option.hh
00001 #ifndef _OPTION_H_ 00002 #define _OPTION_H_ 00003 00004 #include <string> 00005 #include "worldfile.hh" 00006 00007 #include <FL/Fl_Menu_Bar.H> 00008 #include <FL/Fl_Menu_Item.H> 00009 00010 namespace Stg { 00018 class Option { 00019 private: 00020 friend bool compare( const Option* lhs, const Option* rhs ); 00021 00022 std::string optName; 00023 bool value; 00025 std::string wf_token; 00026 std::string shortcut; 00027 Fl_Menu_* menu; 00028 int menuIndex; 00029 Fl_Callback* menuCb; 00030 Fl_Widget* menuCbWidget; 00031 00032 public: 00033 Option( std::string n, std::string tok, std::string key, bool v ); 00034 00035 const std::string name() const { return optName; } 00036 inline bool val() const { return value; } 00037 inline operator bool() { return val(); } 00038 inline bool operator<( const Option& rhs ) const 00039 { return optName<rhs.optName; } 00040 void set( bool val ); 00041 void invert() { set( !value ); } 00042 00043 // Comparator to dereference Option pointers and compare their strings 00044 struct optComp { 00045 inline bool operator()( const Option* lhs, const Option* rhs ) const 00046 { return lhs->operator<(*rhs); } 00047 }; 00048 00049 00050 void createMenuItem( Fl_Menu_Bar* menu, std::string path ); 00051 void menuCallback( Fl_Callback* cb, Fl_Widget* w ); 00052 static void toggleCb( Fl_Widget* w, void* p ); 00053 void Load( Worldfile* wf, int section ); 00054 void Save( Worldfile* wf, int section ); 00055 }; 00056 } 00057 00058 #endif
Generated on Wed Jul 30 11:36:06 2008 for Stage by
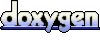