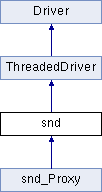
Public Member Functions | |
snd (ConfigFile *cf, int section) | |
virtual int | Setup () |
Initialize the driver. | |
virtual int | Shutdown () |
Finalize the driver. | |
virtual int | ProcessMessage (QueuePointer &resp_queue, player_msghdr *hdr, void *data) |
Message handler. | |
void | WaitForNextGoal () |
void | SignalNextGoal (double goalX, double goalY, double goalA) |
void | Read () |
void | ReadIfWaiting () |
Public Attributes | |
double | robot_radius |
double | min_gap_width |
double | obstacle_avoid_dist |
double | max_speed |
double | max_turn_rate |
double | goal_position_tol |
double | goal_angle_tol |
double | goalX |
double | goalY |
double | goalA |
pthread_t | algorithm_thread |
pthread_mutex_t | goal_mutex |
pthread_cond_t | goal_changed_cond |
double | goal_changed |
pthread_mutex_t | data_mutex |
pthread_cond_t | data_changed_cond |
double | data_changed |
int | data_odometry_ready |
int | data_laser_ready |
Protected Member Functions | |
void | SetSpeedCmd (player_position2d_cmd_vel_t cmd) |
Protected Attributes | |
player_pose2d_t | odom_pose |
std::vector< double > | laser__ranges |
double | laser__resolution |
double | laser__max_range |
uint32_t | laser__ranges_count |
Private Member Functions | |
virtual void | Main () |
Main method for driver thread. | |
int | Odometry_Setup () |
int | Laser_Setup () |
Private Attributes | |
player_devaddr_t | m_position_addr |
player_devaddr_t | m_laser_addr |
player_devaddr_t | laser_addr |
player_devaddr_t | odom_in_addr |
player_devaddr_t | odom_out_addr |
Device * | laser_dev |
Device * | odom_in_dev |
Device * | odom_out_dev |
player_position2d_geom_t | robot_geom |
int | first_goal_has_been_set_to_init_position |
Member Function Documentation
void snd::Main | ( | ) | [private, virtual] |
Main method for driver thread.
drivers have their own thread of execution, created using StartThread(); this is the entry point for the driver thread, and must be overloaded by all threaded drivers.
Implements ThreadedDriver.
References PLAYER_MSG0, Driver::ProcessMessages(), and ThreadedDriver::Wait().
int snd::ProcessMessage | ( | QueuePointer & | resp_queue, |
player_msghdr * | hdr, | ||
void * | data | ||
) | [virtual] |
Message handler.
This function is called once for each message in the incoming queue. Reimplement it to provide message handling. Return 0 if you handled the message and -1 otherwise
- Parameters:
-
resp_queue The queue to which any response should go. hdr The message header data The message body
Reimplemented from Driver.
References player_msghdr::addr, Driver::device_addr, Message::GetHeader(), Message::GetPayload(), Message::MatchMessage(), player_pose2d::pa, PLAYER_LASER_DATA_SCAN, PLAYER_MSG0, PLAYER_MSG3, PLAYER_MSGTYPE_CMD, PLAYER_MSGTYPE_DATA, PLAYER_MSGTYPE_REQ, PLAYER_POSITION2D_CMD_POS, PLAYER_POSITION2D_CMD_VEL, PLAYER_POSITION2D_DATA_STATE, PLAYER_WARN1, player_position2d_cmd_pos::pos, Driver::Publish(), player_pose2d::px, player_pose2d::py, Device::Request(), player_msghdr::size, player_msghdr::subtype, player_msghdr::timestamp, and player_msghdr::type.
int snd::Setup | ( | ) | [virtual] |
Initialize the driver.
This function is called with the first client subscribes; it MUST be implemented by the driver.
- Returns:
- Returns 0 on success.
Reimplemented from ThreadedDriver.
References PLAYER_MSG0.
int snd::Shutdown | ( | ) | [virtual] |
Finalize the driver.
This function is called with the last client unsubscribes; the default version simple stops the driver thread if one is present. In this case resources should be cleaned up in MainQuit.
- Returns:
- Returns 0 on success.
Reimplemented from ThreadedDriver.
References Driver::InQueue, PLAYER_MSG0, ThreadedDriver::StopThread(), and Device::Unsubscribe().
The documentation for this class was generated from the following files:
- snd.h
- snd.cc