RFLEX Class Reference
Inheritance diagram for RFLEX:
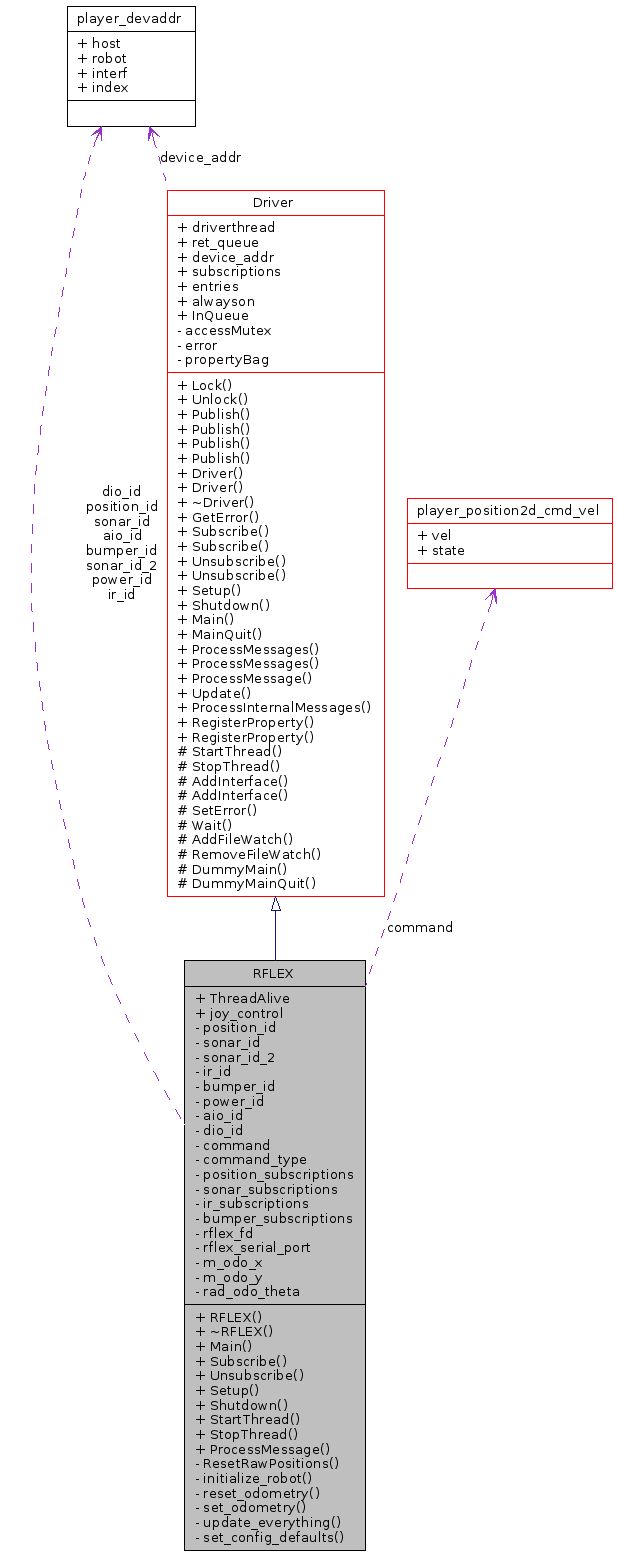
Detailed Description
Definition at line 96 of file rflex.h.
Public Member Functions | |
RFLEX (ConfigFile *cf, int section) | |
~RFLEX () | |
virtual void | Main () |
Main method for driver thread. | |
virtual int | Subscribe (player_devaddr_t addr) |
Subscribe to this driver. | |
virtual int | Unsubscribe (player_devaddr_t addr) |
Unsubscribe from this driver. | |
virtual int | Setup () |
Initialize the driver. | |
virtual int | Shutdown () |
Finalize the driver. | |
virtual void | StartThread (void) |
Start the driver thread. | |
virtual void | StopThread (void) |
Cancel (and wait for termination) of the driver thread. | |
int | ProcessMessage (QueuePointer &resp_queue, player_msghdr *hdr, void *data) |
Message handler. | |
Public Attributes | |
bool | ThreadAlive |
Static Public Attributes | |
static int | joy_control |
Private Member Functions | |
void | ResetRawPositions () |
int | initialize_robot () |
void | reset_odometry () |
void | set_odometry (float, float, float) |
void | update_everything (player_rflex_data_t *d) |
void | set_config_defaults () |
Private Attributes | |
player_devaddr_t | position_id |
player_devaddr_t | sonar_id |
player_devaddr_t | sonar_id_2 |
player_devaddr_t | ir_id |
player_devaddr_t | bumper_id |
player_devaddr_t | power_id |
player_devaddr_t | aio_id |
player_devaddr_t | dio_id |
player_position2d_cmd_vel_t | command |
int | command_type |
int | position_subscriptions |
int | sonar_subscriptions |
int | ir_subscriptions |
int | bumper_subscriptions |
int | rflex_fd |
char | rflex_serial_port [MAX_FILENAME_SIZE] |
double | m_odo_x |
double | m_odo_y |
double | rad_odo_theta |
Member Function Documentation
void RFLEX::Main | ( | ) | [virtual] |
Main method for driver thread.
drivers have their own thread of execution, created using StartThread(); this is the entry point for the driver thread, and must be overloaded by all threaded drivers.
Reimplemented from Driver.
Definition at line 928 of file rflex.cc.
References player_rflex_data::aio, aio_id, player_rflex_data::bumper, bumper_id, command_type, player_rflex_data::dio, dio_id, player_rflex_data::gripper, rflex_config_t::home_on_start, initialize_robot(), player_devaddr::interf, player_rflex_data::ir, ir_id, ir_subscriptions, joy_control, Driver::Lock(), rflex_config_t::mPsec2_trans_acceleration, rflex_config_t::mrad_sonar_poses, rflex_config_t::num_sonars, player_pose2d::pa, PLAYER_AIO_DATA_STATE, PLAYER_BUMPER_DATA_STATE, PLAYER_DIO_DATA_VALUES, PLAYER_ERROR, PLAYER_IR_DATA_RANGES, PLAYER_MSG1, PLAYER_MSGTYPE_DATA, PLAYER_POSITION2D_DATA_STATE, PLAYER_POWER_DATA_STATE, PLAYER_SONAR_DATA_GEOM, PLAYER_SONAR_DATA_RANGES, player_position2d_data::pos, player_sonar_geom::poses, player_sonar_geom::poses_count, player_rflex_data::position, position_id, position_subscriptions, player_rflex_data::power, power_id, Driver::ProcessMessages(), Driver::Publish(), player_pose3d::px, player_pose3d::py, player_pose3d::pyaw, rad_odo_theta, reset_odometry(), rflex_fd, player_rflex_data::sonar, player_rflex_data::sonar2, rflex_config_t::sonar_2nd_bank_start, sonar_id, sonar_id_2, sonar_subscriptions, ThreadAlive, Driver::Unlock(), update_everything(), and rflex_config_t::use_joystick.
Here is the call graph for this function:
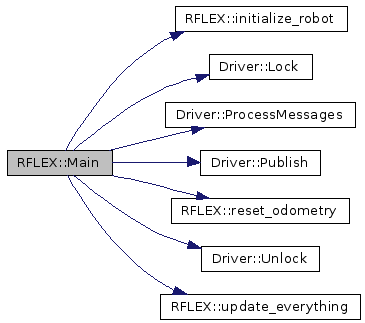
int RFLEX::Subscribe | ( | player_devaddr_t | addr | ) | [virtual] |
Subscribe to this driver.
The Subscribe() and Unsubscribe() methods are used to control subscriptions to the driver; a driver MAY override them, but usually won't.
- Parameters:
-
addr Address of the device to subscribe to (the driver may have more than one interface).
- Returns:
- Returns 0 on success.
Reimplemented from Driver.
Definition at line 866 of file rflex.cc.
References bumper_subscriptions, ir_subscriptions, PLAYER_BUMPER_CODE, PLAYER_IR_CODE, PLAYER_POSITION2D_CODE, PLAYER_SONAR_CODE, position_subscriptions, sonar_subscriptions, and Driver::Subscribe().
Here is the call graph for this function:

int RFLEX::Unsubscribe | ( | player_devaddr_t | addr | ) | [virtual] |
Unsubscribe from this driver.
The Subscribe() and Unsubscribe() methods are used to control subscriptions to the driver; a driver MAY override them, but usually won't.
- Parameters:
-
addr Address of the device to unsubscribe from (the driver may have more than one interface).
- Returns:
- Returns 0 on success.
Reimplemented from Driver.
Definition at line 895 of file rflex.cc.
References bumper_subscriptions, ir_subscriptions, PLAYER_BUMPER_CODE, PLAYER_IR_CODE, PLAYER_POSITION2D_CODE, PLAYER_SONAR_CODE, position_subscriptions, sonar_subscriptions, and Driver::Unsubscribe().
Here is the call graph for this function:

int RFLEX::Setup | ( | ) | [virtual] |
Initialize the driver.
This function is called with the first client subscribes; it MUST be implemented by the driver.
- Returns:
- Returns 0 on success.
Implements Driver.
Definition at line 820 of file rflex.cc.
References StartThread().
Here is the call graph for this function:

int RFLEX::Shutdown | ( | ) | [virtual] |
Finalize the driver.
This function is called with the last client unsubscribes; it MUST be implemented by the driver.
- Returns:
- Returns 0 on success.
Implements Driver.
Definition at line 826 of file rflex.cc.
References rflex_config_t::mPsec2_trans_acceleration, rflex_fd, and StopThread().
Here is the call graph for this function:

void RFLEX::StartThread | ( | void | ) | [virtual] |
Start the driver thread.
This method is usually called from the overloaded Setup() method to create the driver thread. This will call Main().
Reimplemented from Driver.
Definition at line 844 of file rflex.cc.
References Driver::driverthread, Driver::DummyMain(), and ThreadAlive.
Referenced by Setup().
Here is the call graph for this function:

void RFLEX::StopThread | ( | void | ) | [virtual] |
Cancel (and wait for termination) of the driver thread.
This method is usually called from the overloaded Shutdown() method to terminate the driver thread.
Reimplemented from Driver.
Definition at line 853 of file rflex.cc.
References Driver::driverthread, Driver::Lock(), ThreadAlive, and Driver::Unlock().
Referenced by Shutdown().
Here is the call graph for this function:

int RFLEX::ProcessMessage | ( | QueuePointer & | resp_queue, | |
player_msghdr * | hdr, | |||
void * | data | |||
) | [virtual] |
Message handler.
This function is called once for each message in the incoming queue. Reimplement it to provide message handling. Return 0 if you handled the message and -1 otherwise
- Parameters:
-
resp_queue The queue to which any response should go. hdr The message header data The message body
Reimplemented from Driver.
Definition at line 358 of file rflex.cc.
References rflex_config_t::bumper_count, rflex_config_t::bumper_def, player_bumper_geom::bumper_def, player_bumper_geom::bumper_def_count, bumper_id, command_type, ir_id, rflex_config_t::ir_poses, Driver::Lock(), rflex_config_t::m_length, rflex_config_t::m_width, Message::MatchMessage(), rflex_config_t::mrad_sonar_poses, rflex_config_t::num_sonars, player_pose2d::pa, PLAYER_BUMPER_REQ_GET_GEOM, PLAYER_IR_REQ_POSE, PLAYER_IR_REQ_POWER, PLAYER_MSGTYPE_CMD, PLAYER_MSGTYPE_REQ, PLAYER_MSGTYPE_RESP_ACK, PLAYER_POSITION2D_CMD_VEL, PLAYER_POSITION2D_REQ_GET_GEOM, PLAYER_POSITION2D_REQ_MOTOR_POWER, PLAYER_POSITION2D_REQ_RESET_ODOM, PLAYER_POSITION2D_REQ_SET_ODOM, PLAYER_POSITION2D_REQ_VELOCITY_MODE, PLAYER_SONAR_REQ_GET_GEOM, PLAYER_SONAR_REQ_POWER, player_position2d_set_odom_req::pose, player_sonar_geom::poses, player_sonar_geom::poses_count, position_id, Driver::Publish(), player_pose2d::px, player_pose2d::py, reset_odometry(), rflex_fd, set_odometry(), player_position2d_geom::size, player_bbox3d::sl, rflex_config_t::sonar_1st_bank_end, rflex_config_t::sonar_2nd_bank_start, sonar_id, sonar_id_2, player_ir_power_req::state, player_bbox3d::sw, and Driver::Unlock().
Here is the call graph for this function:
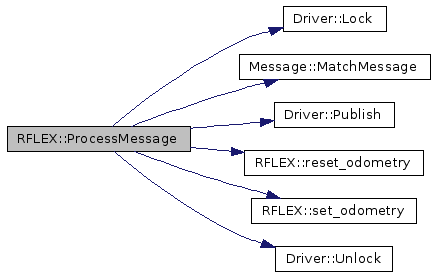
The documentation for this class was generated from the following files: