zoo_controller.h
Go to the documentation of this file.00001 #ifndef _STAGE_ZOO_CONTROLLER_H 00002 #define _STAGE_ZOO_CONTROLLER_H 00003 00004 #include "p_driver.h" 00005 #include <limits.h> 00006 #include <vector> 00007 #include <map> 00008 #include <sys/types.h> 00009 #include <stdio.h> 00010 #include "zoo.h" 00011 00012 /* some forward declarations here */ 00013 class ZooSpecies; 00014 class ZooController; 00015 class ZooDriver; 00016 class ZooReferee; 00017 00018 class ZooController 00019 { 00020 public: 00021 ZooController(ConfigFile *cf, int section, ZooDriver *zd, ZooSpecies *sp); 00022 ~ZooController(); 00023 00024 void Run(int port); 00025 void Kill(void); 00026 inline int GetFrequency(void) { return frequency; } 00027 inline const char *GetCommand(void) { return command; } 00028 00029 #if 0 00030 /* A controller can be a member of more than one species, and each 00031 * species may have its own way of keeping score, so each controller 00032 * should maintain a map from species names to scores */ 00033 std::map< const char *, void * > scoreMap; 00034 std::map< const char *, size_t > scoreSizeMap; 00035 #endif 00036 void *score_data; 00037 int score_size; 00038 00039 static const char *path; 00040 ZooSpecies *species; 00041 ZooDriver *zoo; 00042 private: 00043 pid_t pid; 00044 int frequency; 00045 const char *command; 00046 const char *outfilename, *outfilemode; 00047 const char *errfilename, *errfilemode; 00048 void cpathprintf(char *, const char *fmt, const rmap_t *); 00049 }; 00050 00051 #endif
Generated on Thu Dec 13 14:35:18 2007 for Stage by
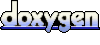