The client proxy base class. More...
#include <clientproxy.h>

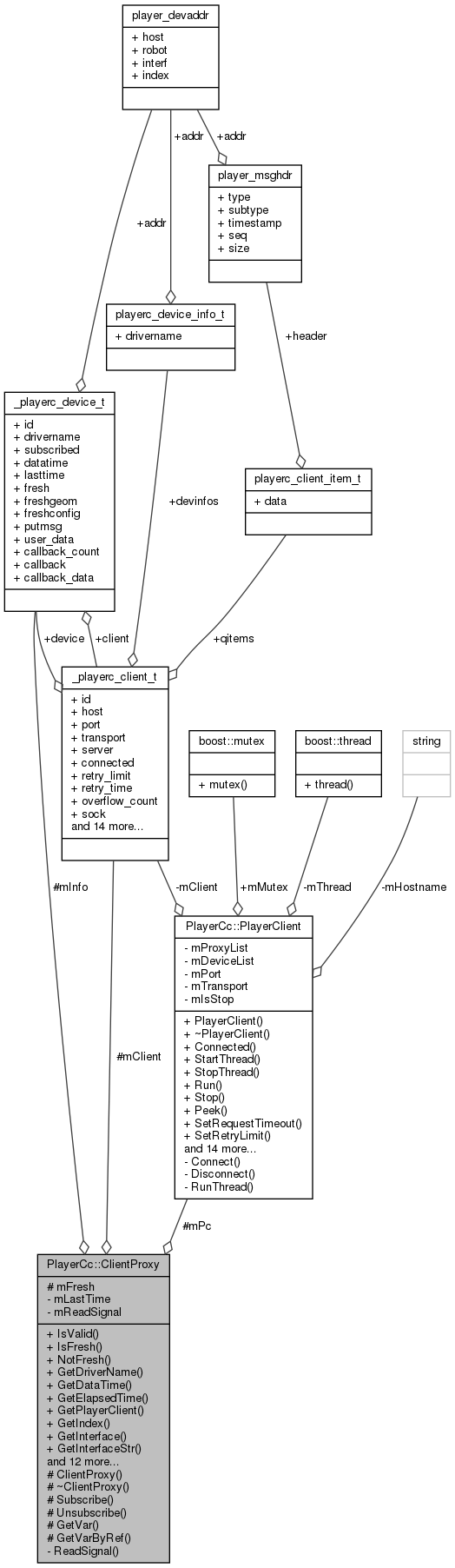
Detailed Description
The client proxy base class.
Base class for all proxy devices. Access to a device is provided by a device-specific proxy class. These classes all inherit from the ClientProxy
class which defines an interface for device proxies. As such, a few methods are common to all devices and we explain them here.
Since the ConnectReadSignal() and DisconnectReadSignal() member functions are based on the Boost signals library, they are conditionally available depending on Boost's presence in the system. See the configure script for more information.
Public Types | |
typedef int | connection_t |
typedef boost::mutex::scoped_lock | scoped_lock_t |
typedef int | read_signal_t |
Public Member Functions | |
bool | IsValid () const |
Proxy has any information. More... | |
bool | IsFresh () const |
Check for fresh data. More... | |
void | NotFresh () |
Reset Fresh flag. More... | |
std::string | GetDriverName () const |
Get the underlying driver's name. More... | |
double | GetDataTime () const |
Returns the received timestamp of the last data sample [s]. | |
double | GetElapsedTime () const |
Returns the time between the current data time and the time of the last data sample [s]. | |
PlayerClient * | GetPlayerClient () const |
Get a pointer to the Player Client. More... | |
uint32_t | GetIndex () const |
Get device index. More... | |
uint32_t | GetInterface () const |
Get Interface Code. More... | |
std::string | GetInterfaceStr () const |
Get Interface Name. More... | |
void | SetReplaceRule (bool aReplace, int aType=-1, int aSubtype=-1) |
Set a replace rule for this proxy on the server. More... | |
int | HasCapability (uint32_t aType, uint32_t aSubtype) |
Request capabilities of device. More... | |
int | GetBoolProp (char *aProperty, bool *aValue) |
Request a boolean property. More... | |
int | SetBoolProp (char *aProperty, bool aValue) |
Set a boolean property. More... | |
int | GetIntProp (char *aProperty, int32_t *aValue) |
Request an integer property. More... | |
int | SetIntProp (char *aProperty, int32_t aValue) |
Set an integer property. More... | |
int | GetDblProp (char *aProperty, double *aValue) |
Request a double property. More... | |
int | SetDblProp (char *aProperty, double aValue) |
Set a double property. More... | |
int | GetStrProp (char *aProperty, char **aValue) |
Request a string property. More... | |
int | SetStrProp (char *aProperty, char *aValue) |
Set a string property. More... | |
template<typename T > | |
connection_t | ConnectReadSignal (T aSubscriber) |
Connect a read signal to this proxy. More... | |
void | DisconnectReadSignal (connection_t aSubscriber) |
Disconnect a signal from this proxy. More... | |
Protected Member Functions | |
ClientProxy (PlayerClient *aPc, uint32_t aIndex) | |
virtual void | Subscribe (uint32_t) |
virtual void | Unsubscribe () |
template<typename T > | |
T | GetVar (const T &aV) const |
template<typename T > | |
void | GetVarByRef (const T aBegin, const T aEnd, T aDest) const |
Protected Attributes | |
PlayerClient * | mPc |
playerc_client_t * | mClient |
playerc_device_t * | mInfo |
bool | mFresh |
Private Member Functions | |
void | ReadSignal () |
Private Attributes | |
double | mLastTime |
read_signal_t | mReadSignal |
Member Function Documentation
◆ ConnectReadSignal()
|
inline |
Connect a read signal to this proxy.
Connects a signal to the proxy to trigger. This functionality depends on boost::signals, and will fail if Player was not compiled against the boost signal library. For more information check out Signals & multithreading
References PlayerCc::PlayerClient::mMutex.
◆ DisconnectReadSignal()
|
inline |
Disconnect a signal from this proxy.
Disconnects a connected read signal from the proxy For more information check out Signals & multithreading
References PlayerCc::PlayerClient::mMutex.
◆ GetBoolProp()
int PlayerCc::ClientProxy::GetBoolProp | ( | char * | aProperty, |
bool * | aValue | ||
) |
Request a boolean property.
Request a boolean property from the driver. If the has the property requested, the current value of the property will be returned.
- Parameters
-
[in] aProperty String containing the desired property name [out] aValue Value of the requested property, if available
- Returns
- 0 If property request was successful, nonzero otherwise
◆ GetDblProp()
int PlayerCc::ClientProxy::GetDblProp | ( | char * | aProperty, |
double * | aValue | ||
) |
Request a double property.
Request a double property from the driver. If the has the property requested, the current value of the property will be returned.
- Parameters
-
[in] aProperty String containing the desired property name [out] aValue Value of the requested property, if available
- Returns
- 0 If property request was successful, nonzero otherwise.
◆ GetDriverName()
|
inline |
Get the underlying driver's name.
Get the name of the driver that the ClientProxy is connected to.
- Returns
- Driver name
- Todo:
- GetDriverName isn't guarded by locks yet
References _playerc_device_t::drivername.
◆ GetIndex()
|
inline |
Get device index.
Returns the device index of the interface the ClientProxy object is connected to.
- Returns
- interface's device index
References _playerc_device_t::addr, and player_devaddr::index.
◆ GetInterface()
|
inline |
Get Interface Code.
Get the interface code of the underlying proxy. See Interface codes for a list of supported interface codes.
- Returns
- Interface code
References _playerc_device_t::addr, and player_devaddr::interf.
◆ GetInterfaceStr()
|
inline |
Get Interface Name.
Get the interface type of the proxy as a string.
- Returns
- Interface name
References _playerc_device_t::addr, and player_devaddr::interf.
◆ GetIntProp()
int PlayerCc::ClientProxy::GetIntProp | ( | char * | aProperty, |
int32_t * | aValue | ||
) |
Request an integer property.
Request an integer property from the driver. If the has the property requested, the current value of the property will be returned.
- Parameters
-
[in] aProperty String containing the desired property name [out] aValue Value of the requested property, if available
- Returns
- 0 If property request was successful, nonzero otherwise.
◆ GetPlayerClient()
|
inline |
Get a pointer to the Player Client.
Returns a pointer to the PlayerClient object that this client proxy is connected through.
◆ GetStrProp()
int PlayerCc::ClientProxy::GetStrProp | ( | char * | aProperty, |
char ** | aValue | ||
) |
Request a string property.
Request a double property from the driver. If the has the property requested, the current value of the property will be returned.
- Parameters
-
[in] aProperty String containing the desired property name [out] aValue Value of the requested property, if available
- Returns
- 0 If property request was successful, nonzero otherwise.
◆ HasCapability()
int PlayerCc::ClientProxy::HasCapability | ( | uint32_t | aType, |
uint32_t | aSubtype | ||
) |
Request capabilities of device.
Send a message asking if the device supports the given message type and subtype. If it does, the return value will be 1, and 0 otherwise.
- Parameters
-
aType The capability type aSubtype The capability subtype
- Returns
- 1 if capability is supported, 0 otherwise.
◆ IsFresh()
|
inline |
Check for fresh data.
Fresh is set to true on each new read. It is up to the user to set it to false if the data has already been read, by using NotFresh() This is most useful when used in conjunction with the PlayerMultiClient
- Returns
- True if new data was read since the Fresh flag was last set false
◆ IsValid()
|
inline |
Proxy has any information.
This function can be used to see if any data has been received from the driver since the ClientProxy was created.
- Returns
- true if we have received any data from the device.
References _playerc_device_t::datatime.
◆ NotFresh()
void PlayerCc::ClientProxy::NotFresh | ( | ) |
Reset Fresh flag.
This sets the client's "Fresh" flag to false. After this is called, IsFresh() will return false until new information is available after a call to a Read() method.
◆ SetBoolProp()
int PlayerCc::ClientProxy::SetBoolProp | ( | char * | aProperty, |
bool | aValue | ||
) |
Set a boolean property.
Set a boolean property to a given value in the driver. If the property exists and can be set, it will be set to the new value.
- Parameters
-
[in] aProperty String containing the property name aValue The value to set the property to
- Returns
- 0 if property change was successful, nonzero otherwise
◆ SetDblProp()
int PlayerCc::ClientProxy::SetDblProp | ( | char * | aProperty, |
double | aValue | ||
) |
Set a double property.
Set an integer property to a given value in the driver. If the property exists and can be set, it will be set to the new value.
- Parameters
-
[in] aProperty String containing the property name aValue The value to set the property to
- Returns
- 0 if property change was successful, nonzero otherwise
◆ SetIntProp()
int PlayerCc::ClientProxy::SetIntProp | ( | char * | aProperty, |
int32_t | aValue | ||
) |
Set an integer property.
Set an integer property to a given value in the driver. If the property exists and can be set, it will be set to the new value.
- Parameters
-
[in] aProperty String containing the property name aValue The value to set the property to
- Returns
- 0 if property change was successful, nonzero otherwise
◆ SetReplaceRule()
void PlayerCc::ClientProxy::SetReplaceRule | ( | bool | aReplace, |
int | aType = -1 , |
||
int | aSubtype = -1 |
||
) |
Set a replace rule for this proxy on the server.
If a rule with the same pattern already exists, it will be replaced with the new rule (i.e., its setting to replace will be updated).
- Parameters
-
aReplace Should we replace these messages aType The type to set replace rule for (-1 for wildcard), see Message types. aSubtype Message subtype to set replace rule for (-1 for wildcard). This is dependent on the Interface specifications.
- Exceptions
-
throws PlayerError if unsuccessfull
◆ SetStrProp()
int PlayerCc::ClientProxy::SetStrProp | ( | char * | aProperty, |
char * | aValue | ||
) |
Set a string property.
Set a string property to a given value in the driver. If the property exists and can be set, it will be set to the new value.
- Parameters
-
[in] aProperty String containing the property name aValue The value to set the property to
- Returns
- 0 if property change was successful, nonzero otherwise
The documentation for this class was generated from the following file: