region.hh
00001 /* 00002 region.hh 00003 data structures supporting multi-resolution ray tracing in world class. 00004 Copyright Richard Vaughan 2008 00005 */ 00006 00007 const uint32_t RBITS = 6; // regions contain (2^RBITS)^2 pixels 00008 const uint32_t SBITS = 5; // superregions contain (2^SBITS)^2 regions 00009 const uint32_t SRBITS = RBITS+SBITS; 00010 00011 typedef struct 00012 { 00013 GSList* list; 00014 } stg_cell_t; 00015 00016 class Stg::Region 00017 { 00018 friend class SuperRegion; 00019 00020 private: 00021 static const uint32_t REGIONWIDTH = 1<<RBITS; 00022 static const uint32_t REGIONSIZE = REGIONWIDTH*REGIONWIDTH; 00023 00024 stg_cell_t cells[REGIONSIZE]; 00025 00026 public: 00027 uint32_t count; // number of blocks rendered into these cells 00028 00029 Region() 00030 { 00031 bzero( cells, REGIONSIZE * sizeof(stg_cell_t)); 00032 count = 0; 00033 } 00034 00035 stg_cell_t* GetCell( int32_t x, int32_t y ) 00036 { 00037 uint32_t index = x + (y*REGIONWIDTH); 00038 #ifdef DEBUG 00039 assert( index >=0 ); 00040 assert( index < REGIONSIZE ); 00041 #endif 00042 return &cells[index]; 00043 } 00044 00045 // add a block to a region cell specified in REGION coordinates 00046 void AddBlock( StgBlock* block, int32_t x, int32_t y, unsigned int* count2 ) 00047 { 00048 stg_cell_t* cell = GetCell( x, y ); 00049 cell->list = g_slist_prepend( cell->list, block ); 00050 block->RecordRenderPoint( &cell->list, cell->list, &this->count, count2 ); 00051 count++; 00052 } 00053 }; 00054 00055 00056 class Stg::SuperRegion 00057 { 00058 friend class StgWorld; 00059 00060 private: 00061 static const uint32_t SUPERREGIONWIDTH = 1<<SBITS; 00062 static const uint32_t SUPERREGIONSIZE = SUPERREGIONWIDTH*SUPERREGIONWIDTH; 00063 00064 Region regions[SUPERREGIONSIZE]; 00065 uint32_t count; // number of blocks rendered into these regions 00066 00067 stg_point_int_t origin; 00068 00069 public: 00070 00071 SuperRegion( int32_t x, int32_t y ) 00072 { 00073 count = 0; 00074 origin.x = x; 00075 origin.y = y; 00076 } 00077 00078 ~SuperRegion() 00079 { 00080 } 00081 00082 // get the region x,y from the region array 00083 Region* GetRegion( int32_t x, int32_t y ) 00084 { 00085 int32_t index = x + (y*SUPERREGIONWIDTH); 00086 assert( index >=0 ); 00087 assert( index < (int)SUPERREGIONSIZE ); 00088 return ®ions[ index ]; 00089 } 00090 00091 // add a block to a cell specified in superregion coordinates 00092 void AddBlock( StgBlock* block, int32_t x, int32_t y ) 00093 { 00094 GetRegion( x>>RBITS, y>>RBITS ) 00095 ->AddBlock( block, 00096 x - ((x>>RBITS)<<RBITS), 00097 y - ((y>>RBITS)<<RBITS), 00098 &count); 00099 count++; 00100 } 00101 00102 // callback wrapper for Draw() 00103 static void Draw_cb( gpointer dummykey, 00104 SuperRegion* sr, 00105 gpointer dummyval ) 00106 { 00107 sr->Draw(); 00108 } 00109 00110 void Draw() 00111 { 00112 glPushMatrix(); 00113 glTranslatef( origin.x<<SRBITS, origin.y<<SRBITS,0); 00114 00115 glColor3f( 1,0,0 ); 00116 00117 for( unsigned int x=0; x<SUPERREGIONWIDTH; x++ ) 00118 for( unsigned int y=0; y<SUPERREGIONWIDTH; y++ ) 00119 { 00120 Region* r = GetRegion(x,y); 00121 00122 for( unsigned int p=0; p<Region::REGIONWIDTH; p++ ) 00123 for( unsigned int q=0; q<Region::REGIONWIDTH; q++ ) 00124 if( r->cells[p+(q*Region::REGIONWIDTH)].list ) 00125 glRecti( p+(x<<RBITS), q+(y<<RBITS), 00126 1+p+(x<<RBITS), 1+q+(y<<RBITS) ); 00127 } 00128 00129 // outline regions 00130 glColor3f( 0,1,0 ); 00131 for( unsigned int x=0; x<SUPERREGIONWIDTH; x++ ) 00132 for( unsigned int y=0; y<SUPERREGIONWIDTH; y++ ) 00133 glRecti( x<<RBITS, y<<RBITS, 00134 (x+1)<<RBITS, (y+1)<<RBITS ); 00135 00136 // outline superregion 00137 glColor3f( 0,0,1 ); 00138 glRecti( 0,0, 1<<SRBITS, 1<<SRBITS ); 00139 00140 glPopMatrix(); 00141 } 00142 00143 static void Floor_cb( gpointer dummykey, 00144 SuperRegion* sr, 00145 gpointer dummyval ) 00146 { 00147 sr->Floor(); 00148 } 00149 00150 void Floor() 00151 { 00152 glPushMatrix(); 00153 glTranslatef( origin.x<<SRBITS, origin.y<<SRBITS, 0 ); 00154 glRecti( 0,0, 1<<SRBITS, 1<<SRBITS ); 00155 glPopMatrix(); 00156 } 00157 };
Generated on Wed Jul 30 11:36:06 2008 for Stage by
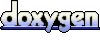