ZooDriver
[Zoo]
Function Documentation
|
Erase the score data for this model. Frees any allocated memory.
|
|
Find a robot in the robot map, searching by model. |
|
Find a robot in the robot map, searching by Controller. |
|
Find a robot in the robot map, searching by port number. |
|
Find a robot in the robot map, searching by model name. |
|
Get a Stage stg_model_t object for the kth model in the robot map.
|
|
Get a Stage stg_model_t object for the model with this name (the stg_model_t.token entry).
|
|
Get a Stage stg_model_t object for the model on the given port.
|
|
Get the number of top-level models. FIXME: This should be the number of top-level models that Zoo cares about; i.e., the ones in the population of some species.
|
|
DEPRECATED: Use ZooDriver::FindRobot instead.
|
|
DEPRECATED: Use ZooDriver::FindRobot instead.
|
|
DEPRECATED: Use ZooDriver::FindRobot instead.
|
|
Get the score data for this model. If no score data has been set yet (using ZooDriver::SetScore), return 0. The caller must provide enough space to store the data, which is in a format unknown to Zoo.
|
|
Get the size of the score data for this model. 0 could mean that no score data has been set yet.
|
|
Locate a species by its name.
|
|
Kill the controller controlling a robot with a given top-level model name.
|
|
Kill the controller for a robot on a given port.
|
|
Kill all active controllers. |
|
Player calls this after everything else is ready; Zoo uses it to tell the referee to start. |
|
Run a controller to control a robot with a given model name. NOTE: currently uses GetModelPortByName, which I want to deprecate in favour of FindRobot.
|
|
Run a controller on a specific port. If more than one species has a robot on this port in its population, then more than one controller will be started.
|
|
Run a controller for every member of the populations of every species. |
|
Set the score data for this model. Reallocates storage, stored locally, each time the function is called.
|
|
Set the callback for drawing score data on the GUI, but do so for every species. |
|
Player wants Setup and Shutdown functions; NOOP for now. |
|
Player wants Setup and Shutdown functions; NOOP for now. |
|
ZooDriver constructor. Load all the species. TODO: The Player config-file reader should really have a way of getting all child sections of a given section. Then the species and controller sections could be included directly in the zoo section, and this would be syntactically meaningful. |
|
Called by player_driver_init, defined in p_driver.cc. Just creates a new ZooDriver object.
|
|
Register the name of this driver, along with the function that creates it, with Player's driver table.;
|
|
ZooDriver destructor. Deletes the species list and robot map entries. |
Variable Documentation
|
Description: An addon to the Stage plugin for Zoo functionality. Author: Adam Lein Date: July 14, 2005 |
Generated on Thu Dec 13 14:35:18 2007 for Stage by
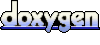