Class for to get images from e-puck camera. More...
#include <epuckCamera.hpp>
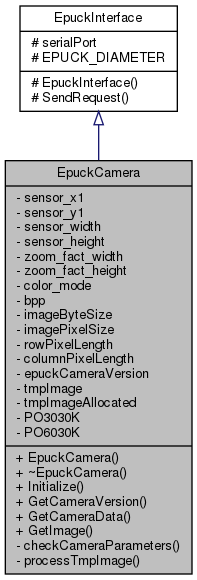
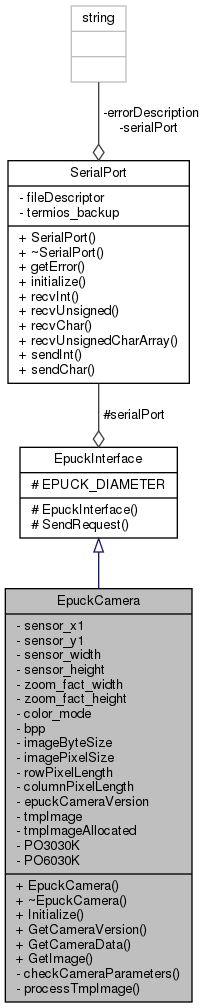
Detailed Description
Class for to get images from e-puck camera.
- Exceptions
-
camera_version_error Raised when the version of camera in e-puck is unknown. window_length_error Raised when the desired image window width or height is larger that e-puck camera can support. window_out_of_range Raised when the positioning of image window is out of image sensor border.
- Date
- October 2008
Classes | |
class | camera_version_error |
class | window_length_error |
class | window_out_of_range |
Public Types | |
enum | ColorModes { GREY_SCALE_MODE = 0, RGB_565_MODE = 1, YUV_MODE = 2 } |
Possible color modes for e-puck camera. More... | |
Public Member Functions | |
EpuckCamera (const SerialPort *const serialPort, unsigned sensor_x1, unsigned sensor_y1, unsigned sensor_width, unsigned sensor_height, unsigned zoom_fact_width, unsigned zoom_fact_height, ColorModes color_mode) throw () | |
The EpuckCamera class constructor. More... | |
void | Initialize () |
Send the configurations givens in EpuckCamera constructor to e-puck. More... | |
std::string | GetCameraVersion () const |
Get the version of camera in e-puck. More... | |
void | GetCameraData (unsigned &imageWidth, unsigned &imageHeight, EpuckCamera::ColorModes &colorMode) const |
Get the relevant configurations camera data. More... | |
void | GetImage (unsigned char *const ptrImage) |
Get a new image from e-puck. More... | |
Private Types | |
enum | { bpp_8, bpp_16 } |
Private Member Functions | |
void | checkCameraParameters () const |
template<typename T > | |
void | processTmpImage (T *ptrImage) const |
Private Attributes | |
unsigned | sensor_x1 |
unsigned | sensor_y1 |
unsigned | sensor_width |
unsigned | sensor_height |
unsigned | zoom_fact_width |
unsigned | zoom_fact_height |
ColorModes | color_mode |
enum EpuckCamera:: { ... } | bpp |
unsigned | imageByteSize |
unsigned | imagePixelSize |
unsigned | rowPixelLength |
unsigned | columnPixelLength |
unsigned | epuckCameraVersion |
unsigned char * | tmpImage |
bool | tmpImageAllocated |
Static Private Attributes | |
static const unsigned | PO3030K = 0x3030 |
static const unsigned | PO6030K = 0x6030 |
Additional Inherited Members | |
![]() | |
enum | Request { CONFIG_CAMERA = 0x02, SET_VEL = 0x13, GET_STEPS = 0x14, STOP_MOTORS = 0x15, GET_IR_PROX = 0x16, GET_CAMERA_IMG = 0x17, SET_LED_POWER = 0x18 } |
Request codes acceptable by e-puck. More... | |
![]() | |
EpuckInterface (const SerialPort *const serialPort) | |
The EpuckInterface class constructor. More... | |
void | SendRequest (Request request) const |
![]() | |
const SerialPort *const | serialPort |
A SerialPort class instance shared among the device interfaces. More... | |
![]() | |
static const float | EPUCK_DIAMETER = 0.07 |
Diameter of e-puck body [m]. | |
Member Enumeration Documentation
◆ ColorModes
Possible color modes for e-puck camera.
