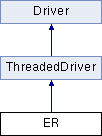
Public Member Functions | |
ER (ConfigFile *cf, int section) | |
int | SetVelocity (double lvel, double rvel) |
void | Stop (int StopMode) |
virtual void | Main () |
Main method for driver thread. | |
virtual int | MainSetup () |
Sets up the resources needed by the driver thread. | |
virtual void | MainQuit () |
Cleanup method for driver thread (called when main exits) | |
virtual int | ProcessMessage (QueuePointer &resp_queue, player_msghdr *hdr, void *data) |
Message handler. | |
void | Test () |
Private Member Functions | |
int | InitOdom () |
int | InitRobot () |
int | WriteBuf (unsigned char *s, size_t len) |
int | ReadBuf (unsigned char *s, size_t len) |
int | SendCommand (unsigned char *cmd, int cmd_len, unsigned char *ret_val, int ret_len) |
int | checksum_ok (unsigned char *buf, int len) |
int | ComputeTickDiff (int from, int to) |
int | ChangeMotorState (int state) |
int | BytesToInt32 (unsigned char *ptr) |
float | BytesToFloat (unsigned char *ptr) |
void | UpdateOdom (int ltics, int rtics) |
void | SpeedCommand (unsigned char address, double speed, int dir) |
void | SetOdometry (long, long, long) |
void | ResetOdometry () |
int | GetOdom (int *ltics, int *rtics) |
int | GetBatteryVoltage (int *voltage) |
int | GetRangeSensor (int s, float *val) |
void | MotorSpeed () |
int | send_command (unsigned char address, unsigned char c, int ret_num, unsigned char *ret) |
int | send_command_2_arg (unsigned char address, unsigned char c, int arg, int ret_num, unsigned char *ret) |
int | send_command_4_arg (unsigned char address, unsigned char c, int arg, int ret_num, unsigned char *ret) |
Private Attributes | |
player_er1_data_t | er1_data |
player_devaddr_t | position_id |
int | position_subscriptions |
int * | _tc_num |
int | _fd |
const char * | _serial_port |
bool | _fd_blocking |
double | _axle_length |
int | _motor_0_dir |
int | _motor_1_dir |
bool | _debug |
bool | _need_to_set_speed |
bool | _odom_initialized |
bool | _stopped |
int | _last_ltics |
int | _last_rtics |
double | _px |
double | _py |
double | _pa |
int | _powered |
int | _resting |
Member Function Documentation
void ER::Main | ( | ) | [virtual] |
Main method for driver thread.
drivers have their own thread of execution, created using StartThread(); this is the entry point for the driver thread, and must be overloaded by all threaded drivers.
Implements ThreadedDriver.
References player_pose2d::pa, PLAYER_MSGTYPE_DATA, PLAYER_POSITION2D_DATA_STATE, player_position2d_data::pos, Driver::ProcessMessages(), Driver::Publish(), player_pose2d::px, player_pose2d::py, player_position2d_data::stall, and player_position2d_data::vel.
void ER::MainQuit | ( | void | ) | [virtual] |
Cleanup method for driver thread (called when main exits)
Overload this method and to do additional cleanup when the driver thread exits.
Reimplemented from ThreadedDriver.
int ER::ProcessMessage | ( | QueuePointer & | resp_queue, |
player_msghdr * | hdr, | ||
void * | data | ||
) | [virtual] |
Message handler.
This function is called once for each message in the incoming queue. Reimplement it to provide message handling. Return 0 if you handled the message and -1 otherwise
- Parameters:
-
resp_queue The queue to which any response should go. hdr The message header data The message body
Reimplemented from Driver.
References player_position2d_cmd_car::angle, Message::MatchMessage(), player_pose2d::pa, PLAYER_MSGTYPE_CMD, PLAYER_MSGTYPE_REQ, PLAYER_MSGTYPE_RESP_ACK, PLAYER_MSGTYPE_RESP_NACK, PLAYER_POSITION2D_CMD_CAR, PLAYER_POSITION2D_CMD_VEL, PLAYER_POSITION2D_REQ_GET_GEOM, PLAYER_POSITION2D_REQ_MOTOR_POWER, PLAYER_POSITION2D_REQ_RESET_ODOM, PLAYER_POSITION2D_REQ_SET_ODOM, player_position2d_set_odom_req::pose, player_position2d_geom::pose, Driver::Publish(), player_pose2d::px, player_pose3d::px, player_pose2d::py, player_pose3d::py, player_pose3d::pyaw, player_position2d_geom::size, player_bbox3d::sl, player_position2d_power_config::state, player_msghdr::subtype, player_bbox3d::sw, player_position2d_cmd_vel::vel, and player_position2d_cmd_car::velocity.
The documentation for this class was generated from the following files:
- er.h
- er.cc