the Stereo
proxy provides access to the stereo device.
More...
#include <playerc++.h>
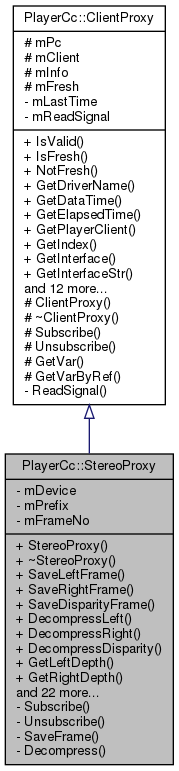
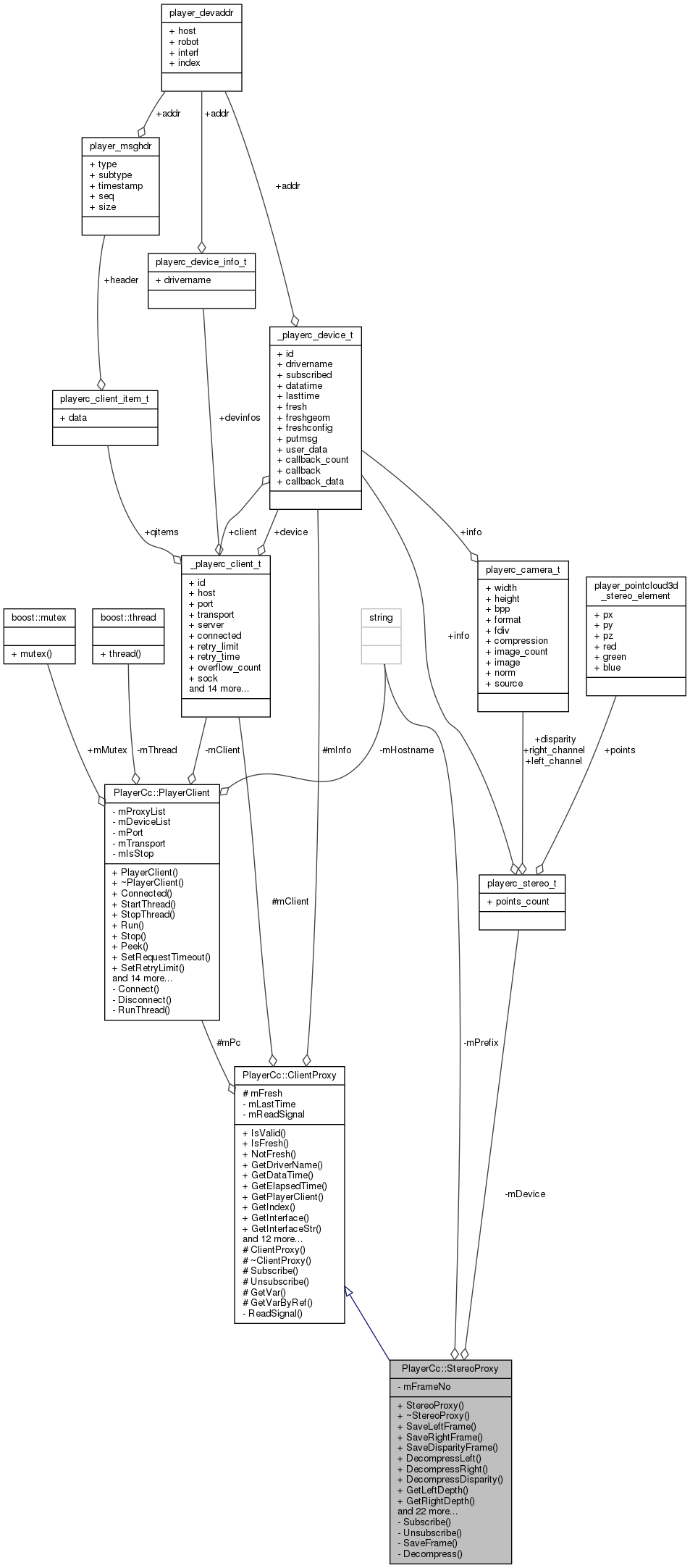
Detailed Description
the Stereo
proxy provides access to the stereo device.