This are the possible color modes for e-puck camera. The YUV_MODE is unknown by Player, because of that, in this mode the variable "format" of playerc_camera_t struct, will be set to PLAYER_CAMERA_FORMAT_MONO16.
Enumerator | |
---|---|
GREY_SCALE_MODE | Grey color mode, with 8 bits per pixel. |
RGB_565_MODE | RGB color mode, with 16 bits per pixel. |
YUV_MODE | YUV color mode, with 16 bits per pixel. |
Constructor & Destructor Documentation
◆ EpuckCamera()
EpuckCamera::EpuckCamera | ( | const SerialPort *const | serialPort, |
unsigned | sensor_x1, | ||
unsigned | sensor_y1, | ||
unsigned | sensor_width, | ||
unsigned | sensor_height, | ||
unsigned | zoom_fact_width, | ||
unsigned | zoom_fact_height, | ||
ColorModes | color_mode | ||
) | |||
throw | ( | ||
) |
The EpuckCamera class constructor.
Except by serialPort, the other variables are mapped directly to variables with same name in e_po3030k_config_cam function, from official e-puck library.
- Parameters
-
serialPort Pointer for a SerialPort class already created and connected with an e-puck. sensor_x1 The X coordinate of the window's corner. sensor_y1 The Y coordinate of the window's corner. sensor_width The Width of the interest area, in FULL sampling scale. sensor_height The Height of the insterest area, in FULL sampling scale . zoom_fact_width The subsampling to apply for the window's width. zoom_fact_height The subsampling to apply for the window's height. color_mode The color mode in which the camera should be configured.
References GREY_SCALE_MODE, RGB_565_MODE, and YUV_MODE.
Member Function Documentation
◆ GetCameraData()
void EpuckCamera::GetCameraData | ( | unsigned & | imageWidth, |
unsigned & | imageHeight, | ||
EpuckCamera::ColorModes & | colorMode | ||
) | const |
Get the relevant configurations camera data.
- Parameters
-
imageWidth Image width in pixels. imageHeight Image height in pixels. colorMode Image color mode.
◆ GetCameraVersion()
std::string EpuckCamera::GetCameraVersion | ( | ) | const |
Get the version of camera in e-puck.
This method must be called after the Initialize method.
It can throw the camera_version_error exception.
- Returns
- A string representing the camera version.
◆ GetImage()
void EpuckCamera::GetImage | ( | unsigned char *const | ptrImage | ) |
Get a new image from e-puck.
- Parameters
-
ptrImage A pointer to a const char vector, where the incoming image will be put it.
References EpuckInterface::GET_CAMERA_IMG, SerialPort::recvUnsignedCharArray(), and EpuckInterface::serialPort.

◆ Initialize()
void EpuckCamera::Initialize | ( | ) |
Send the configurations givens in EpuckCamera constructor to e-puck.
This function must be called before an image be captured.
It can throw the following exceptions: std::length_error, camera_version_error, window_length_error and window_out_of_range.
References EpuckInterface::CONFIG_CAMERA, SerialPort::recvChar(), SerialPort::recvUnsigned(), SerialPort::sendInt(), and EpuckInterface::serialPort.
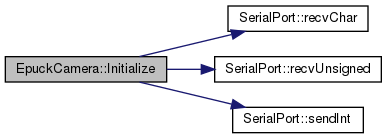
The documentation for this class was generated from the following files:
- epuckCamera.hpp
- epuckCamera.cpp