Stg::World Class Reference
World class More...
#include <stage.hh>
Inherits Stg::Ancestor.
Inherited by Stg::WorldGui.
Classes | |
class | Event |
Public Member Functions | |
virtual void | AddModel (Model *mod) |
void | AddModelName (Model *mod, const std::string &name) |
void | AddPowerPack (PowerPack *pp) |
SuperRegion * | AddSuperRegion (const stg_point_int_t &coord) |
void | AddUpdateCallback (stg_world_callback_t cb, void *user) |
void | CancelQuit () |
void | CancelQuitAll () |
void | ClearRays () |
virtual std::string | ClockString (void) const |
void | ConsumeQueue (unsigned int queue_num) |
Model * | CreateModel (Model *parent, const std::string &typestr) |
SuperRegion * | CreateSuperRegion (stg_point_int_t origin) |
void | DestroySuperRegion (SuperRegion *sr) |
void | Enqueue (unsigned int queue_num, stg_usec_t delay, Model *mod) |
void | ExpireSuperRegion (SuperRegion *sr) |
void | Extend (stg_point3_t pt) |
void | ForEachCellInLine (const stg_point_int_t &pt1, const stg_point_int_t &pt2, CellPtrVec &cells) |
unsigned int | GetEventQueue (Model *mod) |
const stg_bounds3d_t & | GetExtent () |
Model * | GetGround () |
Model * | GetModel (const std::string &name) const |
SuperRegion * | GetSuperRegion (const stg_point_int_t &coord) |
SuperRegion * | GetSuperRegionCached (int32_t x, int32_t y) |
SuperRegion * | GetSuperRegionCached (const stg_point_int_t &coord) |
uint64_t | GetUpdateCount () |
Worldfile * | GetWorldFile () |
virtual bool | IsGUI () const |
virtual void | Load (const char *worldfile_path) |
void | LoadBlock (Worldfile *wf, int entity) |
void | LoadBlockGroup (Worldfile *wf, int entity) |
void | LoadModel (Worldfile *wf, int entity) |
void | Log (Model *mod) |
stg_point_int_t | MetersToPixels (const stg_point_t &pt) |
int32_t | MetersToPixels (stg_meters_t x) |
void | NeedRedraw () |
bool | PastQuitTime () |
bool | Paused () |
virtual void | PopColor () |
virtual void | PushColor (double r, double g, double b, double a) |
virtual void | PushColor (Color col) |
void | Quit () |
void | QuitAll () |
void | Raytrace (const Pose &pose, const stg_meters_t range, const stg_radians_t fov, const stg_ray_test_func_t func, const Model *finder, const void *arg, stg_raytrace_result_t *samples, const uint32_t sample_count, const bool ztest) |
stg_raytrace_result_t | Raytrace (const Pose &pose, const stg_meters_t range, const stg_ray_test_func_t func, const Model *finder, const void *arg, const bool ztest) |
stg_raytrace_result_t | Raytrace (const Ray &ray) |
virtual Model * | RecentlySelectedModel () |
void | RecordRay (double x1, double y1, double x2, double y2) |
void | RegisterOption (Option *opt) |
Register an Option for pickup by the GUI. | |
virtual void | Reload () |
virtual void | RemoveModel (Model *mod) |
void | RemovePowerPack (PowerPack *pp) |
int | RemoveUpdateCallback (stg_world_callback_t cb, void *user) |
double | Resolution () |
virtual bool | Save (const char *filename) |
void | ShowClock (bool enable) |
Control printing time to stdout. | |
stg_usec_t | SimTimeNow (void) |
virtual void | Start () |
virtual void | Stop () |
bool | TestQuit () |
virtual void | TogglePause () |
void | TryCharge (PowerPack *pp, const Pose &pose) |
virtual void | UnLoad () |
virtual bool | Update (void) |
World (const std::string &name="MyWorld", double ppm=DEFAULT_PPM) | |
virtual | ~World () |
Static Public Member Functions | |
static void * | update_thread_entry (std::pair< World *, int > *info) |
static bool | UpdateAll () |
Public Attributes | |
std::set< Model * > | active_energy |
std::set< Model * > | active_velocity |
std::vector < std::priority_queue< Event > > | event_queues |
Model * | ground |
bool | paused |
if true, the simulation is stopped | |
PointIntVec | rt_candidate_cells |
PointIntVec | rt_cells |
stg_usec_t | sim_interval |
Static Public Attributes | |
static std::vector< std::string > | args |
static std::string | ctrlargs |
static const int | DEFAULT_PPM = 50 |
Protected Member Functions | |
void | CallUpdateCallbacks () |
Call all calbacks in cb_list, removing any that return true;. | |
Protected Attributes | |
std::list< std::pair < stg_world_callback_t, void * > > | cb_list |
List of callback functions and arguments. | |
stg_bounds3d_t | extent |
Describes the 3D volume of the world. | |
bool | graphics |
true iff we have a GUI | |
std::set< Option * > | option_table |
GUI options (toggles) registered by models. | |
std::list< PowerPack * > | powerpack_list |
List of all the powerpacks attached to models in the world. | |
stg_usec_t | quit_time |
std::list< float * > | ray_list |
List of rays traced for debug visualization. | |
stg_usec_t | sim_time |
the current sim time in this world in microseconds | |
SuperRegion * | sr_cached |
The last superregion looked up by this world. | |
std::map< stg_point_int_t, SuperRegion * > | superregions |
std::vector< ModelPtrVec > | update_lists |
uint64_t | updates |
the number of simulated time steps executed so far | |
Worldfile * | wf |
If set, points to the worldfile used to create this world. |
Detailed Description
World class
Constructor & Destructor Documentation
World::World | ( | const std::string & | name = "MyWorld" , |
|
double | ppm = DEFAULT_PPM | |||
) |
World::~World | ( | void | ) | [virtual] |
Member Function Documentation
void World::AddModel | ( | Model * | mod | ) | [virtual] |
void World::AddModelName | ( | Model * | mod, | |
const std::string & | name | |||
) |
void World::AddPowerPack | ( | PowerPack * | pp | ) |
SuperRegion * World::AddSuperRegion | ( | const stg_point_int_t & | coord | ) |
void World::AddUpdateCallback | ( | stg_world_callback_t | cb, | |
void * | user | |||
) |
Attach a callback function, to be called with the argument at the end of a complete update step
void World::CallUpdateCallbacks | ( | ) | [protected] |
Call all calbacks in cb_list, removing any that return true;.
void Stg::World::CancelQuit | ( | ) | [inline] |
void Stg::World::CancelQuitAll | ( | ) | [inline] |
void World::ClearRays | ( | ) |
std::string World::ClockString | ( | void | ) | const [virtual] |
Get human readable string that describes the current simulation time.
Reimplemented in Stg::WorldGui.
void World::ConsumeQueue | ( | unsigned int | queue_num | ) |
consume events from the queue up to and including the current sim_time
SuperRegion * World::CreateSuperRegion | ( | stg_point_int_t | origin | ) |
void World::DestroySuperRegion | ( | SuperRegion * | sr | ) |
void World::Enqueue | ( | unsigned int | queue_num, | |
stg_usec_t | delay, | |||
Model * | mod | |||
) |
void Stg::World::ExpireSuperRegion | ( | SuperRegion * | sr | ) |
void World::Extend | ( | stg_point3_t | pt | ) |
Enlarge the bounding volume to include this point
void World::ForEachCellInLine | ( | const stg_point_int_t & | pt1, | |
const stg_point_int_t & | pt2, | |||
CellPtrVec & | cells | |||
) |
add a Cell pointer to the vector for each cell on the line from pt1 to pt2 inclusive
unsigned int World::GetEventQueue | ( | Model * | mod | ) |
returns an event queue index number for a model to use for updates
const stg_bounds3d_t& Stg::World::GetExtent | ( | ) | [inline] |
Return the 3D bounding box of the world, in meters
Model* Stg::World::GetGround | ( | ) | [inline] |
Return the floor model
Model * World::GetModel | ( | const std::string & | name | ) | const |
Returns a pointer to the model identified by name, or NULL if nonexistent
SuperRegion * World::GetSuperRegion | ( | const stg_point_int_t & | coord | ) | [inline] |
SuperRegion * World::GetSuperRegionCached | ( | int32_t | x, | |
int32_t | y | |||
) | [inline] |
SuperRegion * World::GetSuperRegionCached | ( | const stg_point_int_t & | coord | ) | [inline] |
uint64_t Stg::World::GetUpdateCount | ( | ) | [inline] |
Return the number of times the world has been updated.
Worldfile* Stg::World::GetWorldFile | ( | ) | [inline] |
virtual bool Stg::World::IsGUI | ( | ) | const [inline, virtual] |
Reimplemented in Stg::WorldGui.
void World::Load | ( | const char * | worldfile_path | ) | [virtual] |
Reimplemented in Stg::WorldGui.
void World::LoadBlock | ( | Worldfile * | wf, | |
int | entity | |||
) |
void Stg::World::LoadBlockGroup | ( | Worldfile * | wf, | |
int | entity | |||
) |
void World::LoadModel | ( | Worldfile * | wf, | |
int | entity | |||
) |
stg_point_int_t Stg::World::MetersToPixels | ( | const stg_point_t & | pt | ) | [inline] |
int32_t Stg::World::MetersToPixels | ( | stg_meters_t | x | ) | [inline] |
convert a distance in meters to a distance in world occupancy grid pixels
void Stg::World::NeedRedraw | ( | ) | [inline] |
hint that the world needs to be redrawn if a GUI is attached
bool World::PastQuitTime | ( | ) |
Returns true iff the current time is greater than the time we should quit
bool Stg::World::Paused | ( | ) | [inline] |
virtual void Stg::World::PopColor | ( | ) | [inline, virtual] |
Reimplemented in Stg::WorldGui.
virtual void Stg::World::PushColor | ( | double | r, | |
double | g, | |||
double | b, | |||
double | a | |||
) | [inline, virtual] |
Reimplemented in Stg::WorldGui.
virtual void Stg::World::PushColor | ( | Color | col | ) | [inline, virtual] |
Reimplemented in Stg::WorldGui.
void Stg::World::Quit | ( | ) | [inline] |
void Stg::World::QuitAll | ( | ) | [inline] |
void World::Raytrace | ( | const Pose & | pose, | |
const stg_meters_t | range, | |||
const stg_radians_t | fov, | |||
const stg_ray_test_func_t | func, | |||
const Model * | finder, | |||
const void * | arg, | |||
stg_raytrace_result_t * | samples, | |||
const uint32_t | sample_count, | |||
const bool | ztest | |||
) |
stg_raytrace_result_t World::Raytrace | ( | const Pose & | pose, | |
const stg_meters_t | range, | |||
const stg_ray_test_func_t | func, | |||
const Model * | finder, | |||
const void * | arg, | |||
const bool | ztest | |||
) |
stg_raytrace_result_t World::Raytrace | ( | const Ray & | ray | ) |
trace a ray.
virtual Model* Stg::World::RecentlySelectedModel | ( | ) | [inline, virtual] |
void World::RecordRay | ( | double | x1, | |
double | y1, | |||
double | x2, | |||
double | y2 | |||
) |
store rays traced for debugging purposes
void World::Reload | ( | void | ) | [virtual] |
void World::RemoveModel | ( | Model * | mod | ) | [virtual] |
void World::RemovePowerPack | ( | PowerPack * | pp | ) |
int World::RemoveUpdateCallback | ( | stg_world_callback_t | cb, | |
void * | user | |||
) |
Remove a callback function. Any argument data passed to AddUpdateCallback is not automatically freed.
double Stg::World::Resolution | ( | ) | [inline] |
Get the resolution in pixels-per-metre of the underlying discrete raytracing model
bool World::Save | ( | const char * | filename | ) | [virtual] |
Reimplemented in Stg::WorldGui.
void Stg::World::ShowClock | ( | bool | enable | ) | [inline] |
Control printing time to stdout.
stg_usec_t World::SimTimeNow | ( | void | ) |
virtual void Stg::World::Start | ( | ) | [inline, virtual] |
Reimplemented in Stg::WorldGui.
virtual void Stg::World::Stop | ( | ) | [inline, virtual] |
Reimplemented in Stg::WorldGui.
bool Stg::World::TestQuit | ( | ) | [inline] |
virtual void Stg::World::TogglePause | ( | ) | [inline, virtual] |
void World::UnLoad | ( | ) | [virtual] |
Reimplemented in Stg::WorldGui.
bool World::Update | ( | void | ) | [virtual] |
Reimplemented in Stg::WorldGui.
void * World::update_thread_entry | ( | std::pair< World *, int > * | info | ) | [static] |
bool World::UpdateAll | ( | ) | [static] |
returns true when time to quit, false otherwise
Member Data Documentation
std::set<Model*> Stg::World::active_energy |
std::set<Model*> Stg::World::active_velocity |
std::vector< std::string > World::args [static] |
contains the command line arguments passed to Stg::Init(), so that controllers can read them.
std::list<std::pair<stg_world_callback_t,void*> > Stg::World::cb_list [protected] |
List of callback functions and arguments.
std::string World::ctrlargs [static] |
const int Stg::World::DEFAULT_PPM = 50 [static] |
std::vector<std::priority_queue<Event> > Stg::World::event_queues |
stg_bounds3d_t Stg::World::extent [protected] |
Describes the 3D volume of the world.
bool Stg::World::graphics [protected] |
true iff we have a GUI
Special model for the floor of the world
std::set<Option*> Stg::World::option_table [protected] |
GUI options (toggles) registered by models.
bool Stg::World::paused |
if true, the simulation is stopped
std::list<PowerPack*> Stg::World::powerpack_list [protected] |
List of all the powerpacks attached to models in the world.
stg_usec_t Stg::World::quit_time [protected] |
World::quit is set true when this simulation time is reached
std::list<float*> Stg::World::ray_list [protected] |
List of rays traced for debug visualization.
The amount of simulated time to run for each call to Update()
stg_usec_t Stg::World::sim_time [protected] |
the current sim time in this world in microseconds
SuperRegion* Stg::World::sr_cached [protected] |
The last superregion looked up by this world.
std::map<stg_point_int_t,SuperRegion*> Stg::World::superregions [protected] |
std::vector<ModelPtrVec> Stg::World::update_lists [protected] |
uint64_t Stg::World::updates [protected] |
the number of simulated time steps executed so far
Worldfile* Stg::World::wf [protected] |
If set, points to the worldfile used to create this world.
The documentation for this class was generated from the following files:
Generated on Tue Oct 20 15:42:06 2009 for Stage by
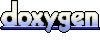