worldfile.hh
00001 /* 00002 * Stage : a multi-robot simulator. 00003 * Copyright (C) 2001, 2002 Richard Vaughan, Andrew Howard and Brian Gerkey. 00004 * 00005 * This program is free software; you can redistribute it and/or modify 00006 * it under the terms of the GNU General Public License as published by 00007 * the Free Software Foundation; either version 2 of the License, or 00008 * (at your option) any later version. 00009 * 00010 * This program is distributed in the hope that it will be useful, 00011 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00012 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00013 * GNU General Public License for more details. 00014 * 00015 * You should have received a copy of the GNU General Public License 00016 * along with this program; if not, write to the Free Software 00017 * Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA 00018 * 00019 */ 00020 /* 00021 * Desc: A class for reading in the world file. 00022 * Author: Andrew Howard 00023 * Date: 15 Nov 2001 00024 * CVS info: $Id: worldfile.hh 6825 2008-07-08 23:41:30Z jeremy_asher $ 00025 */ 00026 00027 #ifndef WORLDFILE_HH 00028 #define WORLDFILE_HH 00029 00030 00031 #include <stdint.h> // for portable int types eg. uint32_t 00032 #include <stdio.h> // for FILE ops 00033 #include <glib.h> 00034 00035 //#include "stage.hh" 00036 00037 namespace Stg { 00038 00039 // Private property class 00040 struct CProperty 00041 { 00042 // Index of entity this property belongs to 00043 int entity; 00044 00045 // Name of property 00046 const char *name; 00047 00048 char* key; // this property's hash table key 00049 00050 // A list of token indexes 00051 int value_count; 00052 int *values; 00053 00054 // Line this property came from 00055 int line; 00056 00057 // Flag set if property has been used 00058 bool used; 00059 }; 00060 00061 00062 // Class for loading/saving world file. This class hides the syntax 00063 // of the world file and provides an 'entity.property = value' style 00064 // interface. Global settings go in entity 0; every other entity 00065 // refers to a specific entity. Parent/child relationships are 00066 // encoded in the form of entity/subentity relationships. 00067 class Worldfile 00068 { 00069 // Standard constructors/destructors 00070 public: Worldfile(); 00071 public: ~Worldfile(); 00072 00073 // replacement for fopen() that checks STAGEPATH dirs for the named file 00074 // (thanks to Douglas S. Blank <dblank@brynmawr.edu>) 00075 protected: FILE* FileOpen(const char *filename, const char* method); 00076 00077 // Load world from file 00078 public: bool Load(const char *filename); 00079 00080 // Save world back into file 00081 // Set filename to NULL to save back into the original file 00082 public: bool Save(const char *filename); 00083 00084 // Check for unused properties and print warnings 00085 public: bool WarnUnused(); 00086 00087 // Read a string 00088 public: const char *ReadString(int entity, const char *name, const char *value); 00089 00090 // Write a string 00091 public: void WriteString(int entity, const char *name, const char *value); 00092 00093 // Read an integer 00094 public: int ReadInt(int entity, const char *name, int value); 00095 00096 // Write an integer 00097 public: void WriteInt(int entity, const char *name, int value); 00098 00099 // Read a float 00100 public: double ReadFloat(int entity, const char *name, double value); 00101 00102 // Write a float 00103 public: void WriteFloat(int entity, const char *name, double value); 00104 00105 // Read a length (includes unit conversion) 00106 public: double ReadLength(int entity, const char *name, double value); 00107 00108 // Write a length (includes units conversion) 00109 public: void WriteLength(int entity, const char *name, double value); 00110 00111 // Read an angle (includes unit conversion) 00112 public: double ReadAngle(int entity, const char *name, double value); 00113 00114 // Read a boolean 00115 // REMOVE? public: bool ReadBool(int entity, const