Public Member Functions | |
StereoProxy (PlayerClient *aPc, uint32_t aIndex=0) | |
Constructor. | |
~StereoProxy () | |
Destructor. | |
void | SaveLeftFrame (const std::string aPrefix, uint32_t aWidth=4) |
Save the left frame. More... | |
void | SaveRightFrame (const std::string aPrefix, uint32_t aWidth=4) |
Save the right frame. More... | |
void | SaveDisparityFrame (const std::string aPrefix, uint32_t aWidth=4) |
Save the disparity frame. More... | |
void | DecompressLeft () |
decompress the left image | |
void | DecompressRight () |
decompress the right image | |
void | DecompressDisparity () |
decompress the disparity image | |
uint32_t | GetLeftDepth () const |
Left image color depth. | |
uint32_t | GetRightDepth () const |
Right image color depth. | |
uint32_t | GetDisparityDepth () const |
Disparity image color depth. | |
uint32_t | GetLeftWidth () const |
Left image width (pixels) | |
uint32_t | GetRightWidth () const |
Right image width (pixels) | |
uint32_t | GetDisparityWidth () const |
Disparity image width (pixels) | |
uint32_t | GetLeftHeight () const |
Left image height (pixels) | |
uint32_t | GetRightHeight () const |
Right image height (pixels) | |
uint32_t | GetDisparityHeight () const |
Disparity image height (pixels) | |
uint32_t | GetLeftFormat () const |
Image format Possible values include. More... | |
uint32_t | GetRightFormat () const |
Image format Possible values include. More... | |
uint32_t | GetDisparityFormat () const |
Image format Possible values include. More... | |
uint32_t | GetLeftImageSize () const |
Size of the left image (bytes) | |
uint32_t | GetRightImageSize () const |
Size of the right image (bytes) | |
uint32_t | GetDisparityImageSize () const |
Size of the disparity image (bytes) | |
void | GetLeftImage (uint8_t *aImage) const |
Left image data This function copies the image data into the data buffer aImage. More... | |
void | GetRightImage (uint8_t *aImage) const |
Right image data This function copies the image data into the data buffer aImage. More... | |
void | GetDisparityImage (uint8_t *aImage) const |
Disparity image data This function copies the image data into the data buffer aImage. More... | |
uint32_t | GetLeftCompression () const |
Get the left image's compression type Currently supported compression types are: More... | |
uint32_t | GetRightCompression () const |
Get the right image's compression type Currently supported compression types are: More... | |
uint32_t | GetDisparityCompression () const |
Get the disparity image's compression type Currently supported compression types are: More... | |
uint32_t | GetCount () const |
return the point count | |
player_pointcloud3d_stereo_element_t | GetPoint (uint32_t aIndex) const |
return a particular point value | |
player_pointcloud3d_stereo_element_t | operator[] (uint32_t aIndex) const |
This operator provides an alternate way of access the point data. More... | |
![]() | |
bool | IsValid () const |
Proxy has any information. More... | |
bool | IsFresh () const |
Check for fresh data. More... | |
void | NotFresh () |
Reset Fresh flag. More... | |
std::string | GetDriverName () const |
Get the underlying driver's name. More... | |
double | GetDataTime () const |
Returns the received timestamp of the last data sample [s]. | |
double | GetElapsedTime () const |
Returns the time between the current data time and the time of the last data sample [s]. | |
PlayerClient * | GetPlayerClient () const |
Get a pointer to the Player Client. More... | |
uint32_t | GetIndex () const |
Get device index. More... | |
uint32_t | GetInterface () const |
Get Interface Code. More... | |
std::string | GetInterfaceStr () const |
Get Interface Name. More... | |
void | SetReplaceRule (bool aReplace, int aType=-1, int aSubtype=-1) |
Set a replace rule for this proxy on the server. More... | |
int | HasCapability (uint32_t aType, uint32_t aSubtype) |
Request capabilities of device. More... | |
int | GetBoolProp (char *aProperty, bool *aValue) |
Request a boolean property. More... | |
int | SetBoolProp (char *aProperty, bool aValue) |
Set a boolean property. More... | |
int | GetIntProp (char *aProperty, int32_t *aValue) |
Request an integer property. More... | |
int | SetIntProp (char *aProperty, int32_t aValue) |
Set an integer property. More... | |
int | GetDblProp (char *aProperty, double *aValue) |
