stage_internal.h
Go to the documentation of this file.00001 #ifndef _STAGE_INTERNAL_H 00002 #define _STAGE_INTERNAL_H 00003 00004 // internal function declarations that are not part of the external 00005 // interface to Stage 00006 00007 #include "stage.h" 00008 #include "math.h" // for lrint() in macros 00009 00010 00018 #ifdef __cplusplus 00019 extern "C" { 00020 #endif 00021 00023 typedef struct 00024 { 00025 stg_rtk_canvas_t* canvas; 00026 00027 stg_world_t* world; // every window shows a single world 00028 00029 // stg_rtk doesn't support status bars, so we'll use gtk directly 00030 GtkStatusbar* statusbar; 00031 GtkLabel* timelabel; 00032 00033 int wf_section; // worldfile section for load/save 00034 00035 stg_rtk_fig_t* bg; // background 00036 stg_rtk_fig_t* matrix; 00037 stg_rtk_fig_t* matrix_tree; 00038 stg_rtk_fig_t* poses; 00039 00040 gboolean show_matrix; 00041 gboolean fill_polygons; 00042 gboolean show_geom; 00043 gboolean show_polygons; 00044 gboolean show_grid; 00045 00046 int frame_series; 00047 int frame_index; 00048 int frame_callback_tag; 00049 int frame_interval; 00050 int frame_format; 00051 00052 stg_model_t* selection_active; 00053 00054 } gui_window_t; 00055 00056 void gui_startup( int* argc, char** argv[] ); 00057 void gui_poll( void ); 00058 void gui_shutdown( void ); 00059 00060 void gui_load( gui_window_t* win, int section ); 00061 void gui_save( gui_window_t* win ); 00062 00063 gui_window_t* gui_world_create( stg_world_t* world ); 00064 void gui_world_destroy( stg_world_t* world ); 00065 int gui_world_update( stg_world_t* world ); 00066 void stg_world_add_model( stg_world_t* world, stg_model_t* mod ); 00067 void gui_world_geom( stg_world_t* world ); 00068 00069 void gui_model_create( stg_model_t* model ); 00070 void gui_model_destroy( stg_model_t* model ); 00071 void gui_model_display_pose( stg_model_t* mod, char* verb ); 00072 void gui_model_features( stg_model_t* mod ); 00073 void gui_model_geom( stg_model_t* model ); 00074 void gui_model_mouse(stg_rtk_fig_t *fig, int event, int mode); 00075 void gui_model_move( stg_model_t* mod ); 00076 void gui_model_nose( stg_model_t* model ); 00077 void gui_model_polygons( stg_model_t* model ); 00078 void gui_model_render_command( stg_model_t* mod ); 00079 void gui_model_render_config( stg_model_t* mod ); 00080 void gui_model_render_data( stg_model_t* mod ); 00081 void gui_window_menus_create( gui_window_t* win ); 00082 void gui_window_menus_destroy( gui_window_t* win ); 00083 00084 void gui_add_view_item( const gchar *name, 00085 const gchar *label, 00086 const gchar *tooltip, 00087 GCallback callback, 00088 gboolean is_active, 00089 void* userdata ); 00090 00091 // callback functions 00092 //typedef void(*func_init_t)(struct _stg_model*); 00093 typedef int(*func_update_t)(struct _stg_model*); 00094 typedef int(*func_startup_t)(struct _stg_model*); 00095 typedef int(*func_shutdown_t)(struct _stg_model*); 00096 typedef void(*func_load_t)(struct _stg_model*); 00097 typedef void(*func_save_t)(struct _stg_model*); 00098 00099 00100 struct _stg_property; 00101 00110 typedef void (*stg_property_storage_func_t)( struct _stg_property* prop, 00111 void* data, size_t len ); 00112 00118 typedef struct _stg_property 00119 { 00120 char name[STG_PROPNAME_MAX]; 00121 void* data; 00122 size_t len; 00123 stg_property_storage_func_t storage_func; 00124 GList* callbacks; // functions called when this property is set 00125 stg_model_t* mod; // the model to which this property belongs 00126 } stg_property_t; 00127 00128 typedef struct 00129 { 00130 stg_model_t* mod; 00131 char propname[STG_PROPNAME_MAX]; 00132 void* data; 00133 size_t len; 00134 void* user; 00135 } stg_property_callback_args_t; 00136 00137 typedef struct 00138 { 00139 stg_model_t* mod; 00140 //char propname[STG_PROPNAME_MAX]; 00141 const char *propname; 00142 stg_property_callback_t callback_on; 00143 stg_property_callback_t callback_off; 00144 void* arg_on; // argument to callback_on 00145 void* arg_off; // argument to callback_off 00146 } stg_property_toggle_args_t; 00147 00148 00149 struct _stg_model 00150 { 00151 stg_id_t id; // used as hash table key 00152 stg_world_t* world; // pointer to the world in which this model exists 00153 char* token; // automatically-generated unique ID string 00154 int type; // what kind of a model am I? 00155 00156 struct _stg_model *parent; // the model that owns this one, possibly NULL 00157 00158 GPtrArray* children; // the models owned by this model 00159 00160 // a datalist can contain arbitrary named data items. Can be used 00161 // by derived model types to store properties, and for user code 00162 // to associate arbitrary items with a model. 00163 GData* props; 00164 00165 // a datalist of stg_rtk_figs, indexed by name (string) 00166 GData* figs; 00167 00168 // the number of children of each type is counted so we can 00169 // automatically generate names for them 00170 int child_type_count[256]; 00171 00172 int subs; // the number of subscriptions to this model 00173 00174 stg_msec_t interval; // time between updates in ms 00175 stg_msec_t interval_elapsed; // time since last update in ms 00176 00177 stg_bool_t disabled; // if non-zero, the model is disabled 00178 00179 // type-dependent functions for this model, implementing simple 00180 // polymorphism 00181 stg_model_initializer_t initializer; 00182 func_startup_t f_startup; 00183 func_shutdown_t f_shutdown; 00184 func_update_t f_update; 00185 func_load_t f_load; 00186 func_save_t f_save; 00187 00188 /* TOFO - thread-safe version */ 00189 00190 // allow exclusive access to this model's properties 00191 pthread_mutex_t mutex; 00192 00193 /* END TODO */ 00194 }; 00195 00196 typedef struct { 00197 const char* keyword; 00198 stg_model_initializer_t initializer; 00199 } stg_type_record_t; 00200 00201 // internal functions 00202 00203 int _model_update( stg_model_t* mod ); 00204 int _model_startup( stg_model_t* mod ); 00205 int _model_shutdown( stg_model_t* mod ); 00206 00207 void stg_model_update_velocity( stg_model_t* model ); 00208 int stg_model_update_pose( stg_model_t* model ); 00209 void stg_model_energy_consume( stg_model_t* mod, stg_watts_t rate ); 00210 void stg_model_map( stg_model_t* mod, gboolean render ); 00211 void stg_model_map_with_children( stg_model_t* mod, gboolean render ); 00212 00213 stg_rtk_fig_t* stg_model_prop_fig_create( stg_model_t* mod, 00214 stg_rtk_fig_t* array[], 00215 stg_id_t propid, 00216 stg_rtk_fig_t* parent, 00217 int layer ); 00218 00219 void stg_model_render_geom( stg_model_t* mod ); 00220 void stg_model_render_pose( stg_model_t* mod ); 00221 void stg_model_render_polygons( stg_model_t* mod ); 00222 00223 int stg_fig_clear_cb( stg_model_t* mod, char* name, 00224 void* data, size_t len, void* userp ); 00225 00226 stg_rtk_fig_t* stg_model_fig_create( stg_model_t* mod, 00227 const char* figname, 00228 const char* parentname, 00229 int layer ); 00230 00231 stg_rtk_fig_t* stg_model_get_fig( stg_model_t* mod, const char* figname ); 00232 void stg_model_fig_clear( stg_model_t* mod, const char* figname ); 00233 00234 void stg_property_refresh( stg_property_t* prop ); 00235 void stg_property_destroy( stg_property_t* prop ); 00236 00240 void stg_model_set_property_ex( stg_model_t* mod, 00241 const char* prop, 00242 void* data, 00243 size_t len, 00244 stg_property_storage_func_t func ); 00245 00246 // defines a simulated world 00247 struct _stg_world 00248 { 00249 stg_id_t id; 00250 00251 GHashTable* models; 00252 GHashTable* models_by_name; 00253 00254 stg_meters_t width; 00255 stg_meters_t height; 00256 00260 int child_type_count[256]; 00261 00262 struct _stg_matrix* matrix; 00263 00264 char* token; 00265 00266 stg_msec_t sim_time; 00267 stg_msec_t sim_interval; 00268 00271 stg_msec_t wall_interval; 00272 00273 stg_msec_t wall_last_update; 00274 00279 stg_msec_t real_interval_measured; 00280 00281 double ppm; 00282 00283 gboolean paused; 00284 00285 gboolean destroy; 00286 00287 gui_window_t* win; 00288 00289 int subs; 00290 }; 00291 00292 // ROTATED RECTANGLES ------------------------------------------------- 00293 00299 typedef struct 00300 { 00301 stg_pose_t pose; 00302 stg_size_t size; 00303 } stg_rotrect_t; // rotated rectangle 00304 00308 void stg_rotrects_normalize( stg_rotrect_t* rects, int num ); 00309 00315 int stg_rotrects_from_image_file( const char* filename, 00316 stg_rotrect_t** rects, 00317 int* rect_count, 00318 int* widthp, int* heightp ); 00319 00320 00326 stg_polygon_t* stg_polygons_from_rotrects( stg_rotrect_t* rects, size_t count ); 00327 00331 // MATRIX ----------------------------------------------------------------------- 00332 00339 typedef struct stg_cell 00340 { 00341 void* data; 00342 double x, y; 00343 double size; 00344 00345 // bounding box 00346 double xmin,ymin,xmax,ymax; 00347 00348 stg_rtk_fig_t* fig; // for debugging 00349 00350 struct stg_cell* children[4]; 00351 struct stg_cell* parent; 00352 } stg_cell_t; 00353 00356 stg_cell_t* stg_cell_locate( stg_cell_t* cell, double x, double y ); 00357 00358 void stg_cell_unrender( stg_cell_t* cell ); 00359 void stg_cell_render( stg_cell_t* cell ); 00360 void stg_cell_render_tree( stg_cell_t* cell ); 00361 void stg_cell_unrender_tree( stg_cell_t* cell ); 00362 00364 typedef struct _stg_matrix 00365 { 00366 double ppm; // pixels per meter (1/resolution) 00367 double width, height; 00368 00369 // A quad tree of cells. Each leaf node contains a list of 00370 // pointers to objects located at that cell 00371 stg_cell_t* root; 00372 00373 // hash table stores all the pointers to objects rendered in the 00374 // quad tree, each associated with a list of cells in which it is 00375 // rendered. This allows us to remove objects from the tree 00376 // without doing the geometry again 00377 GHashTable* ptable; 00378 00379 /* TODO */ 00380 // lists of cells that have changed recently. This is used by the 00381 // GUI to render cells very quickly, and could also be used by devices 00382 //GSList* cells_changed; 00383 00384 // debug figure. if this is non-NULL, debug info is drawn here 00385 stg_rtk_fig_t* fig; 00386 00387 // todo - record a timestamp for matrix mods so devices can see if 00388 //the matrix has changed since they last peeked into it 00389 // stg_msec_t last_mod_time; 00390 } stg_matrix_t; 00391 00392 00395 stg_matrix_t* stg_matrix_create( double ppm, double width, double height ); 00396 00400 void stg_matrix_destroy( stg_matrix_t* matrix ); 00401 00404 void stg_matrix_clear( stg_matrix_t* matrix ); 00405 00408 void* stg_matrix_cell_get( stg_matrix_t* matrix, int r, double x, double y); 00409 00412 void stg_matrix_cell_append( stg_matrix_t* matrix, 00413 double x, double y, void* object ); 00414 00417 void stg_matrix_remove_obect( stg_matrix_t* matrix, void* object ); 00418 00421 void stg_matrix_cell_remove( stg_matrix_t* matrix, 00422 double x, double y, void* object ); 00423 00427 void stg_matrix_rectangle( stg_matrix_t* matrix, 00428 double px, double py, double pth, 00429 double dx, double dy, 00430 void* object ); 00431 00434 void stg_matrix_line( stg_matrix_t* matrix, 00435 double x1, double y1, 00436 double x2, double y2, 00437 void* object ); 00438 00441 typedef struct 00442 { 00443 stg_meters_t x1, y1, x2, y2; 00444 } stg_line_t; 00445 00446 00449 void stg_matrix_lines( stg_matrix_t* matrix, 00450 stg_line_t* lines, int num_lines, 00451 void* object ); 00452 00455 void stg_matrix_polygons( stg_matrix_t* matrix, 00456 double x, double y, double a, 00457 stg_polygon_t* polys, int num_polys, 00458 void* object ); 00459 00462 void stg_matrix_remove_object( stg_matrix_t* matrix, void* object ); 00463 00466 // RAYTRACE ITERATORS ------------------------------------------------------------- 00467 00472 typedef struct 00473 { 00474 double x, y, a; 00475 double cosa, sina, tana; 00476 double range; 00477 double max_range; 00478 double* incr; 00479 00480 GSList* models; 00481 int index; 00482 stg_matrix_t* matrix; 00483 00484 } itl_t; 00485 00486 typedef enum { PointToPoint=0, PointToBearingRange } itl_mode_t; 00487 00488 typedef int(*stg_itl_test_func_t)(stg_model_t* finder, stg_model_t* found ); 00489 00490 itl_t* itl_create( double x, double y, double a, double b, 00491 stg_matrix_t* matrix, itl_mode_t pmode ); 00492 00493 void itl_destroy( itl_t* itl ); 00494 void itl_raytrace( itl_t* itl ); 00495 00496 stg_model_t* itl_first_matching( itl_t* itl, 00497 stg_itl_test_func_t func, 00498 stg_model_t* finder ); 00499 00506 // C wrappers for C++ worldfile functions 00507 int wf_property_exists( int section, char* token ); 00508 int wf_read_int( int section, char* token, int def ); 00509 double wf_read_length( int section, char* token, double def ); 00510 double wf_read_angle( int section, char* token, double def ); 00511 double wf_read_float( int section, char* token, double def ); 00512 const char* wf_read_tuple_string( int section, char* token, int index, char* def ); 00513 double wf_read_tuple_float( int section, char* token, int index, double def ); 00514 double wf_read_tuple_length( int section, char* token, int index, double def ); 00515 double wf_read_tuple_angle( int section, char* token, int index, double def ); 00516 const char* wf_read_string( int section, char* token, char* def ); 00517 00518 void wf_write_int( int section, char* token, int value ); 00519 void wf_write_length( int section, char* token, double value ); 00520 void wf_write_angle( int section, char* token, double value ); 00521 void wf_write_float( int section, char* token, double value ); 00522 void wf_write_tuple_string( int section, char* token, int index, char* value ); 00523 void wf_write_tuple_float( int section, char* token, int index, double value ); 00524 void wf_write_tuple_length( int section, char* token, int index, double value ); 00525 void wf_write_tuple_angle( int section, char* token, int index, double value ); 00526 void wf_write_string( int section, char* token, char* value ); 00527 00528 void wf_save( void ); 00529 void wf_load( char* path ); 00530 int wf_section_count( void ); 00531 const char* wf_get_section_type( int section ); 00532 int wf_get_parent_section( int section ); 00533 const char* wf_get_filename( void); 00534 00537 // CALLBACK WRAPPERS ------------------------------------------------------------ 00538 00539 // callback wrappers for other functions 00540 void model_update_cb( gpointer key, gpointer value, gpointer user ); 00541 void model_print_cb( gpointer key, gpointer value, gpointer user ); 00542 void model_destroy_cb( gpointer mod ); 00543 00544 // Error macros - output goes to stderr 00545 #define PRINT_ERR(m) fprintf( stderr, "\033[41merr\033[0m: "m" (%s %s)\n", __FILE__, __FUNCTION__) 00546 #define PRINT_ERR1(m,a) fprintf( stderr, "\033[41merr\033[0m: "m" (%s %s)\n", a, __FILE__, __FUNCTION__) 00547 #define PRINT_ERR2(m,a,b) fprintf( stderr, "\033[41merr\033[0m: "m" (%s %s)\n", a, b, __FILE__, __FUNCTION__) 00548 #define PRINT_ERR3(m,a,b,c) fprintf( stderr, "\033[41merr\033[0m: "m" (%s %s)\n", a, b, c, __FILE__, __FUNCTION__) 00549 #define PRINT_ERR4(m,a,b,c,d) fprintf( stderr, "\033[41merr\033[0m: "m" (%s %s)\n", a, b, c, d, __FILE__, __FUNCTION__) 00550 #define PRINT_ERR5(m,a,b,c,d,e) fprintf( stderr, "\033[41merr\033[0m: "m" (%s %s)\n", a, b, c, d, e, __FILE__, __FUNCTION__) 00551 00552 // Warning macros 00553 #define PRINT_WARN(m) printf( "\033[44mwarn\033[0m: "m" (%s %s)\n", __FILE__, __FUNCTION__) 00554 #define PRINT_WARN1(m,a) printf( "\033[44mwarn\033[0m: "m" (%s %s)\n", a, __FILE__, __FUNCTION__) 00555 #define PRINT_WARN2(m,a,b) printf( "\033[44mwarn\033[0m: "m" (%s %s)\n", a, b, __FILE__, __FUNCTION__) 00556 #define PRINT_WARN3(m,a,b,c) printf( "\033[44mwarn\033[0m: "m" (%s %s)\n", a, b, c, __FILE__, __FUNCTION__) 00557 #define PRINT_WARN4(m,a,b,c,d) printf( "\033[44mwarn\033[0m: "m" (%s %s)\n", a, b, c, d, __FILE__, __FUNCTION__) 00558 #define PRINT_WARN5(m,a,b,c,d,e) printf( "\033[44mwarn\033[0m: "m" (%s %s)\n", a, b, c, d, e, __FILE__, __FUNCTION__) 00559 00560 // Message macros 00561 #ifdef DEBUG 00562 #define PRINT_MSG(m) printf( "stage: "m" (%s %s)\n", __FILE__, __FUNCTION__) 00563 #define PRINT_MSG1(m,a) printf( "stage: "m" (%s %s)\n", a, __FILE__, __FUNCTION__) 00564 #define PRINT_MSG2(m,a,b) printf( "stage: "m" (%s %s)\n", a, b, __FILE__, __FUNCTION__) 00565 #define PRINT_MSG3(m,a,b,c) printf( "stage: "m" (%s %s)\n", a, b, c, __FILE__, __FUNCTION__) 00566 #define PRINT_MSG4(m,a,b,c,d) printf( "stage: "m" (%s %s)\n", a, b, c, d, __FILE__, __FUNCTION__) 00567 #define PRINT_MSG5(m,a,b,c,d,e) printf( "stage: "m" (%s %s)\n", a, b, c, d, e,__FILE__, __FUNCTION__) 00568 #else 00569 #define PRINT_MSG(m) printf( "stage: "m"\n" ) 00570 #define PRINT_MSG1(m,a) printf( "stage: "m"\n", a) 00571 #define PRINT_MSG2(m,a,b) printf( "stage: "m"\n,", a, b ) 00572 #define PRINT_MSG3(m,a,b,c) printf( "stage: "m"\n", a, b, c ) 00573 #define PRINT_MSG4(m,a,b,c,d) printf( "stage: "m"\n", a, b, c, d ) 00574 #define PRINT_MSG5(m,a,b,c,d,e) printf( "stage: "m"\n", a, b, c, d, e ) 00575 #endif 00576 00577 // DEBUG macros 00578 #ifdef DEBUG 00579 #define PRINT_DEBUG(m) printf( "debug: "m" (%s %s)\n", __FILE__, __FUNCTION__) 00580 #define PRINT_DEBUG1(m,a) printf( "debug: "m" (%s %s)\n", a, __FILE__, __FUNCTION__) 00581 #define PRINT_DEBUG2(m,a,b) printf( "debug: "m" (%s %s)\n", a, b, __FILE__, __FUNCTION__) 00582 #define PRINT_DEBUG3(m,a,b,c) printf( "debug: "m" (%s %s)\n", a, b, c, __FILE__, __FUNCTION__) 00583 #define PRINT_DEBUG4(m,a,b,c,d) printf( "debug: "m" (%s %s)\n", a, b, c ,d, __FILE__, __FUNCTION__) 00584 #define PRINT_DEBUG5(m,a,b,c,d,e) printf( "debug: "m" (%s %s)\n", a, b, c ,d, e, __FILE__, __FUNCTION__) 00585 #else 00586 #define PRINT_DEBUG(m) 00587 #define PRINT_DEBUG1(m,a) 00588 #define PRINT_DEBUG2(m,a,b) 00589 #define PRINT_DEBUG3(m,a,b,c) 00590 #define PRINT_DEBUG4(m,a,b,c,d) 00591 #define PRINT_DEBUG5(m,a,b,c,d,e) 00592 #endif 00593 00594 00595 // end documentation group stage 00601 // TODO - some of this needs to be implemented, the rest junked. 00602 00603 /* // -------------------------------------------------------------- */ 00604 00605 /* // standard energy consumption of some devices in W. */ 00606 /* // */ 00607 /* // The MOTIONKG value is a hack to approximate cost of motion, as */ 00608 /* // Stage does not yet have an acceleration model. */ 00609 /* // */ 00610 /* #define STG_ENERGY_COST_LASER 20.0 // 20 Watts! (LMS200 - from SICK web site) */ 00611 /* #define STG_ENERGY_COST_FIDUCIAL 10.0 // 10 Watts */ 00612 /* #define STG_ENERGY_COST_RANGER 0.5 // 500mW (estimate) */ 00613 /* #define STG_ENERGY_COST_MOTIONKG 10.0 // 10 Watts per KG when moving */ 00614 /* #define STG_ENERGY_COST_BLOB 4.0 // 4W (estimate) */ 00615 00616 /* typedef struct */ 00617 /* { */ 00618 /* stg_joules_t joules; // current energy stored in Joules/1000 */ 00619 /* stg_watts_t watts; // current power expenditure in mW (mJoules/sec) */ 00620 /* int charging; // 1 if we are receiving energy, -1 if we are */ 00621 /* // supplying energy, 0 if we are neither charging nor */ 00622 /* // supplying energy. */ 00623 /* stg_meters_t range; // the range that our charging probe hit a charger */ 00624 /* } stg_energy_data_t; */ 00625 00626 /* typedef struct */ 00627 /* { */ 00628 /* stg_joules_t capacity; // maximum energy we can store (we start fully charged) */ 00629 /* stg_meters_t probe_range; // the length of our recharge probe */ 00630 /* //stg_pose_t probe_pose; // TODO - the origin of our probe */ 00631 00632 /* stg_watts_t give_rate; // give this many Watts to a probe that hits me (possibly 0) */ 00633 00634 /* stg_watts_t trickle_rate; // this much energy is consumed or */ 00635 /* // received by this device per second as a */ 00636 /* // baseline trickle. Positive values mean */ 00637 /* // that the device is just burning energy */ 00638 /* // stayting alive, which is appropriate */ 00639 /* // for most devices. Negative values mean */ 00640 /* // that the device is receiving energy */ 00641 /* // from the environment, simulating a */ 00642 /* // solar cell or some other ambient energy */ 00643 /* // collector */ 00644 00645 /* } stg_energy_config_t; */ 00646 00647 00648 /* // BLINKENLIGHT ------------------------------------------------------------ */ 00649 00650 /* // a number of milliseconds, used for example as the blinkenlight interval */ 00651 /* #define STG_LIGHT_ON UINT_MAX */ 00652 /* #define STG_LIGHT_OFF 0 */ 00653 00654 //typedef int stg_interval_ms_t; 00655 00656 00657 /* // TOKEN ----------------------------------------------------------------------- */ 00658 /* // token stuff for parsing worldfiles - this may one day replace 00659 the worldfile c++ code */ 00660 00661 /* #define CFG_OPEN '(' */ 00662 /* #define CFG_CLOSE ')' */ 00663 /* #define STR_OPEN '\"' */ 00664 /* #define STR_CLOSE '\"' */ 00665 /* #define TPL_OPEN '[' */ 00666 /* #define TPL_CLOSE ']' */ 00667 00668 /* typedef enum { */ 00669 /* STG_T_NUM = 0, */ 00670 /* STG_T_BOOLEAN, */ 00671 /* STG_T_MODELPROP, */ 00672 /* STG_T_WORLDPROP, */ 00673 /* STG_T_NAME, */ 00674 /* STG_T_STRING, */ 00675 /* STG_T_KEYWORD, */ 00676 /* STG_T_CFG_OPEN, */ 00677 /* STG_T_CFG_CLOSE, */ 00678 /* STG_T_TPL_OPEN, */ 00679 /* STG_T_TPL_CLOSE, */ 00680 /* } stg_token_type_t; */ 00681 00682 00683 00684 00685 /* typedef struct stg_token */ 00686 /* { */ 00687 /* char* token; ///< the text of the token */ 00688 /* stg_token_type_t type; ///< the type of the token */ 00689 /* unsigned int line; ///< the line on which the token appears */ 00690 00691 /* struct stg_token* next; ///< linked list support */ 00692 /* struct stg_token* child; ///< tree support */ 00693 00694 /* } stg_token_t; */ 00695 00696 /* stg_token_t* stg_token_next( stg_token_t* tokens ); */ 00697 /* /// print [token] formatted for a human reader, with a string [prefix] */ 00698 /* void stg_token_print( char* prefix, stg_token_t* token ); */ 00699 00700 /* /// print a token array suitable for human reader */ 00701 /* void stg_tokens_print( stg_token_t* tokens ); */ 00702 /* void stg_tokens_free( stg_token_t* tokens ); */ 00703 00704 /* /// create a new token structure from the arguments */ 00705 /* stg_token_t* stg_token_create( const char* token, stg_token_type_t type, int line ); */ 00706 00707 /* /// add a token to a list */ 00708 /* stg_token_t* stg_token_append( stg_token_t* head, */ 00709 /* char* token, stg_token_type_t type, int line ); */ 00710 00711 /* const char* stg_token_type_string( stg_token_type_t type ); */ 00712 00713 /* const char* stg_model_type_string( stg_model_type_t type ); */ 00714 00715 /* // functions for parsing worldfiles */ 00716 /* stg_token_t* stg_tokenize( FILE* wf ); */ 00717 /* //stg_world_t* sc_load_worldblock( stg_client_t* cli, stg_token_t** tokensptr ); */ 00718 /* //stg_model_t* sc_load_modelblock( stg_world_t* world, stg_model_t* parent, */ 00719 /* // stg_token_t** tokensptr ); */ 00720 00721 00722 00723 00724 #ifdef __cplusplus 00725 } 00726 #endif 00727 00729 // end of libstage_internal documentation 00730 00731 #endif // _STAGE_INTERNAL_H 00732
Generated on Thu Aug 11 13:08:10 2005 for Stage by
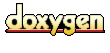