char *name, bool value); 00116 00117 // Read a color (includes text to RGB conversion) 00118 public: uint32_t ReadColor(int entity, const char *name, uint32_t value); 00119 00120 // Read a file name. Always returns an absolute path. If the 00121 // filename is entered as a relative path, we prepend the world 00122 // files path to it. 00123 public: const char *ReadFilename(int entity, const char *name, const char *value); 00124 00125 // Read a string from a tuple 00126 public: const char *ReadTupleString(int entity, const char *name, 00127 int index, const char *value); 00128 00129 // Write a string to a tuple 00130 public: void WriteTupleString(int entity, const char *name, 00131 int index, const char *value); 00132 00133 // Read a float from a tuple 00134 public: double ReadTupleFloat(int entity, const char *name, 00135 int index, double value); 00136 00137 // Write a float to a tuple 00138 public: void WriteTupleFloat(int entity, const char *name, 00139 int index, double value); 00140 00141 // Read a length from a tuple (includes units conversion) 00142 public: double ReadTupleLength(int entity, const char *name, 00143 int index, double value); 00144 00145 // Write a to a tuple length (includes units conversion) 00146 public: void WriteTupleLength(int entity, const char *name, 00147 int index, double value); 00148 00149 // Read an angle form a tuple (includes units conversion) 00150 public: double ReadTupleAngle(int entity, const char *name, 00151 int index, double value); 00152 00153 // Write an angle to a tuple (includes units conversion) 00154 public: void WriteTupleAngle(int entity, const char *name, 00155 int index, double value); 00156 00157 00159 // Private methods used to load stuff from the world file 00160 00161 // Load tokens from a file. 00162 private: bool LoadTokens(FILE *file, int include); 00163 00164 // Read in a comment token 00165 private: bool LoadTokenComment(FILE *file, int *line, int include); 00166 00167 // Read in a word token 00168 private: bool LoadTokenWord(FILE *file, int *line, int include); 00169 00170 // Load an include token; this will load the include file. 00171 private: bool LoadTokenInclude(FILE *file, int *line, int include); 00172 00173 // Read in a number token 00174 private: bool LoadTokenNum(FILE *file, int *line, int include); 00175 00176 // Read in a string token 00177 private: bool LoadTokenString(FILE *file, int *line, int include); 00178 00179 // Read in a whitespace token 00180 private: bool LoadTokenSpace(FILE *file, int *line, int include); 00181 00182 // Save tokens to a file. 00183 private: bool SaveTokens(FILE *file); 00184 00185 // Clear the token list 00186 private: void ClearTokens(); 00187 00188 // Add a token to the token list 00189 private: bool AddToken(int type, const char *value, int include); 00190 00191 // Set a token in the token list 00192 private: bool SetTokenValue(int index, const char *value); 00193 00194 // Get the value of a token 00195 private: const char *GetTokenValue(int index); 00196 00197 // Dump the token list (for debugging). 00198 private: void DumpTokens(); 00199 00200 // Parse a line 00201 private: bool ParseTokens(); 00202 00203 // Parse an include statement 00204 private: bool ParseTokenInclude(int *index, int *line); 00205 00206 // Parse a macro definition 00207 private: bool ParseTokenDefine(int *index, int *line); 00208 00209 // Parse an word (could be a entity or an property) from the token list. 00210 private: bool ParseTokenWord(int entity, int *index, int *line); 00211 00212 // Parse a entity from the token list. 00213 private: bool ParseTokenEntity(int entity, int *index, int *line); 00214 00215 // Parse an property from the token list. 00216 private: bool ParseTokenProperty(int entity, int *index, int *line); 00217 00218 // Parse a tuple. 