canvas.hh
Go to the documentation of this file.00001 00002 #include "stage.hh" 00003 #include "option.hh" 00004 00005 #include <map> 00006 #include <stack> 00007 00008 namespace Stg 00009 { 00010 class Canvas : public Fl_Gl_Window 00011 { 00012 friend class WorldGui; // allow access to private members 00013 friend class Model; 00014 00015 private: 00016 00017 class GlColorStack 00018 { 00019 public: 00020 GlColorStack() : colorstack() {} 00021 ~GlColorStack() {} 00022 00023 void Push( double r, double g, double b, double a=1.0 ) 00024 { 00025 Push( Color(r,g,b,a) ); 00026 } 00027 00028 void Push( Color col ) 00029 { 00030 colorstack.push( col ); 00031 glColor4f( col.r, col.g, col.b, col.a ); 00032 } 00033 00034 void Pop() 00035 { 00036 if( colorstack.size() < 1 ) 00037 PRINT_WARN1( "Attempted to ColorStack.Pop() but ColorStack %p is empty", 00038 this ); 00039 else 00040 { 00041 Color& old = colorstack.top(); 00042 colorstack.pop(); 00043 glColor4f( old.r, old.g, old.b, old.a ); 00044 } 00045 } 00046 00047 unsigned int Length() 00048 { return colorstack.size(); } 00049 00050 private: 00051 std::stack<Color> colorstack; 00052 } colorstack; 00053 00054 std::list<Model*> models_sorted; 00055 00056 Camera* current_camera; 00057 OrthoCamera camera; 00058 PerspectiveCamera perspective_camera; 00059 bool dirty_buffer; 00060 Worldfile* wf; 00061 00062 int startx, starty; 00063 bool selectedModel; 00064 bool clicked_empty_space; 00065 int empty_space_startx, empty_space_starty; 00066 std::list<Model*> selected_models; 00067 Model* last_selection; 00068 00069 00070 stg_msec_t interval; // window refresh interval in ms 00071 00072 void RecordRay( double x1, double y1, double x2, double y2 ); 00073 void DrawRays(); 00074 void ClearRays(); 00075 void DrawGlobalGrid(); 00076 00077 void AddModel( Model* mod ); 00078 void RemoveModel( Model* mod ); 00079 00080 Option //showBlinken, 00081 showBBoxes, 00082 showBlocks, 00083 showBlur, 00084 showClock, 00085 showData, 00086 showFlags, 00087 showFollow, 00088 showFootprints, 00089 showGrid, 00090 showOccupancy, 00091 showScreenshots, 00092 showStatus, 00093 showTrailArrows, 00094 showTrailRise, 00095 showTrails, 00096 showVoxels, 00097 pCamOn, 00098 visualizeAll; 00099 00100 public: 00101 Canvas( WorldGui* world, int x, int y, int width, int height); 00102 ~Canvas(); 00103 00104 bool graphics; 00105 WorldGui* world; 00106 unsigned long frames_rendered_count; 00107 int screenshot_frame_skip; 00108 00109 std::map< std::string, Option* > _custom_options; 00110 00111 void Screenshot(); 00112 void InitGl(); 00113 void createMenuItems( Fl_Menu_Bar* menu, std::string path ); 00114 00115 void FixViewport(int W,int H); 00116 void DrawFloor(); //simpler floor compared to grid 00117 void DrawBlocks(); 00118 void DrawBoundingBoxes(); 00119 void resetCamera(); 00120 virtual void renderFrame(); 00121 virtual void draw(); 00122 virtual int handle( int event ); 00123 void resize(int X,int Y,int W,int H); 00124 00125 void CanvasToWorld( int px, int py, 00126 double *wx, double *wy, double* wz ); 00127 00128 Model* getModel( int x, int y ); 00129 bool selected( Model* mod ); 00130 void select( Model* mod ); 00131 void unSelect( Model* mod ); 00132 void unSelectAll(); 00133 00134 inline void setDirtyBuffer( void ) { dirty_buffer = true; } 00135 inline bool dirtyBuffer( void ) const { return dirty_buffer; } 00136 00137 void PushColor( Color col ) 00138 { colorstack.Push( col ); } 00139 00140 void PushColor( double r, double g, double b, double a ) 00141 { colorstack.Push( r,g,b,a ); } 00142 00143 void PopColor(){ colorstack.Pop(); } 00144 00145 void InvertView( uint32_t invertflags ); 00146 00147 bool VisualizeAll(){ return ! visualizeAll; } 00148 00149 static void TimerCallback( Canvas* canvas ); 00150 static void perspectiveCb( Fl_Widget* w, void* p ); 00151 00152 void EnterScreenCS(); 00153 void LeaveScreenCS(); 00154 00155 void Load( Worldfile* wf, int section ); 00156 void Save( Worldfile* wf, int section ); 00157 }; 00158 00159 } // namespace Stg
Generated on Tue Oct 20 15:42:05 2009 for Stage by
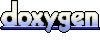