PlayerCc::PlannerProxy Class Reference
[Proxies]
#include <playerc++.h>
Inheritance diagram for PlayerCc::PlannerProxy:

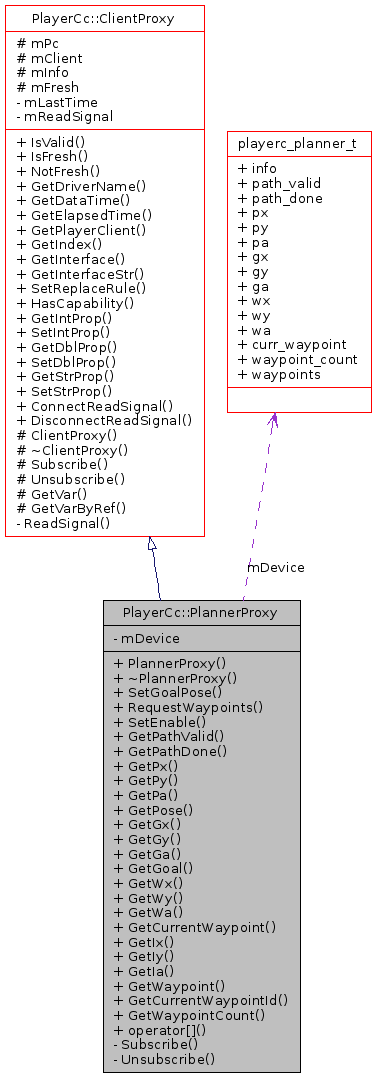
Detailed Description
ThePlannerProxy
proxy provides an interface to a 2D motion planner.
Definition at line 1453 of file playerc++.h.
Public Member Functions | |
PlannerProxy (PlayerClient *aPc, uint32_t aIndex=0) | |
constructor | |
~PlannerProxy () | |
destructor | |
void | SetGoalPose (double aGx, double aGy, double aGa) |
Set the goal pose (gx, gy, ga). | |
void | RequestWaypoints () |
Get the list of waypoints. | |
void | SetEnable (bool aEnable) |
Enable/disable the robot's motion. | |
uint32_t | GetPathValid () const |
Did the planner find a valid path? | |
uint32_t | GetPathDone () const |
Have we arrived at the goal? | |
double | GetPx () const |
Current pose (m). | |
double | GetPy () const |
Current pose (m). | |
double | GetPa () const |
Current pose (radians). | |
player_pose2d_t | GetPose () const |
Get the current pose. | |
double | GetGx () const |
Goal location (m). | |
double | GetGy () const |
Goal location (m). | |
double | GetGa () const |
Goal location (radians). | |
player_pose2d_t | GetGoal () const |
Get the goal. | |
double | GetWx () const |
Current waypoint location (m). | |
double | GetWy () const |
Current waypoint location (m). | |
double | GetWa () const |
Current waypoint location (rad). | |
player_pose2d_t | GetCurrentWaypoint () const |
Get the current waypoint. | |
double | GetIx (int i) const |
Grab a particular waypoint location (m). | |
double | GetIy (int i) const |
Grab a particular waypoint location (m). | |
double | GetIa (int i) const |
Grab a particular waypoint location (rad). | |
player_pose2d_t | GetWaypoint (uint32_t aIndex) const |
Get the waypoint. | |
int | GetCurrentWaypointId () const |
Current waypoint index (handy if you already have the list of waypoints). | |
uint32_t | GetWaypointCount () const |
Number of waypoints in the plan. | |
player_pose2d_t | operator[] (uint32_t aIndex) const |
Waypoint access operator This operator provides an alternate way of access the waypoint data. | |
Private Member Functions | |
void | Subscribe (uint32_t aIndex) |
void | Unsubscribe () |
Private Attributes | |
playerc_planner_t * | mDevice |
Member Function Documentation
void PlayerCc::PlannerProxy::RequestWaypoints | ( | ) |
Get the list of waypoints.
Writes the result into the proxy rather than returning it to the caller.
void PlayerCc::PlannerProxy::SetEnable | ( | bool | aEnable | ) |
Enable/disable the robot's motion.
Set state to true to enable, false to disable.
double PlayerCc::PlannerProxy::GetPx | ( | ) | const [inline] |
Current pose (m).
- Deprecated:
- use GetPose() instead
Definition at line 1490 of file playerc++.h.
References PlayerCc::ClientProxy::GetVar(), mDevice, and playerc_planner_t::px.
Here is the call graph for this function:

double PlayerCc::PlannerProxy::GetPy | ( | ) | const [inline] |
Current pose (m).
- Deprecated:
- use GetPose() instead
Definition at line 1493 of file playerc++.h.
References PlayerCc::ClientProxy::GetVar(), mDevice, and playerc_planner_t::py.
Here is the call graph for this function:

double PlayerCc::PlannerProxy::GetPa | ( | ) | const [inline] |
Current pose (radians).
- Deprecated:
- use GetPose() instead
Definition at line 1496 of file playerc++.h.
References PlayerCc::ClientProxy::GetVar(), mDevice, and playerc_planner_t::pa.
Here is the call graph for this function:

double PlayerCc::PlannerProxy::GetGx | ( | ) | const [inline] |
Goal location (m).
- Deprecated:
- use GetGoal() instead
Definition at line 1511 of file playerc++.h.
References PlayerCc::ClientProxy::GetVar(), playerc_planner_t::gx, and mDevice.
Here is the call graph for this function:

double PlayerCc::PlannerProxy::GetGy | ( | ) | const [inline] |
Goal location (m).
- Deprecated:
- use GetGoal() instead
Definition at line 1514 of file playerc++.h.
References PlayerCc::ClientProxy::GetVar(), playerc_planner_t::gy, and mDevice.
Here is the call graph for this function:

double PlayerCc::PlannerProxy::GetGa | ( | ) | const [inline] |
Goal location (radians).
- Deprecated:
- use GetGoal() instead
Definition at line 1517 of file playerc++.h.
References playerc_planner_t::ga, PlayerCc::ClientProxy::GetVar(), and mDevice.
Here is the call graph for this function:

double PlayerCc::PlannerProxy::GetWx | ( | ) | const [inline] |
Current waypoint location (m).
- Deprecated:
- use GetCurWaypoint() instead
Definition at line 1532 of file playerc++.h.
References PlayerCc::ClientProxy::GetVar(), mDevice, and playerc_planner_t::wx.
Here is the call graph for this function:

double PlayerCc::PlannerProxy::GetWy | ( | ) | const [inline] |
Current waypoint location (m).
- Deprecated:
- use GetCurWaypoint() instead
Definition at line 1535 of file playerc++.h.
References PlayerCc::ClientProxy::GetVar(), mDevice, and playerc_planner_t::wy.
Here is the call graph for this function:

double PlayerCc::PlannerProxy::GetWa | ( | ) | const [inline] |
Current waypoint location (rad).
- Deprecated:
- use GetCurWaypoint() instead
Definition at line 1538 of file playerc++.h.
References PlayerCc::ClientProxy::GetVar(), mDevice, and playerc_planner_t::wa.
Here is the call graph for this function:

double PlayerCc::PlannerProxy::GetIx | ( | int | i | ) | const |
double PlayerCc::PlannerProxy::GetIy | ( | int | i | ) | const |
double PlayerCc::PlannerProxy::GetIa | ( | int | i | ) | const |
int PlayerCc::PlannerProxy::GetCurrentWaypointId | ( | ) | const [inline] |
Current waypoint index (handy if you already have the list of waypoints).
May be negative if there's no plan, or if the plan is done
Definition at line 1576 of file playerc++.h.
References playerc_planner_t::curr_waypoint, PlayerCc::ClientProxy::GetVar(), and mDevice.
Here is the call graph for this function:

player_pose2d_t PlayerCc::PlannerProxy::operator[] | ( | uint32_t | aIndex | ) | const [inline] |
Waypoint access operator This operator provides an alternate way of access the waypoint data.
For example, given a PlannerProxy
named pp
, the following expressions are equivalent: pp.GetWaypoint(0)
and pp
[0].
Definition at line 1587 of file playerc++.h.
References GetWaypoint().
Here is the call graph for this function:

The documentation for this class was generated from the following file: