This class controls the turn on and turn off of e-puck LEDs. More...
#include <epuckLEDs.hpp>
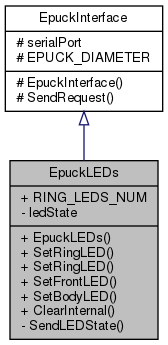
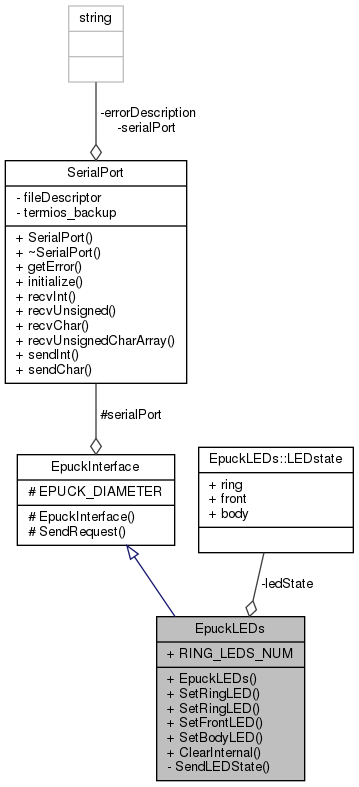
Detailed Description
This class controls the turn on and turn off of e-puck LEDs.
- Date
- December 2008
Classes | |
struct | LEDstate |
Struct with the state of e-puck LEDs. More... | |
Public Member Functions | |
EpuckLEDs (const SerialPort *const serialPort) | |
EpuckLEDs class constructor. More... | |
void | SetRingLED (bool ringLED[RING_LEDS_NUM]) |
Set the e-puck ring LEDs. More... | |
void | SetRingLED (unsigned id, bool state) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. More... | |
void | SetFrontLED (bool state) |
Set the e-puck front LED. More... | |
void | SetBodyLED (bool state) |
Set the e-puck body LED. More... | |
void | ClearInternal () |
Turn off the internal representation of all e-puck LEDs. More... | |
Static Public Attributes | |
static const unsigned | RING_LEDS_NUM = 8 |
The quantity of LEDs in e-puck ring LEDs. | |
Private Member Functions | |
void | SendLEDState () const |
Private Attributes | |
LEDstate | ledState |
Additional Inherited Members | |
![]() | |
enum | Request { CONFIG_CAMERA = 0x02, SET_VEL = 0x13, GET_STEPS = 0x14, STOP_MOTORS = 0x15, GET_IR_PROX = 0x16, GET_CAMERA_IMG = 0x17, SET_LED_POWER = 0x18 } |
Request codes acceptable by e-puck. More... | |
![]() | |
EpuckInterface (const SerialPort *const serialPort) | |
The EpuckInterface class constructor. More... | |
void | SendRequest (Request request) const |
![]() | |
const SerialPort *const | serialPort |
A SerialPort class instance shared among the device interfaces. More... | |
![]() | |
static const float | EPUCK_DIAMETER = 0.07 |
Diameter of e-puck body [m]. | |
Constructor & Destructor Documentation
◆ EpuckLEDs()
EpuckLEDs::EpuckLEDs | ( | const SerialPort *const | serialPort | ) |
EpuckLEDs class constructor.
- Parameters
-
serialPort Pointer for a SerialPort class already created and connected with an e-puck.
References EpuckLEDs::LEDstate::body, EpuckLEDs::LEDstate::front, SerialPort::recvChar(), EpuckLEDs::LEDstate::ring, RING_LEDS_NUM, SerialPort::sendChar(), EpuckInterface::serialPort, EpuckInterface::SET_LED_POWER, and SetRingLED().
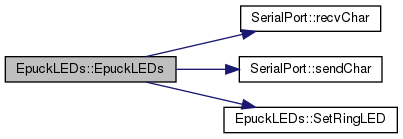
Member Function Documentation
◆ ClearInternal()
void EpuckLEDs::ClearInternal | ( | ) |
Turn off the internal representation of all e-puck LEDs.
This function turn off only the internal representation of the LEDs, in e-puck their state won't change. This is useful in unsubscribe operation, at end of program the LEDs won't chage. But in a new client program execution, only the desired LEDs will tuned on.
References EpuckLEDs::LEDstate::body, EpuckLEDs::LEDstate::front, EpuckLEDs::LEDstate::ring, and RING_LEDS_NUM.
◆ SetBodyLED()
void EpuckLEDs::SetBodyLED | ( | bool | state | ) |
Set the e-puck body LED.
- Parameters
-
state True for on, false for off.
References EpuckLEDs::LEDstate::body.
◆ SetFrontLED()
void EpuckLEDs::SetFrontLED | ( | bool | state | ) |
Set the e-puck front LED.
- Parameters
-
state True for on, false for off.
References EpuckLEDs::LEDstate::front.
◆ SetRingLED() [1/2]
void EpuckLEDs::SetRingLED | ( | bool | ringLED[RING_LEDS_NUM] | ) |
Set the e-puck ring LEDs.
The state of all ring LEDs is given in ringLED parameter.
- Parameters
-
ringLED The state of each ring LED, where true is LED on.
Referenced by EpuckLEDs().
◆ SetRingLED() [2/2]
void EpuckLEDs::SetRingLED | ( | unsigned | id, |
bool | state | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
The state of only one LED will be set, the others won't change.
- Parameters
-
id The index of the ring LED. state The desired state. True for on, false for off.
References EpuckLEDs::LEDstate::ring, and RING_LEDS_NUM.
The documentation for this class was generated from the following files:
- epuckLEDs.hpp
- epuckLEDs.cpp