worldfile.hh
Go to the documentation of this file.00001 /* 00002 * Stage : a multi-robot simulator. 00003 * Copyright (C) 2001, 2002 Richard Vaughan, Andrew Howard and Brian Gerkey. 00004 * 00005 * This program is free software; you can redistribute it and/or modify 00006 * it under the terms of the GNU General Public License as published by 00007 * the Free Software Foundation; either version 2 of the License, or 00008 * (at your option) any later version. 00009 * 00010 * This program is distributed in the hope that it will be useful, 00011 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00012 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00013 * GNU General Public License for more details. 00014 * 00015 * You should have received a copy of the GNU General Public License 00016 * along with this program; if not, write to the Free Software 00017 * Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA 00018 * 00019 */ 00020 /* 00021 * Desc: A class for reading in the world file. 00022 * Author: Andrew Howard, Richard Vaughan 00023 * Date: 15 Nov 2001 00024 * CVS info: $Id: worldfile.hh 8264 2009-09-26 01:28:12Z rtv $ 00025 */ 00026 00027 #ifndef WORLDFILE_HH 00028 #define WORLDFILE_HH 00029 00030 00031 #include <stdint.h> // for portable int types eg. uint32_t 00032 #include <stdio.h> // for FILE ops 00033 00034 namespace Stg { 00035 00037 class CProperty 00038 { 00039 public: 00041 int entity; 00042 00044 std::string name; 00045 00047 std::vector<int> values; 00048 00050 int line; 00051 00053 bool used; 00054 00055 CProperty( int entity, const char* name, int line ) : 00056 entity(entity), 00057 name(name), 00058 values(), 00059 line(line), 00060 used(false) {} 00061 }; 00062 00063 00064 // Class for loading/saving world file. This class hides the syntax 00065 // of the world file and provides an 'entity.property = value' style 00066 // interface. Global settings go in entity 0; every other entity 00067 // refers to a specific entity. Parent/child relationships are 00068 // encoded in the form of entity/subentity relationships. 00069 class Worldfile 00070 { 00071 // Standard constructors/destructors 00072 public: Worldfile(); 00073 public: ~Worldfile(); 00074 00075 // replacement for fopen() that checks STAGEPATH dirs for the named file 00076 // (thanks to Douglas S. Blank <dblank@brynmawr.edu>) 00077 protected: FILE* FileOpen(const char *filename, const char* method); 00078 00079 // Load world from file 00080 public: bool Load(const char *filename); 00081 00082 // Save world back into file 00083 // Set filename to NULL to save back into the original file 00084 public: bool Save(const char *filename); 00085 00086 // Check for unused properties and print warnings 00087 public: bool WarnUnused(); 00088 00089 // Read a string 00090 public: const std::string ReadString(int entity, const char* name, const std::string& value); 00091 00092 // Write a string 00093 public: void WriteString(int entity, const char *name, const std::string& value ); 00094 00095 // Read an integer 00096 public: int ReadInt(int entity, const char *name, int value); 00097 00098 // Write an integer 00099 public: void WriteInt(int entity, const char *name, int value); 00100 00101 // Read a float 00102 public: double ReadFloat(int entity, const char *name, double value); 00103 00104 // Write a float 00105 public: void WriteFloat(int entity, const char *name, double value); 00106 00107 // Read a length (includes unit conversion) 00108 public: double ReadLength(int entity, const char *name, double value); 00109 00110 // Write a length (includes units conversion) 00111 public: void WriteLength(int entity, const char *name, double value); 00112 00113 // Read an angle (includes unit conversion) 00114 public: double ReadAngle(int entity, const char *name, double value); 00115 00116 // Read a boolean 00117 // REMOVE? public: bool ReadBool(int entity, const char *name, bool value); 00118 00119 // Read a color (includes text to RGB conversion) 00120 public: uint32_t ReadColor(int entity, const char *name, uint32_t value); 00121 00122 // Read a file name. Always returns an absolute path. If the 00123 // filename is entered as a relative path, we prepend the world 00124 // files path to it. 00125 public: const char *ReadFilename(int entity, const char *name, const char *value); 00126 00127 // Read a string from a tuple 00128 public: const char *ReadTupleString(int entity, const char *name, 00129 int index, const char *value); 00130 00131 // Write a string to a tuple 00132 public: void WriteTupleString(int entity, const char *name, 00133 int index, const char *value); 00134 00135 // Read a float from a tuple 00136 public: double ReadTupleFloat(int entity, const char *name, 00137 int index, double value); 00138 00139 // Write a float to a tuple 00140 public: void WriteTupleFloat(int entity, const char *name, 00141 int index, double value); 00142 00143 // Read a length from a tuple (includes units conversion) 00144 public: double ReadTupleLength(int entity, const char *name, 00145 int index, double value); 00146 00147 // Write a to a tuple length (includes units conversion) 00148 public: void WriteTupleLength(int entity, const char *name, 00149 int index, double value); 00150 00151 // Read an angle form a tuple (includes units conversion) 00152 public: double ReadTupleAngle(int entity, const char *name, 00153 int index, double value); 00154 00155 // Write an angle to a tuple (includes units conversion) 00156 public: void WriteTupleAngle(int entity, const char *name, 00157 int index, double value); 00158 00159 00161 // Private methods used to load stuff from the world file 00162 00163 // Load tokens from a file. 00164 private: bool LoadTokens(FILE *file, int include); 00165 00166 // Read in a comment token 00167 private: bool LoadTokenComment(FILE *file, int *line, int include); 00168 00169 // Read in a word token 00170 private: bool LoadTokenWord(FILE *file, int *line, int include); 00171 00172 // Load an include token; this will load the include file. 00173 private: bool LoadTokenInclude(FILE *file, int *line, int include); 00174 00175 // Read in a number token 00176 private: bool LoadTokenNum(FILE *file, int *line, int include); 00177 00178 // Read in a string token 00179 private: bool LoadTokenString(FILE *file, int *line, int include); 00180 00181 // Read in a whitespace token 00182 private: bool LoadTokenSpace(FILE *file, int *line, int include); 00183 00184 // Save tokens to a file. 00185 private: bool SaveTokens(FILE *file); 00186 00187 // Clear the token list 00188 private: void ClearTokens(); 00189 00190 // Add a token to the token list 00191 private: bool AddToken(int type, const char *value, int include); 00192 00193 // Set a token in the token list 00194 private: bool SetTokenValue(int index, const char *value); 00195 00196 // Get the value of a token 00197 private: const char *GetTokenValue(int index); 00198 00199 // Dump the token list (for debugging). 00200 private: void DumpTokens(); 00201 00202 // Parse a line 00203 private: bool ParseTokens(); 00204 00205 // Parse an include statement 00206 private: bool ParseTokenInclude(int *index, int *line); 00207 00208 // Parse a macro definition 00209 private: bool ParseTokenDefine(int *index, int *line); 00210 00211 // Parse an word (could be a entity or an property) from the token list. 00212 private: bool ParseTokenWord(int entity, int *index, int *line); 00213 00214 // Parse a entity from the token list. 00215 private: bool ParseTokenEntity(int entity, int *index, int *line); 00216 00217 // Parse an property from the token list. 00218 private: bool ParseTokenProperty(int entity, int *index, int *line); 00219 00220 // Parse a tuple. 00221 private: bool ParseTokenTuple( CProperty* property, int *index, int *line); 00222 00223 // Clear the macro list 00224 private: void ClearMacros(); 00225 00226 // Add a macro 00227 private: void AddMacro(const char *macroname, const char *entityname, 00228 int line, int starttoken, int endtoken); 00229 00230 // Lookup a macro by name 00231 00232 // Returns a pointer to a macro with this name, or NULL if there is none.. 00233 class CMacro; 00234 private: CMacro* LookupMacro(const char *macroname); 00235 00236 // Dump the macro list for debugging 00237 private: void DumpMacros(); 00238 00239 // Clear the entity list 00240 private: void ClearEntities(); 00241 00242 // Add a entity 00243 private: int AddEntity(int parent, const char *type); 00244 00245 // Get the number of entities. 