRequest (Request request) const |
![]() | |
const SerialPort *const | serialPort |
A SerialPort class instance shared among the device interfaces. More... | |
![]() | |
static const float | EPUCK_DIAMETER = 0.07 |
Diameter of e-puck body [m]. | |
Constructor & Destructor Documentation
◆ EpuckPosition2d()
EpuckPosition2d::EpuckPosition2d | ( | const SerialPort *const | serialPort | ) |
The EpuckPosition2d class constructor.
- Parameters
-
serialPort Pointer for a SerialPort class already created and connected with an e-puck.
References EpuckInterface::EPUCK_DIAMETER, EpuckPosition2d::BodyGeometry::height, and EpuckPosition2d::BodyGeometry::width.
Member Function Documentation
◆ GetGeometry()
|
inline |
Give the e-puck geometry.
- Returns
- A BodyGeometry struct.
◆ SetOdometry()
void EpuckPosition2d::SetOdometry | ( | Triple | odometry | ) |
Set the current pose estimated by odometry.
Set the internal representation for e-puck current pose estimated from odometry to the given value.
- Parameters
-
odometry The value which will be set.
References EpuckInterface::GET_STEPS, EpuckPosition2d::DynamicConfiguration::pose, SerialPort::recvInt(), EpuckInterface::serialPort, EpuckInterface::Triple::theta, EpuckInterface::Triple::x, and EpuckInterface::Triple::y.

◆ SetVel()
void EpuckPosition2d::SetVel | ( | float | px, |
float | pa | ||
) | const |
Set linear and angular velocities to e-puck.
Receive the linear and agular velocities, convert to correspondent steps per seconds motors commands and send to e-puck.
- Parameters
-
px Linear velocity. [m/s] pa Angular velocity. [rad/s]
References SerialPort::recvChar(), SerialPort::sendInt(), EpuckInterface::serialPort, and EpuckInterface::SET_VEL.
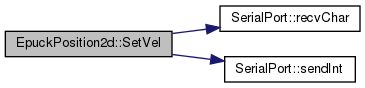
◆ StopMotors()
void EpuckPosition2d::StopMotors | ( | ) | const |
Stop the e-puck motors.
Stop the two motors and update the odometry by the steps yet non computed.
References SerialPort::recvChar(), EpuckInterface::serialPort, and EpuckInterface::STOP_MOTORS.

◆ UpdateOdometry()
EpuckPosition2d::DynamicConfiguration EpuckPosition2d::UpdateOdometry | ( | ) |
Estimate the current pose and velocities.
Receive from e-puck the motors steps made since the last call at this function, and estimate the new current pose and velocities.
- Returns
- A DynamicConfiguration struct.
References EpuckInterface::GET_STEPS, EpuckTimer::intervalDelay(), EpuckPosition2d::DynamicConfiguration::pose, SerialPort::recvInt(), EpuckTimer::resetInterval(), EpuckInterface::serialPort, EpuckInterface::Triple::theta, EpuckPosition2d::DynamicConfiguration::velocity, EpuckInterface::Triple::x, and EpuckInterface::Triple::y.
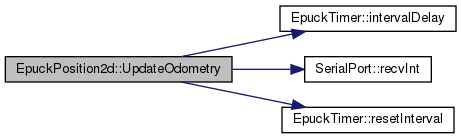
The documentation for this class was generated from the following files:
- epuckPosition2d.hpp
- epuckPosition2d.cpp