00219 private: bool ParseTokenTuple( CProperty* property, int *index, int *line); 00220 00221 // Clear the macro list 00222 private: void ClearMacros(); 00223 00224 // Add a macro 00225 private: int AddMacro(const char *macroname, const char *entityname, 00226 int line, int starttoken, int endtoken); 00227 00228 // Lookup a macro by name 00229 // Returns -1 if there is no macro with this name. 00230 private: int LookupMacro(const char *macroname); 00231 00232 // Dump the macro list for debugging 00233 private: void DumpMacros(); 00234 00235 // Clear the entity list 00236 private: void ClearEntities(); 00237 00238 // Add a entity 00239 private: int AddEntity(int parent, const char *type); 00240 00241 // Get the number of entities. 00242 public: int GetEntityCount(); 00243 00244 // Get a entity (returns the entity type value) 00245 public: const char *GetEntityType(int entity); 00246 00247 // Lookup a entity number by type name 00248 // Returns -1 if there is entity with this type 00249 public: int LookupEntity(const char *type); 00250 00251 // Get a entity's parent entity. 00252 // Returns -1 if there is no parent. 00253 public: int GetEntityParent(int entity); 00254 00255 // Dump the entity list for debugging 00256 private: void DumpEntities(); 00257 00258 // Clear the property list 00259 private: void ClearProperties(); 00260 00261 // Add an property 00262 private: CProperty* AddProperty(int entity, const char *name, int line); 00263 // Add an property value. 00264 private: void AddPropertyValue( CProperty* property, int index, int value_token); 00265 00266 // Get an property 00267 public: CProperty* GetProperty(int entity, const char *name); 00268 00269 // returns true iff the property exists in the file, so that you can 00270 // be sure that GetProperty() will work 00271 bool PropertyExists( int section, const char* token ); 00272 00273 // Set the value of an property. 00274 private: void SetPropertyValue( CProperty* property, int index, const char *value); 00275 00276 // Get the value of an property. 00277 private: const char *GetPropertyValue( CProperty* property, int index); 00278 00279 // Dump the property list for debugging 00280 private: void DumpProperties(); 00281 00282 // Token types. 00283 private: enum 00284 { 00285 TokenComment, 00286 TokenWord, TokenNum, TokenString, 00287 TokenOpenEntity, TokenCloseEntity, 00288 TokenOpenTuple, TokenCloseTuple, 00289 TokenSpace, TokenEOL 00290 }; 00291 00292 // Token structure. 00293 private: struct CToken 00294 { 00295 // Non-zero if token is from an include file. 00296 int include; 00297 00298 // Token type (enumerated value). 00299 int type; 00300 00301 // Token value 00302 char *value; 00303 }; 00304 00305 // A list of tokens loaded from the file. 00306 // Modified values are written back into the token list. 00307 private: int token_size, token_count; 00308 private: CToken *tokens; 00309 00310 // Private macro class 00311 private: struct CMacro 00312 { 00313 // Name of macro 00314 const char *macroname; 00315 00316 // Name of entity 00317 const char *entityname; 00318 00319 // Line the macro definition starts on. 00320 int line; 00321 00322 // Range of tokens in the body of the macro definition. 00323 int starttoken, endtoken; 00324 }; 00325 00326 // Macro list 00327 private: int macro_size; 00328 private: int macro_count; 00329 private: CMacro *macros; 00330 00331 // Private entity class 00332 private: struct CEntity 00333 { 00334 // Parent entity 00335 int parent; 00336 00337 // Type of entity (i.e. position, laser, etc). 00338 const char *type; 00339 }; 00340 00341 // Entity list 00342 private: int entity_size; 00343 private: int entity_count; 00344 private: CEntity *entities; 00345 00346 00347 // Property list 00348 //private: int property_size; 00349 private: int property_count; 00350 //private: CProperty *properties; 00351 00352 private: GHashTable* nametable; 00353 00354 // Name of the file we loaded 00355 public: char *filename; 00356 00357 // Conversion units 00358 private: double unit_length; 00359 private: double unit_angle; 00360 }; 00361 00362 }; 00363 00364 #endif
Generated on Wed Jul 30 11:36:06 2008 for Stage by
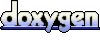