00246 public: int GetEntityCount(); 00247 00248 // Get a entity (returns the entity type value) 00249 public: const char *GetEntityType(int entity); 00250 00251 // Lookup a entity number by type name 00252 // Returns -1 if there is entity with this type 00253 public: int LookupEntity(const char *type); 00254 00255 // Get a entity's parent entity. 00256 // Returns -1 if there is no parent. 00257 public: int GetEntityParent(int entity); 00258 00259 // Dump the entity list for debugging 00260 private: void DumpEntities(); 00261 00262 // Clear the property list 00263 private: void ClearProperties(); 00264 00265 // Add an property 00266 private: CProperty* AddProperty(int entity, const char *name, int line); 00267 // Add an property value. 00268 private: void AddPropertyValue( CProperty* property, int index, int value_token); 00269 00270 // Get an property 00271 public: CProperty* GetProperty(int entity, const char *name); 00272 00273 // returns true iff the property exists in the file, so that you can 00274 // be sure that GetProperty() will work 00275 bool PropertyExists( int section, const char* token ); 00276 00277 // Set the value of an property. 00278 private: void SetPropertyValue( CProperty* property, int index, const char *value); 00279 00280 // Get the value of an property. 00281 public: const char *GetPropertyValue( CProperty* property, int index); 00282 00283 // Dump the property list for debugging 00284 private: void DumpProperties(); 00285 00286 // Token types. 00287 private: enum 00288 { 00289 TokenComment, 00290 TokenWord, TokenNum, TokenString, 00291 TokenOpenEntity, TokenCloseEntity, 00292 TokenOpenTuple, TokenCloseTuple, 00293 TokenSpace, TokenEOL 00294 }; 00295 00296 // Token structure. 00297 private: 00298 class CToken 00299 { 00300 public: 00301 // Non-zero if token is from an include file. 00302 int include; 00303 00304 // Token type (enumerated value). 00305 int type; 00306 00307 // Token value 00308 std::string value; 00309 00310 CToken( int include, int type, const char* value ) : 00311 include(include), type(type), value(value) {} 00312 }; 00313 00314 // A list of tokens loaded from the file. 00315 // Modified values are written back into the token list. 00316 //private: int token_size, token_count; 00317 private: std::vector<CToken> tokens; 00318 00319 // Private macro class 00320 private: 00321 class CMacro 00322 { 00323 public: 00324 // Name of macro 00325 std::string macroname; 00326 00327 // Name of entity 00328 std::string entityname; 00329 00330 // Line the macro definition starts on. 00331 int line; 00332 00333 // Range of tokens in the body of the macro definition. 00334 int starttoken, endtoken; 00335 00336 CMacro(const char *macroname, const char *entityname, 00337 int line, int starttoken, int endtoken) : 00338 macroname(macroname), 00339 entityname(entityname), 00340 line(line), 00341 starttoken(starttoken), 00342 endtoken(endtoken) {} 00343 }; 00344 00345 // Macro table 00346 private: std::map<std::string,CMacro> macros; 00347 00348 // Private entity class 00349 private: 00350 class CEntity 00351 { 00352 public: 00353 // Parent entity 00354 int parent; 00355 00356 // Type of entity (i.e. position, laser, etc). 00357 std::string type; 00358 00359 CEntity( int parent, const char* type ) : parent(parent), type(type) {} 00360 }; 00361 00362 // Entity list 00363 private: std::vector<CEntity> entities; 00364 00365 // Property list 00366 private: std::map<std::string,CProperty*> properties; 00367 00368 // Name of the file we loaded 00369 public: std::string filename; 00370 00371 // Conversion units 00372 public: double unit_length; 00373 public: double unit_angle; 00374 00375 }; 00376 00377 }; 00378 00379 #endif
Generated on Tue Oct 20 15:42:05 2009 for Stage by
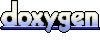