Request a double property. More... | |
int | SetDblProp (char *aProperty, double aValue) |
Set a double property. More... | |
int | GetStrProp (char *aProperty, char **aValue) |
Request a string property. More... | |
int | SetStrProp (char *aProperty, char *aValue) |
Set a string property. More... | |
template<typename T > | |
connection_t | ConnectReadSignal (T aSubscriber) |
Connect a read signal to this proxy. More... | |
void | DisconnectReadSignal (connection_t aSubscriber) |
Disconnect a signal from this proxy. More... | |
Private Member Functions | |
void | Subscribe (uint32_t aIndex) |
void | Unsubscribe () |
void | SaveFrame (const std::string aPrefix, uint32_t aWidth, playerc_camera_t aDevice, uint8_t aIndex) |
Save a frame. | |
void | Decompress (playerc_camera_t aDevice) |
Decompress an image. | |
Private Attributes | |
playerc_stereo_t * | mDevice |
libplayerc data structure | |
std::string | mPrefix |
Default image prefix. | |
uint32_t | mFrameNo [3] |
Frame counter for image saving methods. | |
Additional Inherited Members | |
![]() | |
typedef int | connection_t |
typedef boost::mutex::scoped_lock | scoped_lock_t |
typedef int | read_signal_t |
![]() | |
ClientProxy (PlayerClient *aPc, uint32_t aIndex) | |
template<typename T > | |
T | GetVar (const T &aV) const |
template<typename T > | |
void | GetVarByRef (const T aBegin, const T aEnd, T aDest) const |
![]() | |
PlayerClient * | mPc |
playerc_client_t * | mClient |
playerc_device_t * | mInfo |
bool | mFresh |
Member Function Documentation
◆ GetDisparityCompression()
|
inline |
Get the disparity image's compression type Currently supported compression types are:
References playerc_camera_t::compression.
◆ GetDisparityFormat()
|
inline |
Image format Possible values include.
- PLAYER_CAMERA_FORMAT_MONO8
- PLAYER_CAMERA_FORMAT_MONO16
- PLAYER_CAMERA_FORMAT_RGB565
- PLAYER_CAMERA_FORMAT_RGB888
References playerc_camera_t::format.
◆ GetDisparityImage()
|
inline |
Disparity image data This function copies the image data into the data buffer aImage.
The buffer should be allocated according to the width, height, and depth of the image. The size can be found by calling GetDisparityImageSize().
References playerc_camera_t::image, and playerc_camera_t::image_count.
◆ GetLeftCompression()
|
inline |
Get the left image's compression type Currently supported compression types are:
References playerc_camera_t::compression.
◆ GetLeftFormat()
|
inline |
Image format Possible values include.
- PLAYER_CAMERA_FORMAT_MONO8
- PLAYER_CAMERA_FORMAT_MONO16
- PLAYER_CAMERA_FORMAT_RGB565
- PLAYER_CAMERA_FORMAT_RGB888
References playerc_camera_t::format.
◆ GetLeftImage()
|
inline |
Left image data This function copies the image data into the data buffer aImage.
The buffer should be allocated according to the width, height, and depth of the image. The size can be found by calling GetLeftImageSize().
References playerc_camera_t::image, and playerc_camera_t::image_count.
◆ GetRightCompression()
|
inline |
Get the right image's compression type Currently supported compression types are:
References playerc_camera_t::compression.
◆ GetRightFormat()
|
inline |
Image format Possible values include.
- PLAYER_CAMERA_FORMAT_MONO8
- PLAYER_CAMERA_FORMAT_MONO16
- PLAYER_CAMERA_FORMAT_RGB565
- PLAYER_CAMERA_FORMAT_RGB888
References playerc_camera_t::format.
◆ GetRightImage()
|
inline |
Right image data This function copies the image data into the data buffer aImage.
The buffer should be allocated according to the width, height, and depth of the image. The size can be found by calling GetRightImageSize().
References playerc_camera_t::image, and playerc_camera_t::image_count.
◆ operator[]()
|
inline |
This operator provides an alternate way of access the point data.
For example, StereoProxy[0] == StereoProxy.GetPoint(0)
◆ SaveDisparityFrame()
|
inline |
Save the disparity frame.
- aPrefix is the string prefix to name the image.
- aWidth is the number of 0s to pad the image numbering with.
◆ SaveLeftFrame()
|
inline |
Save the left frame.
- aPrefix is the string prefix to name the image.
- aWidth is the number of 0s to pad the image numbering with.
◆ SaveRightFrame()
|
inline |
Save the right frame.
- aPrefix is the string prefix to name the image.
- aWidth is the number of 0s to pad the image numbering with.
The documentation for this class was generated from the following file: