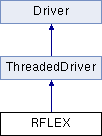
Public Member Functions | |
RFLEX (ConfigFile *cf, int section) | |
virtual void | Main () |
Main method for driver thread. | |
virtual int | Subscribe (player_devaddr_t addr) |
Subscribe to this driver. | |
virtual int | Unsubscribe (player_devaddr_t addr) |
Unsubscribe from this driver. | |
virtual void | MainQuit () |
Cleanup method for driver thread (called when main exits) | |
int | ProcessMessage (QueuePointer &resp_queue, player_msghdr *hdr, void *data) |
Message handler. | |
Static Public Attributes | |
static int | joy_control |
Private Member Functions | |
void | ResetRawPositions () |
int | initialize_robot () |
void | reset_odometry () |
void | set_odometry (float, float, float) |
void | update_everything (player_rflex_data_t *d) |
void | set_config_defaults () |
Private Attributes | |
player_devaddr_t | position_id |
player_devaddr_t | sonar_id |
player_devaddr_t | sonar_id_2 |
player_devaddr_t | ir_id |
player_devaddr_t | bumper_id |
player_devaddr_t | power_id |
player_devaddr_t | aio_id |
player_devaddr_t | dio_id |
player_position2d_cmd_vel_t | command |
int | command_type |
int | position_subscriptions |
int | sonar_subscriptions |
int | ir_subscriptions |
int | bumper_subscriptions |
int | rflex_fd |
char | rflex_serial_port [MAX_FILENAME_SIZE] |
double | m_odo_x |
double | m_odo_y |
double | rad_odo_theta |
Member Function Documentation
void RFLEX::Main | ( | ) | [virtual] |
Main method for driver thread.
drivers have their own thread of execution, created using StartThread(); this is the entry point for the driver thread, and must be overloaded by all threaded drivers.
Implements ThreadedDriver.
References player_devaddr::interf, PLAYER_AIO_DATA_STATE, PLAYER_BUMPER_DATA_STATE, PLAYER_DIO_DATA_VALUES, PLAYER_ERROR, PLAYER_IR_DATA_RANGES, PLAYER_MSG1, PLAYER_MSGTYPE_DATA, PLAYER_POSITION2D_DATA_STATE, PLAYER_POWER_DATA_STATE, PLAYER_SONAR_DATA_GEOM, PLAYER_SONAR_DATA_RANGES, player_sonar_geom::poses, player_sonar_geom::poses_count, Driver::ProcessMessages(), Driver::Publish(), player_pose3d::px, player_pose3d::py, player_pose3d::pyaw, and ThreadedDriver::Wait().
void RFLEX::MainQuit | ( | void | ) | [virtual] |
Cleanup method for driver thread (called when main exits)
Overload this method and to do additional cleanup when the driver thread exits.
Reimplemented from ThreadedDriver.
int RFLEX::ProcessMessage | ( | QueuePointer & | resp_queue, |
player_msghdr * | hdr, | ||
void * | data | ||
) | [virtual] |
Message handler.
This function is called once for each message in the incoming queue. Reimplement it to provide message handling. Return 0 if you handled the message and -1 otherwise
- Parameters:
-
resp_queue The queue to which any response should go. hdr The message header data The message body
Reimplemented from Driver.
References player_bumper_geom::bumper_def, player_bumper_geom::bumper_def_count, Message::MatchMessage(), player_pose2d::pa, PLAYER_BUMPER_REQ_GET_GEOM, PLAYER_IR_REQ_POSE, PLAYER_IR_REQ_POWER, PLAYER_MSGTYPE_CMD, PLAYER_MSGTYPE_REQ, PLAYER_MSGTYPE_RESP_ACK, PLAYER_POSITION2D_CMD_VEL, PLAYER_POSITION2D_REQ_GET_GEOM, PLAYER_POSITION2D_REQ_MOTOR_POWER, PLAYER_POSITION2D_REQ_RESET_ODOM, PLAYER_POSITION2D_REQ_SET_ODOM, PLAYER_POSITION2D_REQ_VELOCITY_MODE, PLAYER_SONAR_REQ_GET_GEOM, PLAYER_SONAR_REQ_POWER, player_position2d_set_odom_req::pose, player_sonar_geom::poses, player_sonar_geom::poses_count, Driver::Publish(), player_pose2d::px, player_pose2d::py, player_position2d_geom::size, player_bbox3d::sl, player_ir_power_req::state, player_msghdr::subtype, and player_bbox3d::sw.
int RFLEX::Subscribe | ( | player_devaddr_t | addr | ) | [virtual] |
Subscribe to this driver.
The Subscribe() and Unsubscribe() methods are used to control subscriptions to the driver; a driver MAY override them, but usually won't.
- Parameters:
-
addr Address of the device to subscribe to (the driver may have more than one interface).
- Returns:
- Returns 0 on success.
Reimplemented from Driver.
References Driver::Subscribe().
int RFLEX::Unsubscribe | ( | player_devaddr_t | addr | ) | [virtual] |
Unsubscribe from this driver.
The Subscribe() and Unsubscribe() methods are used to control subscriptions to the driver; a driver MAY override them, but usually won't.
- Parameters:
-
addr Address of the device to unsubscribe from (the driver may have more than one interface).
- Returns:
- Returns 0 on success.
Reimplemented from Driver.
References Driver::Unsubscribe().
The documentation for this class was generated from the following files:
- rflex.h
- rflex.cc